


Leveraging C++ coroutines can greatly improve the responsiveness of your server architecture because it allows you to write asynchronous code that asynchronousizes blocking I/O operations. For example, a network server can handle requests asynchronously by using coroutines for network I/O. Additionally, coroutines can be used to optimize distributed systems and game development.
Use C++ coroutine to optimize the response speed of the server architecture
Coroutine is a lightweight user space thread. Allows you to write asynchronous code without using threads or callbacks. This can greatly improve the responsiveness of your server application, especially when handling large numbers of concurrent requests.
C++ Coroutine
C++ 20 introduces the coroutine library and provides support for coroutines. Coroutines are declared using co_return
and co_await
, which allow you to pause and resume coroutine execution.
struct MyCoroutine { bool done = false; int result; void main() { co_await std::async(slow_function); co_return result; } };
Optimize server architecture
You can use coroutines to optimize your server architecture by asynchronously making blocking I/O operations. For example, a network server can handle requests asynchronously by using coroutines for network I/O.
struct HttpServer { void start() { auto tasks = std::vector<std::coroutine_handle<int>>{}; while (true) { auto client_socket = accept_client(); tasks.push_back(std::coroutine_handle<int>(handle_client(client_socket))); } // 处理所有挂起的协程 for (auto& task : tasks) { task.resume(); } } std::coroutine_handle<int> handle_client(int client_socket) { // 读取请求 auto request = co_await read_request(client_socket); // 处理请求 auto response = process_request(request); // 写入响应 co_await write_response(client_socket, response); } };
Practical case
The following is a practical case of using coroutine to optimize the server architecture:
- Network server:Coroutines can be used to process network requests asynchronously to improve the throughput and response speed of the server.
- Distributed systems: Coroutines can be used to coordinate different components of a distributed system, reducing latency and improving throughput.
- Game development: Coroutines can be used to implement asynchronous game logic and provide a smooth and responsive game experience.
Conclusion
By leveraging C++ coroutines, you can optimize your server architecture, improve response times, and handle more concurrent requests. Coroutines provide a safe and efficient asynchronous programming method to help you create efficient and scalable applications.
The above is the detailed content of Optimizing the responsiveness of server architectures using C++ coroutines. For more information, please follow other related articles on the PHP Chinese website!
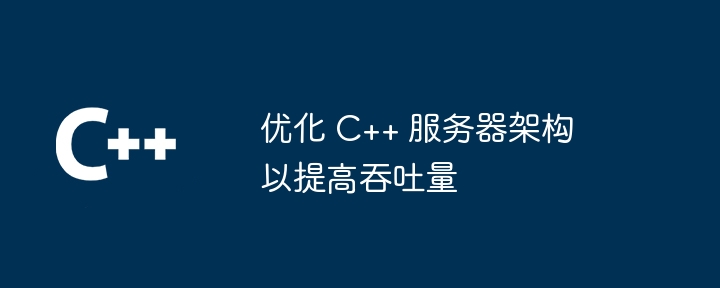
优化C++服务器吞吐量策略:线程池:预先创建线程池,快速响应请求。非阻塞I/O:在等待I/O时执行其他任务,提升吞吐量。HTTP/2:使用二进制协议,支持多路复用和内容压缩,提高性能。
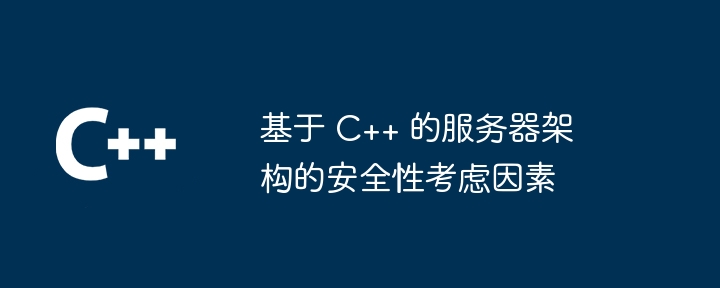
在设计基于C++的服务器架构时,安全考虑至关重要:使用std::string或std::vector避免缓冲区溢出。使用正则表达式或库函数验证用户输入。采用输出转义防止跨站点脚本(XSS)。预编译语句或参数化查询防止SQL注入。
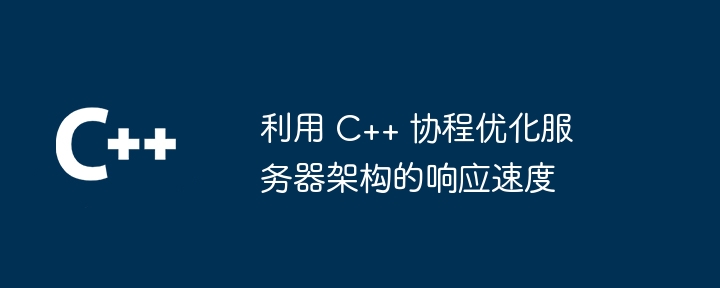
利用C++协程可以极大地提高服务器架构的响应速度,因为它允许您编写异步代码,将阻塞I/O操作异步化。例如,网络服务器可以通过使用协程进行网络I/O来实现异步处理请求。此外,协程还可用于优化分布式系统和游戏开发。
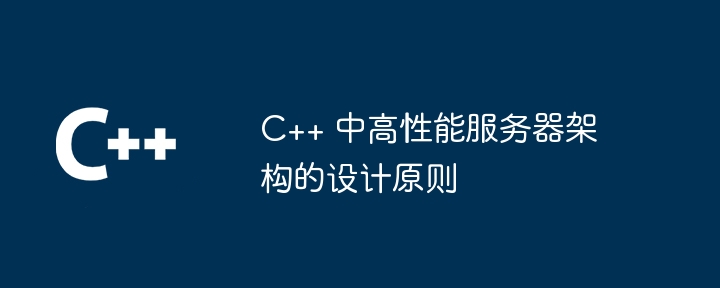
C++高性能服务器架构的设计原则包括:选择合适的线程模型(单线程、多线程或事件驱动)使用非阻塞I/O技术(select()、poll()、epoll())优化内存管理(避免泄漏、碎片化,使用智能指针、内存池)关注实战案例(例如使用BoostAsio实现非阻塞I/O模型和内存池管理连接)
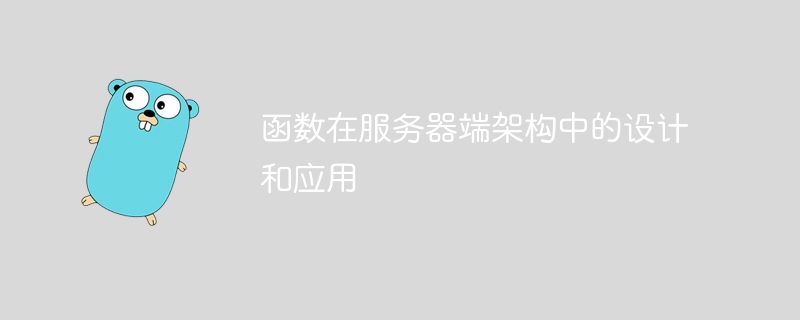
函数在服务器端架构中扮演着至关重要的角色,可以提升代码可读性、可测试性和可维护性,遵循单一职责、松散耦合、可重用性、可测试性和错误处理等设计原则,典型应用包括数据处理、API端点、事件处理、定时作业和消息队列处理。例如,使用Express.js,我们创建了一个简单的函数,当客户端发送GET请求到/hello路由时,返回Hello,world!。
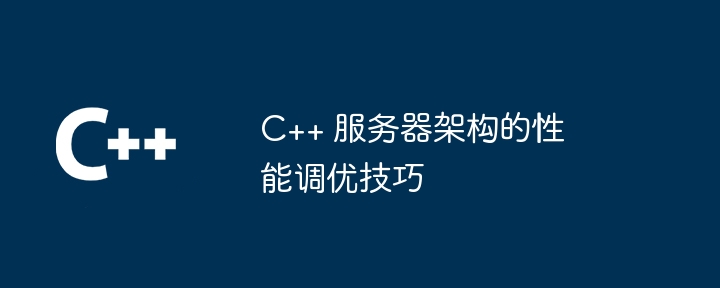
优化C++服务器架构性能的技巧:使用多线程:创建和管理线程,以并行处理请求,提高并发性。采用非阻塞I/O:使用事件驱动模型,执行非阻塞操作,防止I/O瓶颈。优化内存管理:使用内存池或智能指针,减少内存分配和释放成本。避免使用全局变量、优化数据结构、使用性能分析工具、使用缓存和监控服务器状态以进一步提升性能。
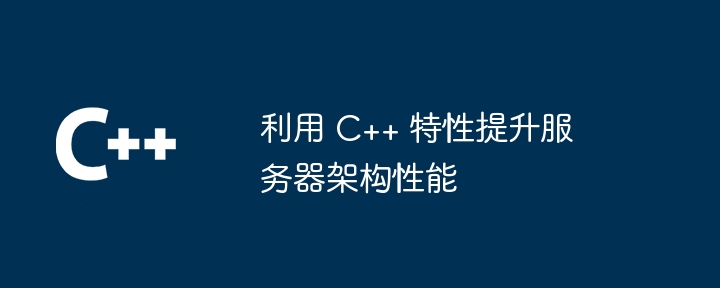
充分利用C++特性,如并发性、模板编程和内存管理,可以显著提高服务器架构性能:1.通过使用线程和多线程,可以并行执行多个任务,提高吞吐量。2.模板编程可以在编译时生成代码,避免运行时开销。3.细粒度的内存管理可以优化内存分配和避免碎片化,提高内存效率。
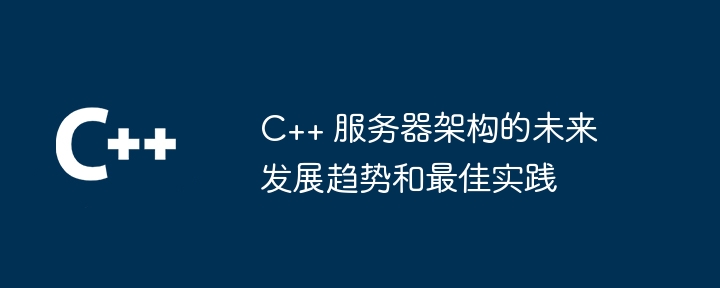
未来C++服务器架构趋势包括:异步和非阻塞编程可提升性能;微服务架构提高可扩展性和灵活性;云原生设计带来无状态性和可观测性。最佳实践包括:使用libcuckoo优化数据存储;采用tcmalloc提升内存管理;运用RAII防止内存泄漏;通过性能分析工具优化效率。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Linux new version
SublimeText3 Linux latest version
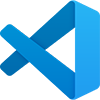
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
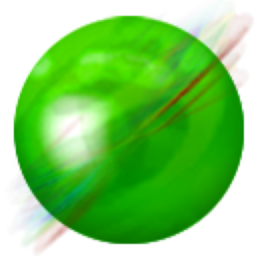
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
