


C++ algorithm efficiency and performance optimization in IoT and embedded systems
In the Internet of Things and embedded systems, the efficiency optimization of C algorithms includes: selecting appropriate data structures, using loop optimization and algorithm divide and conquer. Performance optimizations include managing memory usage, leveraging hardware features, and reducing function calls. Practical examples include image processing on embedded devices, data routing for wireless sensor networks, and machine learning model inference on IoT gateways. These optimization techniques maximize algorithm efficiency and performance and are critical for developing reliable and efficient embedded systems.
C Algorithm Efficiency and Performance Optimization in IoT and Embedded Systems
Introduction
In Internet of Things (IoT) and embedded systems, algorithm performance and efficiency are critical. C is one of the programming languages of choice for these systems due to its speed, resource management capabilities, and memory safety features.
Optimize algorithm efficiency
-
Use appropriate data structures: Choose data structures suitable for specific algorithm operations, such as arrays , linked list or hash table.
// 使用数组存储连续值 int values[] = {1, 2, 3, 4, 5}; // 使用链表存储可变长度的元素 struct Node { int value; Node* next; }; Node* head = new Node{1, new Node{2, new Node{3, nullptr}}};
-
Take advantage of loop optimization: Eliminate unnecessary loops and use optimizing compiler options to improve loop efficiency.
// 优化循环条件 for (int i = 0; i < n; ++i) {} // > // 优化循环变量类型 for (unsigned int i = 0; i < n; ++i) {} // >
-
Algorithm Divide and Conquer: Divide a complex algorithm into smaller sub-problems and solve them recursively or iteratively.
int binarySearch(int* arr, int low, int high, int target) { if (low > high) return -1; int mid = (low + high) / 2; if (arr[mid] == target) return mid; else if (arr[mid] > target) return binarySearch(arr, low, mid - 1, target); else return binarySearch(arr, mid + 1, high, target); }
Optimize performance
##Manage memory usage: Carefully manage memory allocation and release, Avoid memory leaks and fragmentation.
// 使用智能指针自动管理内存 std::unique_ptr<int> ptr = std::make_unique<int>(5);
Use Hardware Features: Take advantage of hardware features such as parallel processing or specific instruction sets.
// 利用 SIMD 指令进行并行计算 __m128i a = _mm_loadu_si128(array); __m128i b = _mm_loadu_si128(array2); __m128i c = _mm_add_epi32(a, b);
Reduce function calls: Function calls have overhead, try to reduce their use.
// 展开递归函数 void recursiveFunction(int n) { if (n == 0) return; recursiveFunction(n - 1); }
Practical case
In the following cases, algorithm efficiency and performance optimization are applied:- Embedding Image processing algorithms on conventional devices
- Data routing protocols in wireless sensor networks
- Machine learning model inference on IoT gateways
Conclusion
By adopting these optimization techniques, the efficiency and performance of C algorithms can be maximized in IoT and embedded systems. This is critical for developing reliable, efficient embedded systems with limited resources.The above is the detailed content of C++ algorithm efficiency and performance optimization in IoT and embedded systems. For more information, please follow other related articles on the PHP Chinese website!
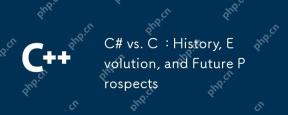
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
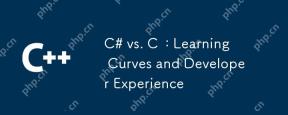
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
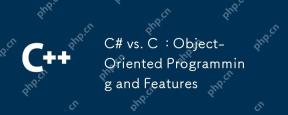
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
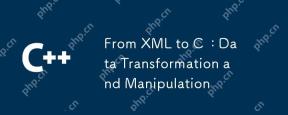
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
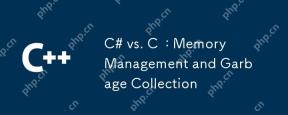
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
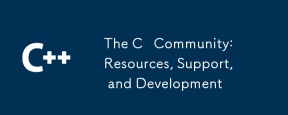
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
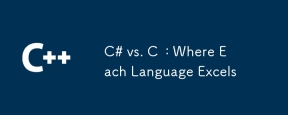
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
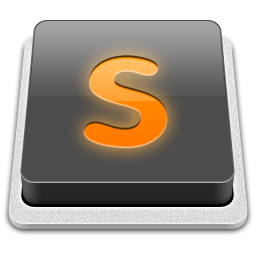
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 English version
Recommended: Win version, supports code prompts!

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.