Retrieving JSON data from the database in Golang can be done through the following steps: use the sql.RawBytes type to store JSON data; execute a query and scan the data into the sql.RawBytes variable; use the json.Unmarshal function to deserialize the JSON data.
How to retrieve JSON data from database in Golang
In Golang, you can use sql.RawBytes type to store and retrieve JSON data. This approach allows you to store JSON data as binary data in the database and then deserialize it into a JSON object upon retrieval.
Here are the steps on how to retrieve JSON data from database in Golang:
1. Install necessary libraries
import ( "database/sql" _ "github.com/go-sql-driver/mysql" // 数据库驱动程序(根据需要更改) "encoding/json" )
2. Open a database connection
db, err := sql.Open("mysql", "user:password@tcp(host:port)/database") if err != nil { // 处理错误 }
3. Execute the query
To retrieve JSON data from the database, use the sql.RawBytes
type.
rows, err := db.Query("SELECT json_column FROM table_name") if err != nil { // 处理错误 }
4. Scan rows
For each row of the query, use the Scan
method to scan the json_column
column tosql.RawBytes
type variable.
var jsonBytes []byte err = rows.Scan(&jsonBytes) if err != nil { // 处理错误 }
5. Deserializing JSON data
To deserialize binary JSON data into a JSON object, use the json.Unmarshal
function .
var jsonObject map[string]interface{} err = json.Unmarshal(jsonBytes, &jsonObject) if err != nil { // 处理错误 }
Practical case
Suppose you have a table named users
, which has a table named json_data
JSON
columns. To retrieve the json_data
column from the users
table:
rows, err := db.Query("SELECT json_data FROM users") if err != nil { // 处理错误 } for rows.Next() { var jsonBytes []byte err = rows.Scan(&jsonBytes) if err != nil { // 处理错误 } var jsonObject map[string]interface{} err = json.Unmarshal(jsonBytes, &jsonObject) if err != nil { // 处理错误 } fmt.Println(jsonObject) }
The above code will iterate through each row in the users
table and replace the json_data
Deserialize the column into a JSON object and print it to the console.
The above is the detailed content of How to retrieve JSON data from database in Golang?. For more information, please follow other related articles on the PHP Chinese website!

本篇文章给大家带来了关于mysql的相关知识,其中主要介绍了关于索引优化器工作原理的相关内容,其中包括了MySQL Server的组成,MySQL优化器选择索引额原理以及SQL成本分析,最后通过 select 查询总结整个查询过程,下面一起来看一下,希望对大家有帮助。
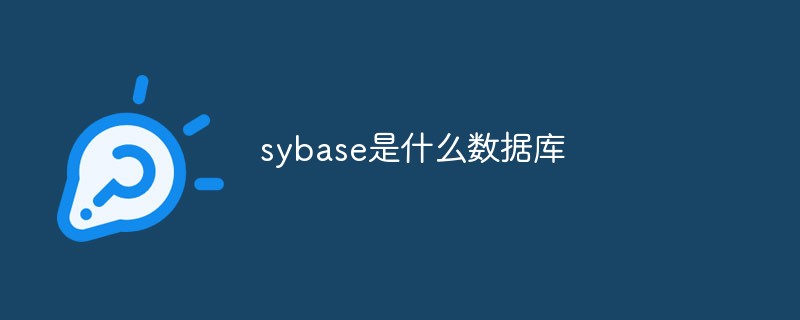
sybase是基于客户/服务器体系结构的数据库,是一个开放的、高性能的、可编程的数据库,可使用事件驱动的触发器、多线索化等来提高性能。
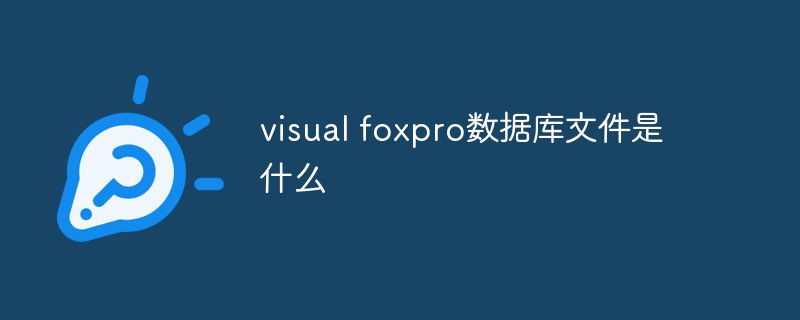
visual foxpro数据库文件是管理数据库对象的系统文件。在VFP中,用户数据是存放在“.DBF”表文件中;VFP的数据库文件(“.DBC”)中不存放用户数据,它只起将属于某一数据库的 数据库表与视图、连接、存储过程等关联起来的作用。
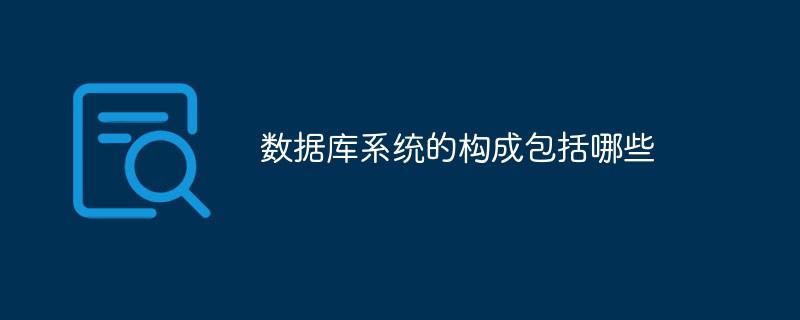
数据库系统由4个部分构成:1、数据库,是指长期存储在计算机内的,有组织,可共享的数据的集合;2、硬件,是指构成计算机系统的各种物理设备,包括存储所需的外部设备;3、软件,包括操作系统、数据库管理系统及应用程序;4、人员,包括系统分析员和数据库设计人员、应用程序员(负责编写使用数据库的应用程序)、最终用户(利用接口或查询语言访问数据库)、数据库管理员(负责数据库的总体信息控制)。
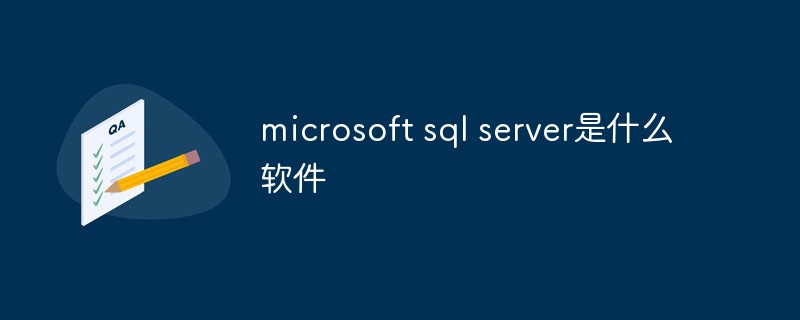
microsoft sql server是Microsoft公司推出的关系型数据库管理系统,是一个全面的数据库平台,使用集成的商业智能(BI)工具提供了企业级的数据管理,具有使用方便可伸缩性好与相关软件集成程度高等优点。SQL Server数据库引擎为关系型数据和结构化数据提供了更安全可靠的存储功能,使用户可以构建和管理用于业务的高可用和高性能的数据应用程序。
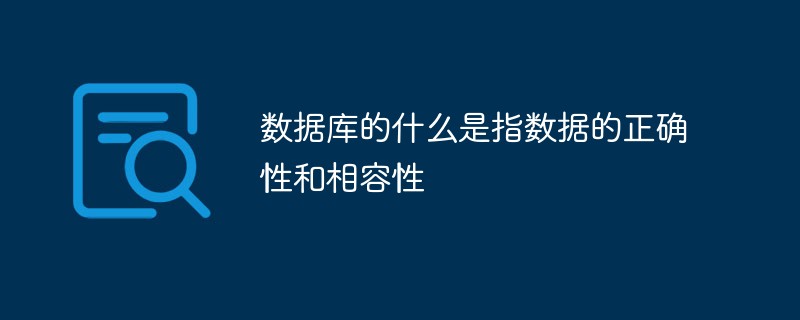
数据库的“完整性”是指数据的正确性和相容性。完整性是指数据库中数据在逻辑上的一致性、正确性、有效性和相容性。完整性对于数据库系统的重要性:1、数据库完整性约束能够防止合法用户使用数据库时向数据库中添加不合语义的数据;2、合理的数据库完整性设计,能够同时兼顾数据库的完整性和系统的效能;3、完善的数据库完整性有助于尽早发现应用软件的错误。
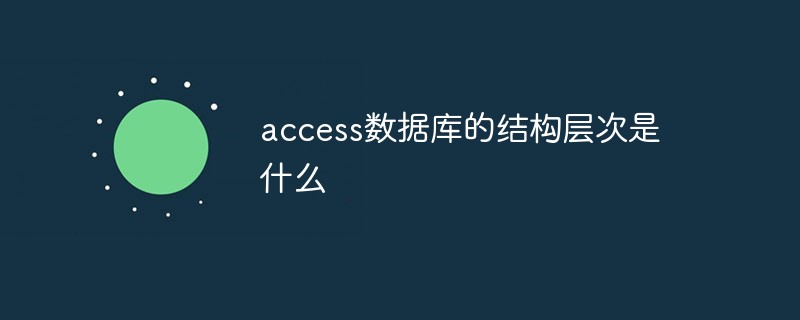
结构层次是“数据库→数据表→记录→字段”;字段构成记录,记录构成数据表,数据表构成了数据库。数据库是一个完整的数据的记录的整体,一个数据库包含0到N个表,一个表包含0到N个字段,记录是表中的行。
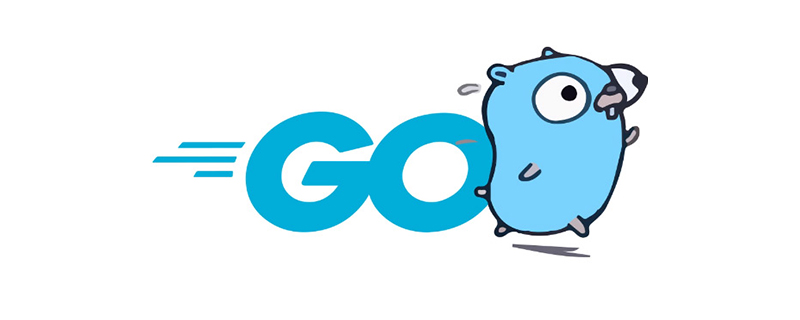
go语言可以写数据库。Go语言和其他语言不同的地方是,Go官方没有提供数据库驱动,而是编写了开发数据库驱动的标准接口,开发者可以根据定义的接口来开发相应的数据库驱动;这样做的好处在于,只要是按照标准接口开发的代码,以后迁移数据库时,不需要做任何修改,极大方便了后期的架构调整。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Linux new version
SublimeText3 Linux latest version
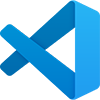
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
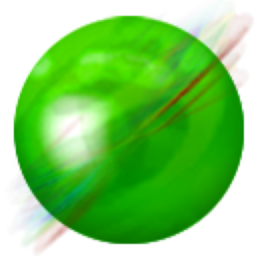
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
