


In Golang, use lambda expressions to implement functional programming: Lambda expressions: anonymous functions, passing functions as parameters, used to simplify the use of higher-order functions; closures: functions that capture data from surrounding functions, access creation variables in the environment. Practical cases: Concurrency processing: use lambda expressions to process tasks in parallel to improve performance; state management: use closures to create functions containing state, track and maintain across calls or modules.
Functional Programming in Golang: Exploring the Concepts of Lambdas and Closures
Introduction
Functional programming is a programming paradigm that emphasizes functions as first-class citizens. In Golang, lambda expressions and closures allow us to write code in a functional style. This article will explore both concepts and their application in real projects.
Lambda expressions
Lambda expressions are anonymous functions that allow us to pass functions as arguments in a concise way. They are typically used to pass functionality to higher-order functions such as map
or filter
.
Syntax:
func(parameters) return_values { // 函数体 }
Example:
numbers := []int{1, 2, 3, 4, 5} // 使用 lambda 表达式来平方每个数字 squaredNumbers := map(numbers, func(x int) int { return x * x })
Closure
A closure is surrounded by Function A function that captures data. Closures enable us to access variables in the environment in which they were created, even after the containing function has returned.
Grammar:
func outerFunc(x int) func() { // 捕获 x return func() { fmt.Println(x) // 内部函数可以访问 x } }
Example:
// 表示一个计数器 increment := outerFunc(0) // 每次调用都会对计数进行递增 for i := 0; i < 5; i++ { increment() // 输出 1, 2, 3, 4, 5 }
Practical case
Use lambda expression Expressions and Concurrency
Lambda expressions can be used with concurrency to process tasks in parallel, thereby improving performance. For example:
func main() { numbers := []int{1, 2, 3, 4, 5} resultChan := make(chan int) // 使用 lambda 并行处理任务 for _, number := range numbers { go func(num int) { resultChan <- num * num }(number) } // 收集并输出结果 for i := 0; i < len(numbers); i++ { fmt.Println(<-resultChan) } }
Using Closures for State Management
Closures can be used to create functions that contain state even after the containing function returns. This is useful in situations where you need to track or maintain state across multiple calls or modules.
func makeCounter() func() int { var counter int // 内部函数捕获 counter return func() int { counter++ return counter } } func main() { // 创建两个计数器 counterA := makeCounter() counterB := makeCounter() // 调用计数器以递增状态 for i := 0; i < 5; i++ { fmt.Println("Counter A:", counterA()) fmt.Println("Counter B:", counterB()) } }
The above is the detailed content of Golang Functional Programming: Exploring the Concepts of Lambdas and Closures. For more information, please follow other related articles on the PHP Chinese website!
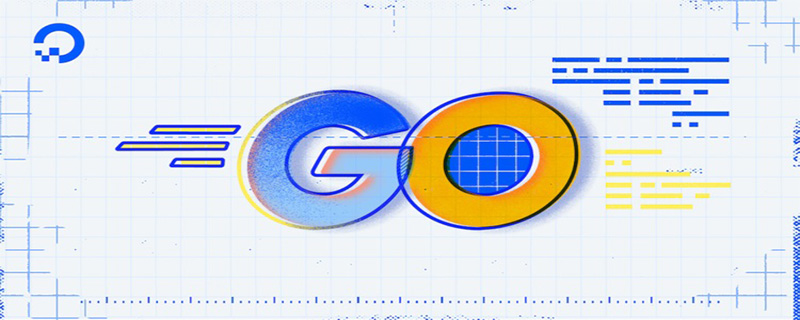
go语言有缩进。在go语言中,缩进直接使用gofmt工具格式化即可(gofmt使用tab进行缩进);gofmt工具会以标准样式的缩进和垂直对齐方式对源代码进行格式化,甚至必要情况下注释也会重新格式化。
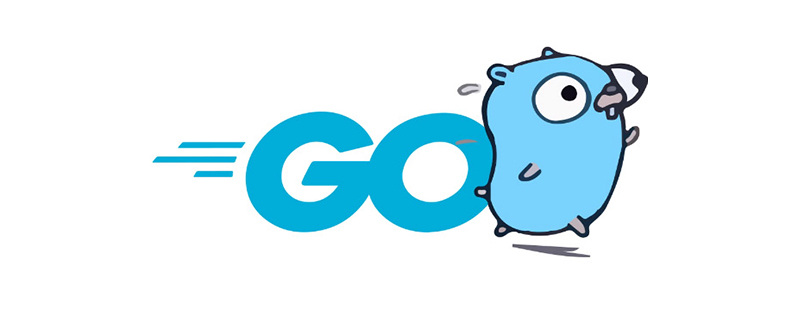
本篇文章带大家了解一下golang 的几种常用的基本数据类型,如整型,浮点型,字符,字符串,布尔型等,并介绍了一些常用的类型转换操作。
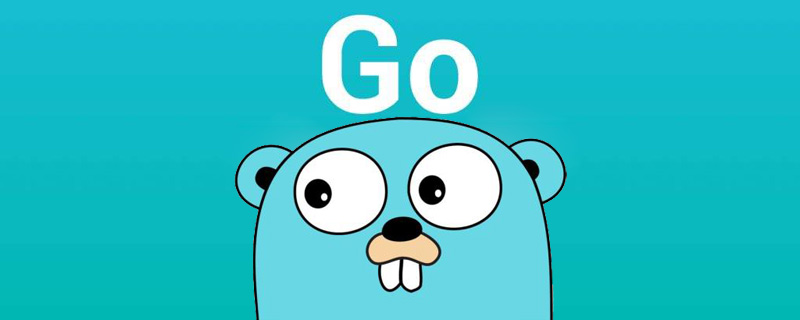
go语言叫go的原因:想表达这门语言的运行速度、开发速度、学习速度(develop)都像gopher一样快。gopher是一种生活在加拿大的小动物,go的吉祥物就是这个小动物,它的中文名叫做囊地鼠,它们最大的特点就是挖洞速度特别快,当然可能不止是挖洞啦。
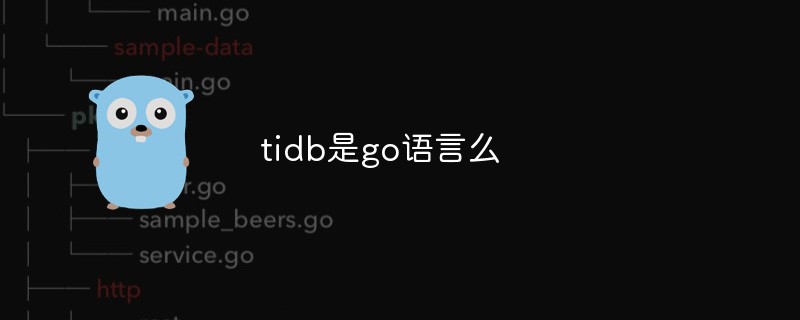
是,TiDB采用go语言编写。TiDB是一个分布式NewSQL数据库;它支持水平弹性扩展、ACID事务、标准SQL、MySQL语法和MySQL协议,具有数据强一致的高可用特性。TiDB架构中的PD储存了集群的元信息,如key在哪个TiKV节点;PD还负责集群的负载均衡以及数据分片等。PD通过内嵌etcd来支持数据分布和容错;PD采用go语言编写。
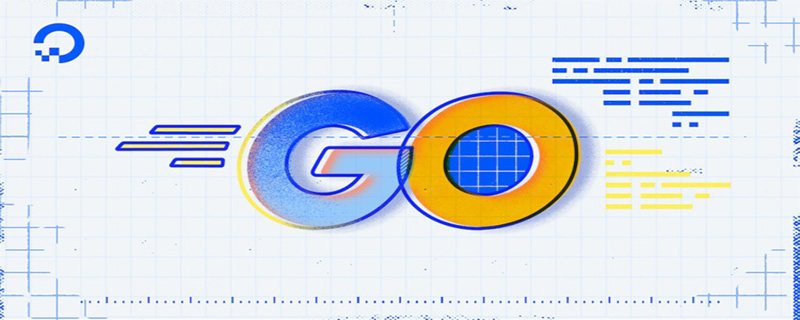
在写 Go 的过程中经常对比这两种语言的特性,踩了不少坑,也发现了不少有意思的地方,下面本篇就来聊聊 Go 自带的 HttpClient 的超时机制,希望对大家有所帮助。
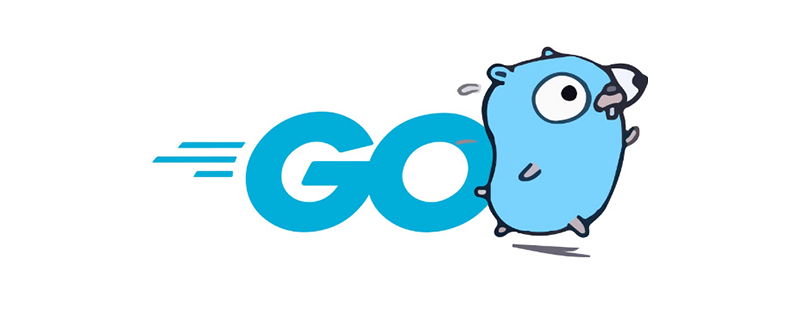
go语言需要编译。Go语言是编译型的静态语言,是一门需要编译才能运行的编程语言,也就说Go语言程序在运行之前需要通过编译器生成二进制机器码(二进制的可执行文件),随后二进制文件才能在目标机器上运行。
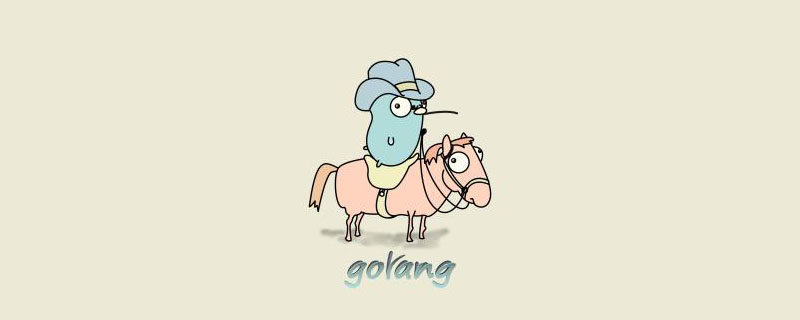
删除map元素的两种方法:1、使用delete()函数从map中删除指定键值对,语法“delete(map, 键名)”;2、重新创建一个新的map对象,可以清空map中的所有元素,语法“var mapname map[keytype]valuetype”。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
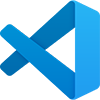
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Notepad++7.3.1
Easy-to-use and free code editor
