The following emerging frameworks in the Go language ecosystem are worth watching: gRPC: A high-performance RPC framework using the HTTP/2 protocol. Echo: An easy-to-use, flexible web framework that supports modern web technologies. Beego: A full-stack web framework based on the MVC pattern, with CRUD functionality out of the box. Gorilla: A lightweight web toolkit that makes it easy to create HTTP servers and middleware. Iris: A fast, modern application-optimized web framework with outstanding performance.
The emerging Go framework that has attracted much attention
In the booming ecosystem of the Go language, various innovations are constantly emerging Framework that provides developers with the tools to build powerful, efficient applications. Here are some emerging frameworks worth keeping an eye on:
gRPC:
gRPC is a general-purpose remote procedure call (RPC) framework that uses the HTTP/2 protocol communications, delivering superior performance and scalability.
package main import ( "context" "log" "net" "time" pb "github.com/go-grpc-tutorial/protos" "google.golang.org/grpc" ) func main() { lis, err := net.Listen("tcp", ":9000") if err != nil { log.Fatalf("failed to listen: %v", err) } s := grpc.NewServer() pb.RegisterGreeterServer(s, &server{}) if err := s.Serve(lis); err != nil { log.Fatalf("failed to serve: %v", err) } } type server struct { pb.UnimplementedGreeterServer } func (s *server) SayHello(ctx context.Context, in *pb.HelloRequest) (*pb.HelloReply, error) { ctx, cancel := context.WithTimeout(ctx, time.Second) defer cancel() return &pb.HelloReply{Message: "Hello " + in.GetName()}, nil }
Echo:
Echo is a high-performance web framework known for its ease of use, flexibility and support for modern web technologies.
package main import ( "github.com/labstack/echo" ) func main() { e := echo.New() e.GET("/hello", func(c echo.Context) error { return c.String(200, "Hello, World!") }) e.Logger.Fatal(e.Start(":1323")) }
Beego:
Beego is a full-stack web framework built on the MVC pattern, providing out-of-the-box CRUD functionality and other useful features.
package main import ( "github.com/beego/beego/v2/server/web" ) func main() { web.Router("/", &controllers.MainController{}) web.Run() } type controllers.MainController struct { web.Controller } func (c *controllers.MainController) Get() { c.Data["Website"] = "beego.me" c.TplName = "index.tpl" }
Gorilla:
Gorilla is a lightweight web toolkit that provides a set of functions to create HTTP servers and middleware.
package main import ( "net/http" "github.com/gorilla/mux" ) func main() { r := mux.NewRouter() // /hello/{name}/ 路由匹配 r.HandleFunc("/hello/{name}", HelloHandler) http.Handle("/", r) http.ListenAndServe(":8080", nil) } func HelloHandler(w http.ResponseWriter, r *http.Request) { vars := mux.Vars(r) name := vars["name"] w.Write([]byte("Hello, " + name + "!")) }
Iris:
Iris is a fast web framework with great performance and features optimized for modern applications.
package main import ( "github.com/kataras/iris/v12" ) func main() { app := iris.New() app.Get("/", func(ctx iris.Context) { ctx.HTML("<h1 id="Hello-World">Hello, World!</h1>") }) app.Run(iris.Addr(":8080")) }
These frameworks are just some noteworthy examples of Go’s emerging ecosystem, offering a variety of features and benefits to suit different application development needs.
The above is the detailed content of Which emerging Go frameworks are worth paying attention to?. For more information, please follow other related articles on the PHP Chinese website!
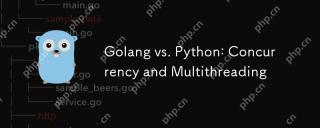
Golang is more suitable for high concurrency tasks, while Python has more advantages in flexibility. 1.Golang efficiently handles concurrency through goroutine and channel. 2. Python relies on threading and asyncio, which is affected by GIL, but provides multiple concurrency methods. The choice should be based on specific needs.
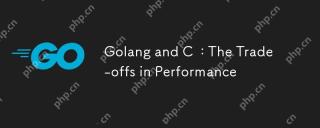
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
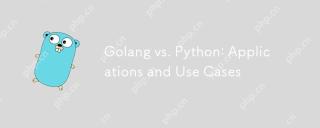
ChooseGolangforhighperformanceandconcurrency,idealforbackendservicesandnetworkprogramming;selectPythonforrapiddevelopment,datascience,andmachinelearningduetoitsversatilityandextensivelibraries.

Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
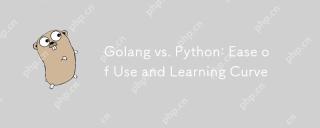
In what aspects are Golang and Python easier to use and have a smoother learning curve? Golang is more suitable for high concurrency and high performance needs, and the learning curve is relatively gentle for developers with C language background. Python is more suitable for data science and rapid prototyping, and the learning curve is very smooth for beginners.
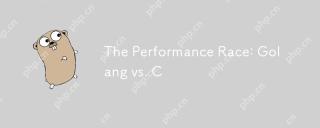
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
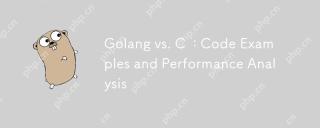
Golang is suitable for rapid development and concurrent programming, while C is more suitable for projects that require extreme performance and underlying control. 1) Golang's concurrency model simplifies concurrency programming through goroutine and channel. 2) C's template programming provides generic code and performance optimization. 3) Golang's garbage collection is convenient but may affect performance. C's memory management is complex but the control is fine.
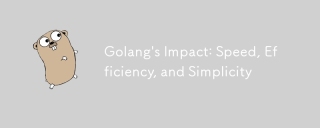
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Zend Studio 13.0.1
Powerful PHP integrated development environment
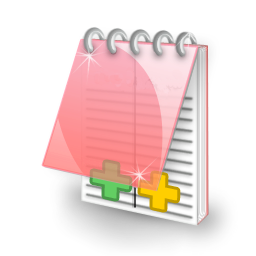
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 English version
Recommended: Win version, supports code prompts!

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.