


Performance comparison of popular libraries and frameworks in the C++ ecosystem
Libraries and frameworks vary in performance across the C++ ecosystem: Boost excels in vector and string processing. Eigen is the most efficient among matrix operations. fmt provides the fastest string formatting. Protobuf takes the lead in binary serialization.
Performance comparison of popular libraries and frameworks in the C++ ecosystem
Introduction
C++ is a powerful programming language with a rich ecosystem of libraries and frameworks that simplify development, improve code quality, and optimize performance. This article will explore the performance differences of several popular C++ libraries and frameworks and illustrate them with practical examples.
Benchmark.js
For performance comparison, we will use Benchmark.js, a library for Node.js and browser performance testing. This library provides an easy-to-use API for creating and running benchmarks.
Libraries and frameworks participating in the test
- Boost: A set of cross-platform C++ libraries that provide various functions.
- Eigen: A line generation library optimized for numerically intensive calculations.
- fmt: A fast and efficient formatting library.
- Protobuf: A binary protocol for data serialization and deserialization.
Practical case
We will compare the performance of these libraries and frameworks in the following scenarios:
- Vector calculation
- Matrix Multiplication
- String Formatting
- Binary Serialization
##Code Example
Vector calculation
#include <iostream> #include <vector> #include <benchmark/benchmark.h> using namespace std; void BM_VectorSum(benchmark::State& state) { vector<double> v(state.range(0)); for (auto _ : state) { double sum = 0; for (auto x : v) { sum += x; } } }
Matrix multiplication
#include <iostream> #include <Eigen/Dense> #include <benchmark/benchmark.h> using namespace Eigen; void BM_MatrixMult(benchmark::State& state) { MatrixXd A = MatrixXd::Random(state.range(0), state.range(1)); MatrixXd B = MatrixXd::Random(state.range(1), state.range(2)); for (auto _ : state) { MatrixXd C = A * B; } }
String formatting
#include <iostream> #include <fmt/core.h> #include <benchmark/benchmark.h> using namespace fmt; void BM_StringFormat(benchmark::State& state) { for (auto _ : state) { format("Hello, {}!", "World"); } }
Binary sequence ization
#include <iostream> #include <google/protobuf/message.h> #include <benchmark/benchmark.h> using namespace google::protobuf; class MyMessage : public Message { public: int32_t id; string name; }; void BM_ProtobufSerialize(benchmark::State& state) { MyMessage msg; msg.id = 1; msg.name = "John"; for (auto _ : state) { msg.SerializeToString(); } }
Result Analysis
Benchmark results may vary depending on system configuration and compiler optimizations. However, in general, we observe the following results:- Boost performs well on vector and string processing.
- Eigen is the most efficient among matrix operations.
- fmt provides the fastest string formatting.
- Protobuf stands out in binary serialization.
Conclusion
This article demonstrated the performance differences of popular libraries and frameworks in the C++ ecosystem. Through practical cases, we see which library or framework is most suitable in different scenarios. This helps developers make informed decisions in performance-critical applications.The above is the detailed content of Performance comparison of popular libraries and frameworks in the C++ ecosystem. For more information, please follow other related articles on the PHP Chinese website!
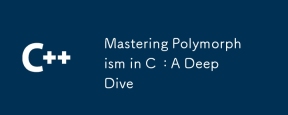
Mastering polymorphisms in C can significantly improve code flexibility and maintainability. 1) Polymorphism allows different types of objects to be treated as objects of the same base type. 2) Implement runtime polymorphism through inheritance and virtual functions. 3) Polymorphism supports code extension without modifying existing classes. 4) Using CRTP to implement compile-time polymorphism can improve performance. 5) Smart pointers help resource management. 6) The base class should have a virtual destructor. 7) Performance optimization requires code analysis first.
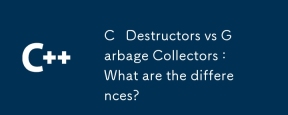
C destructorsprovideprecisecontroloverresourcemanagement,whilegarbagecollectorsautomatememorymanagementbutintroduceunpredictability.C destructors:1)Allowcustomcleanupactionswhenobjectsaredestroyed,2)Releaseresourcesimmediatelywhenobjectsgooutofscop
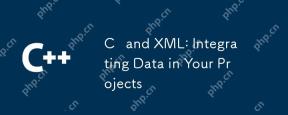
Integrating XML in a C project can be achieved through the following steps: 1) parse and generate XML files using pugixml or TinyXML library, 2) select DOM or SAX methods for parsing, 3) handle nested nodes and multi-level properties, 4) optimize performance using debugging techniques and best practices.
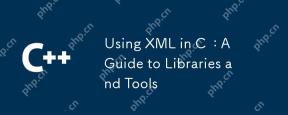
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
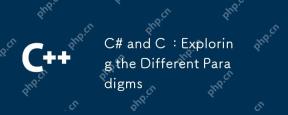
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
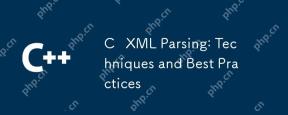
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
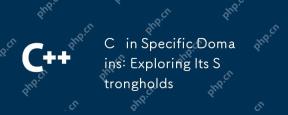
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
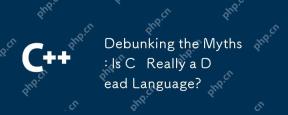
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
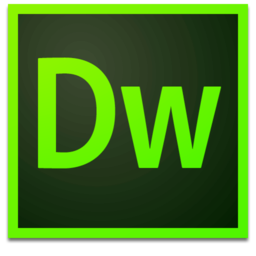
Dreamweaver Mac version
Visual web development tools
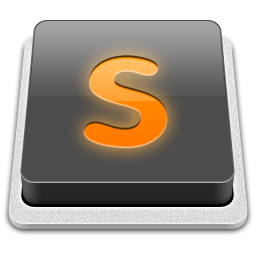
SublimeText3 Mac version
God-level code editing software (SublimeText3)
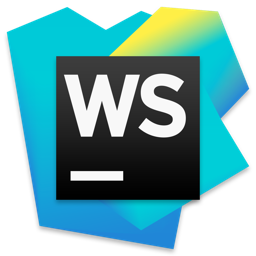
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
