


Deadlock prevention and detection mechanism in C++ multi-threaded programming
Multi-thread deadlock prevention mechanism includes: 1. Lock sequence; 2. Test and set up. Detection mechanisms include: 1. Timeout; 2. Deadlock detector. The article takes an example of a shared bank account and avoids deadlock through lock sequence. The transfer function first requests the lock of the transfer out account and then the transfer in account.
Deadlock prevention and detection mechanism in C++ multi-threaded programming
In a multi-threaded environment, deadlock is a common errors that may cause the program to stop responding. A deadlock occurs when multiple threads wait indefinitely for each other to release their locks, creating a waiting loop.
In order to avoid and detect deadlocks, C++ provides several mechanisms:
Prevention mechanism
- Lock order: Develop a strict request lock order for all shared mutable data to ensure that all threads always request locks in the same order.
-
Test and set: Use
std::atomic
provided by thestd::atomic
library to test and set variables to check whether the lock has been request and then set it up immediately.
Detection mechanism
- Timeout:Set a timeout for the lock request. If the lock is not obtained after the time, then Throw an exception or take other appropriate action.
- Deadlock Detector: Use third-party libraries such as Boost.Thread to monitor thread activity, detect deadlocks and take necessary actions.
Practical case:
Consider the following shared bank account example:
class BankAccount { private: std::mutex m_; int balance_; public: void deposit(int amount) { std::lock_guard<std::mutex> lock(m_); balance_ += amount; } bool withdraw(int amount) { std::lock_guard<std::mutex> lock(m_); if (balance_ >= amount) { balance_ -= amount; return true; } return false; } };
The way to avoid deadlock is to use lock order: request first deposit()
lock, and then request withdraw()
lock.
void transfer(BankAccount& from, BankAccount& to, int amount) { std::lock_guard<std::mutex> fromLock(from.m_); std::lock_guard<std::mutex> toLock(to.m_); if (from.withdraw(amount)) { to.deposit(amount); } }
Deadlock can be prevented by requesting locks in the order of transfers.
The above is the detailed content of Deadlock prevention and detection mechanism in C++ multi-threaded programming. For more information, please follow other related articles on the PHP Chinese website!
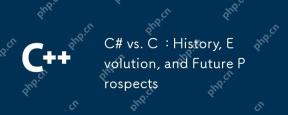
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
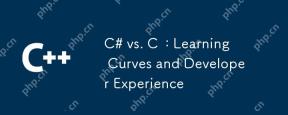
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
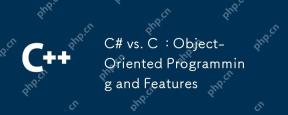
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
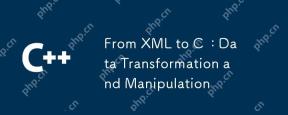
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
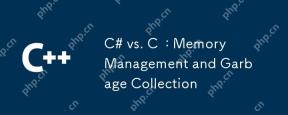
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
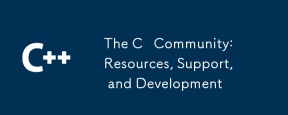
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
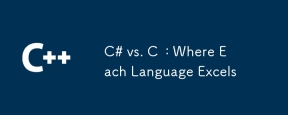
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Linux new version
SublimeText3 Linux latest version

SublimeText3 Chinese version
Chinese version, very easy to use

Atom editor mac version download
The most popular open source editor
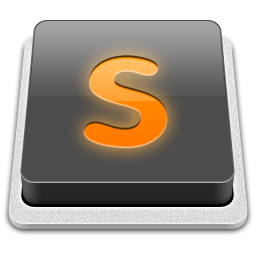
SublimeText3 Mac version
God-level code editing software (SublimeText3)