


The Go technology stack provides a variety of tools, frameworks and libraries to facilitate development: Tools: including Go compiler, code formatting tools, etc., for writing, debugging and optimizing code. Frameworks: including Echo, Gin, Beego, etc., which can be used to quickly build web servers and APIs. Library: Contains gorm, xorm, go-redis, etc., for interacting with databases, processing Redis data, and more.
Golang technology stack detailed explanation: tools, frameworks and libraries
Golang is a dynamically compiled language with rapid development, high Features such as performance and concise syntax. This article will introduce the commonly used tools, frameworks and libraries in the Golang technology stack, and provide practical practical cases.
Tools
- Go: Go language compiler and runtime environment.
- Go fmt: Code formatting tool.
- Go vet: Code inspection tool.
Practical case:
package main func main() { fmt.Println("Hello, world!") }
Run the following command to format the code:
go fmt main.go
Framework
- Echo: A framework for creating high-performance web servers and APIs.
- Gin: A lightweight web framework focused on performance and ease of use.
- Beego: Full-stack web development framework, providing ORM, template engine and routing.
Practical case:
package main import ( "github.com/labstack/echo" ) func main() { e := echo.New() e.GET("/", func(c echo.Context) error { return c.String(200, "Hello, world!") }) e.Logger.Fatal(e.Start(":8080")) }
Run the following command to start the server:
go run main.go
Library
- gorm: Object-relational mapping (ORM) library for interacting with databases.
- xorm: Another ORM library that supports multiple databases.
- go-redis: Redis client library.
Practical case:
package main import ( "github.com/jinzhu/gorm" _ "github.com/jinzhu/gorm/dialects/mysql" ) type User struct { ID uint `gorm:"primary_key"` Name string } func main() { db, err := gorm.Open("mysql", "username:password@tcp(127.0.0.1:3306)/dbname?charset=utf8&parseTime=True&loc=Local") if err != nil { panic(err) } defer db.Close() db.AutoMigrate(&User{}) }
The above code creates the MySQL database table users
, with ID
and name
field.
The above is the detailed content of Detailed explanation of Golang technology stack: tools, frameworks and libraries. For more information, please follow other related articles on the PHP Chinese website!
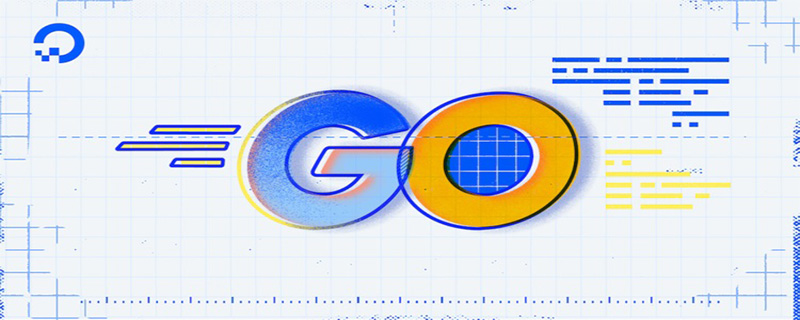
go语言有缩进。在go语言中,缩进直接使用gofmt工具格式化即可(gofmt使用tab进行缩进);gofmt工具会以标准样式的缩进和垂直对齐方式对源代码进行格式化,甚至必要情况下注释也会重新格式化。
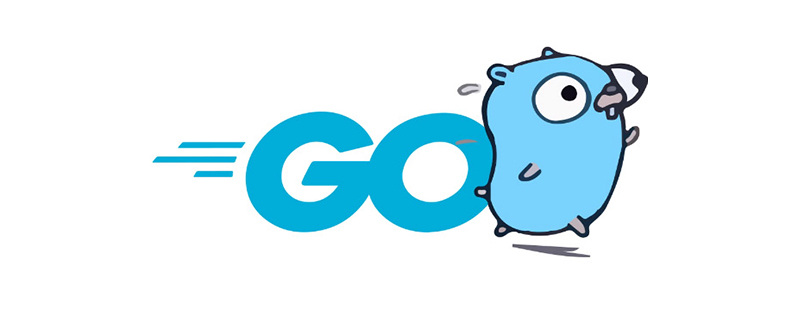
本篇文章带大家了解一下golang 的几种常用的基本数据类型,如整型,浮点型,字符,字符串,布尔型等,并介绍了一些常用的类型转换操作。
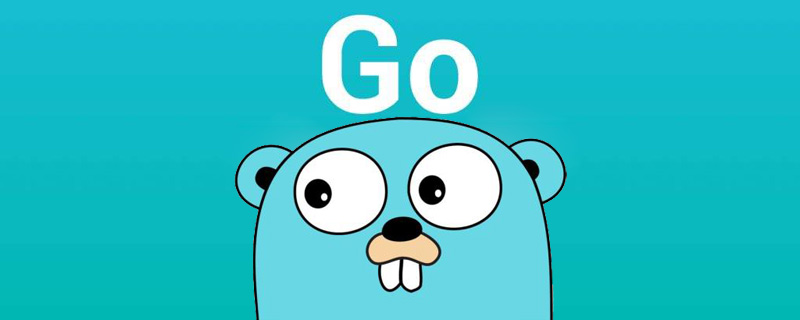
go语言叫go的原因:想表达这门语言的运行速度、开发速度、学习速度(develop)都像gopher一样快。gopher是一种生活在加拿大的小动物,go的吉祥物就是这个小动物,它的中文名叫做囊地鼠,它们最大的特点就是挖洞速度特别快,当然可能不止是挖洞啦。
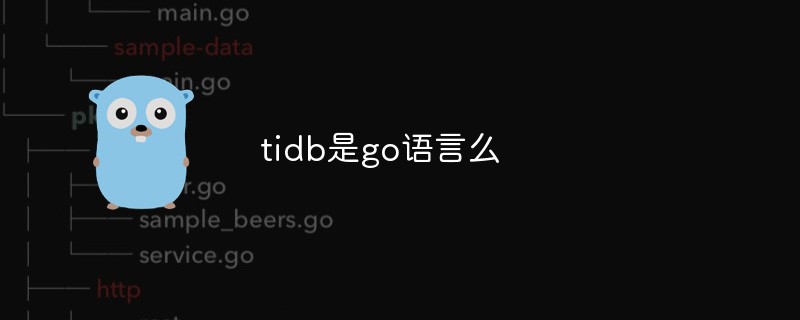
是,TiDB采用go语言编写。TiDB是一个分布式NewSQL数据库;它支持水平弹性扩展、ACID事务、标准SQL、MySQL语法和MySQL协议,具有数据强一致的高可用特性。TiDB架构中的PD储存了集群的元信息,如key在哪个TiKV节点;PD还负责集群的负载均衡以及数据分片等。PD通过内嵌etcd来支持数据分布和容错;PD采用go语言编写。
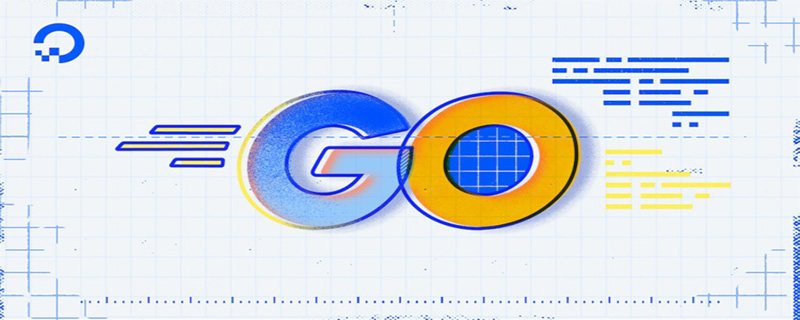
在写 Go 的过程中经常对比这两种语言的特性,踩了不少坑,也发现了不少有意思的地方,下面本篇就来聊聊 Go 自带的 HttpClient 的超时机制,希望对大家有所帮助。
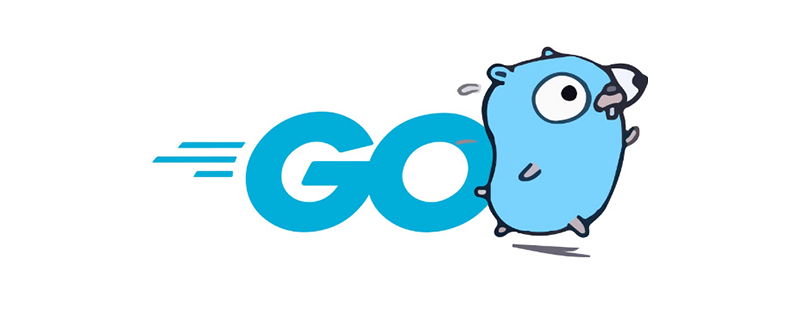
go语言需要编译。Go语言是编译型的静态语言,是一门需要编译才能运行的编程语言,也就说Go语言程序在运行之前需要通过编译器生成二进制机器码(二进制的可执行文件),随后二进制文件才能在目标机器上运行。
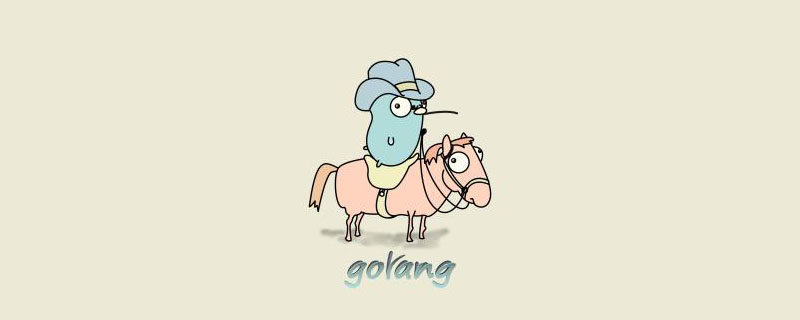
删除map元素的两种方法:1、使用delete()函数从map中删除指定键值对,语法“delete(map, 键名)”;2、重新创建一个新的map对象,可以清空map中的所有元素,语法“var mapname map[keytype]valuetype”。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
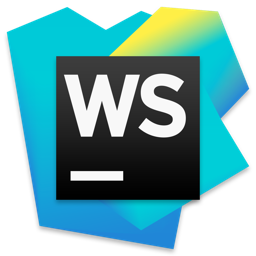
WebStorm Mac version
Useful JavaScript development tools
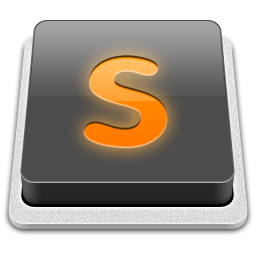
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 Chinese version
Chinese version, very easy to use

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
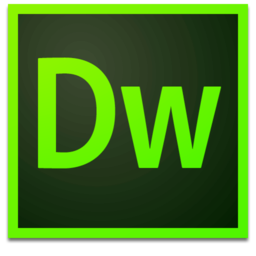
Dreamweaver Mac version
Visual web development tools
