Passing errors through pipelines can efficiently expose operation errors to the outside, so that they can be processed uniformly in business logic. The specific usage is as follows: type the pipeline and clearly convey the type of data and error type. Use a typed result structure on the sender side to pass data and errors. Use type assertions on the receiver side to receive data and errors from the pipeline and handle them based on whether there are errors.
How to use pipes in Go language to handle errors
Pipelines are a concurrent communication mechanism that allows goroutines to Pass data efficiently. Pipelines can not only transfer data, but also errors, allowing us to expose operational errors to the outside through pipelines, process them uniformly in business logic, and improve development efficiency.
Usage method
When using a pipeline to transmit errors, you need to type the pipeline and clarify the type of data passed in the pipeline, including the data type and error type. As shown in the following example:
type result struct { data int err error } var ch = make(chan result)
Practical case
The following is a practical case showing how to use pipes to pass errors:
package main import ( "errors" "fmt" "time" ) func task(ch chan<- result) { time.Sleep(time.Second) if n := rand.Intn(5); n % 2 == 0 { ch <- result{n, nil} } else { ch <- result{0, errors.New("error occurred")} } } func main() { ch := make(chan result) defer close(ch) go task(ch) select { case r := <-ch: if r.err != nil { fmt.Println("Error: ", r.err) } else { fmt.Println("Data: ", r.data) } case <-time.After(time.Second * 2): fmt.Println("Timeout") } }
Run Result:
Error: error occurred
In this example, the task
function simulates an asynchronous task using random numbers. If the random number is even, it will send data without any errors; otherwise, it will send an error. main
The function receives the results from the pipe and handles them based on whether there are errors.
Note:
- Make sure the pipe is typed correctly to avoid type conversion errors.
- Always check data received from a pipe for errors, even if you think it won't.
- Use the
select
statement to process data from multiple pipelines, or to handle pipeline timeouts.
The above is the detailed content of How to handle errors using pipes in Go?. For more information, please follow other related articles on the PHP Chinese website!
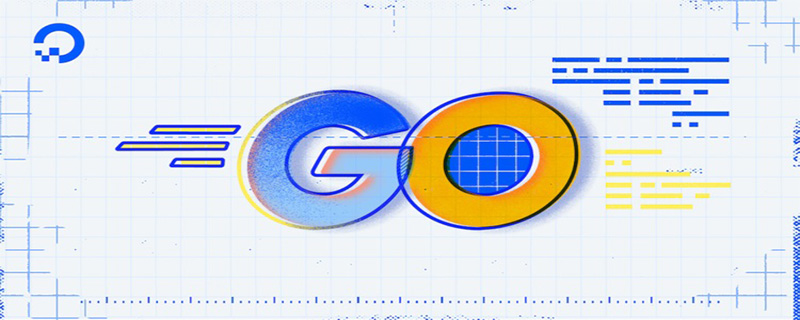
go语言有缩进。在go语言中,缩进直接使用gofmt工具格式化即可(gofmt使用tab进行缩进);gofmt工具会以标准样式的缩进和垂直对齐方式对源代码进行格式化,甚至必要情况下注释也会重新格式化。
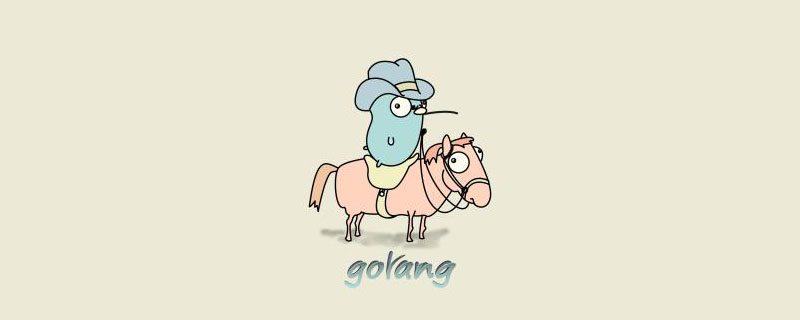
闭包(closure)是一个函数以及其捆绑的周边环境状态(lexical environment,词法环境)的引用的组合。 换而言之,闭包让开发者可以从内部函数访问外部函数的作用域。 闭包会随着函数的创建而被同时创建。
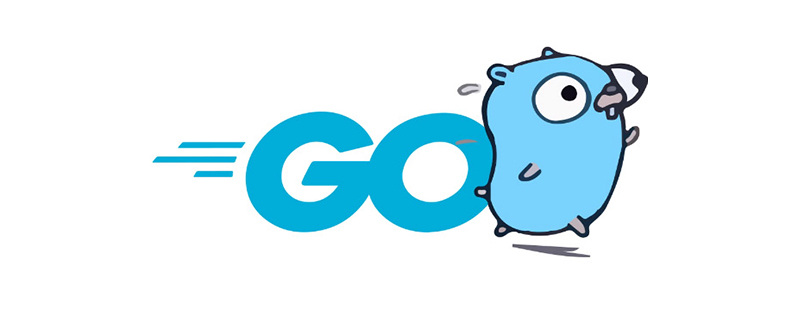
本篇文章带大家了解一下golang 的几种常用的基本数据类型,如整型,浮点型,字符,字符串,布尔型等,并介绍了一些常用的类型转换操作。
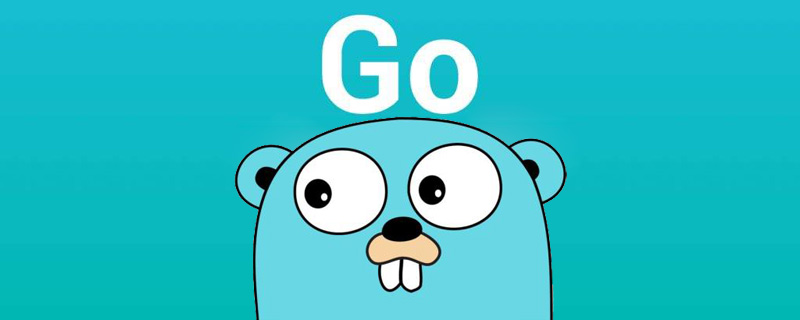
go语言叫go的原因:想表达这门语言的运行速度、开发速度、学习速度(develop)都像gopher一样快。gopher是一种生活在加拿大的小动物,go的吉祥物就是这个小动物,它的中文名叫做囊地鼠,它们最大的特点就是挖洞速度特别快,当然可能不止是挖洞啦。
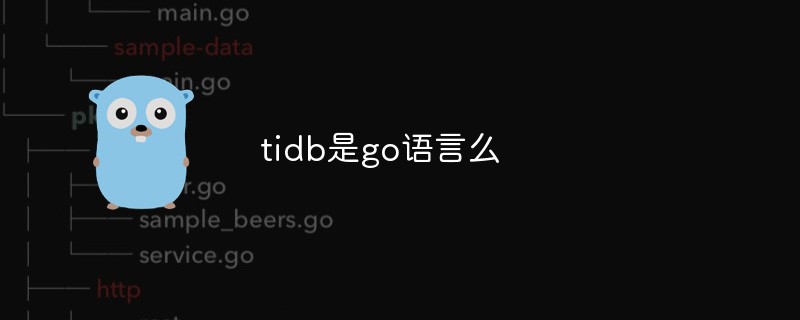
是,TiDB采用go语言编写。TiDB是一个分布式NewSQL数据库;它支持水平弹性扩展、ACID事务、标准SQL、MySQL语法和MySQL协议,具有数据强一致的高可用特性。TiDB架构中的PD储存了集群的元信息,如key在哪个TiKV节点;PD还负责集群的负载均衡以及数据分片等。PD通过内嵌etcd来支持数据分布和容错;PD采用go语言编写。
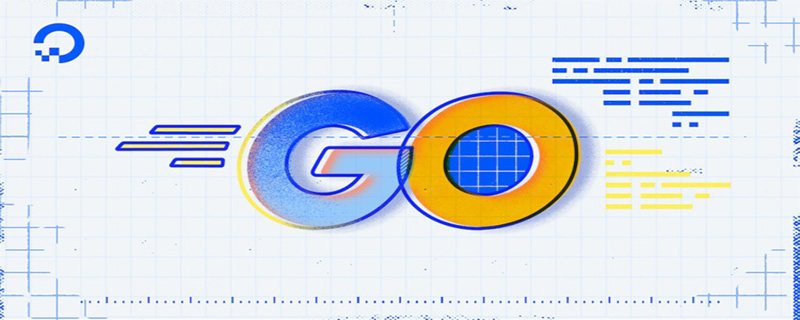
在写 Go 的过程中经常对比这两种语言的特性,踩了不少坑,也发现了不少有意思的地方,下面本篇就来聊聊 Go 自带的 HttpClient 的超时机制,希望对大家有所帮助。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
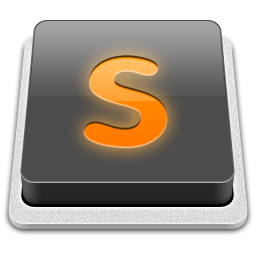
SublimeText3 Mac version
God-level code editing software (SublimeText3)

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
