How to use loose coupling in object-oriented programming?
Answer: Loose coupling is an OOP principle that reduces dependencies between classes and improves the maintainability and scalability of the code. Advantages: Flexibility: Modify and replace classes easily. Reusability: Reduce class dependencies and improve reusability. Testability: Reduce interaction and facilitate single class testing. Implementation method: use abstract interfaces, define methods, and implementation classes provide concrete implementations. Use dependency injection instead of creating instances of dependencies internally. Case: Shopping cart application, the Cart class relies on the Product interface to track products, achieving loose coupling and can easily replace different Product implementations.
The application of loose coupling in object-oriented programming
Loose coupling is an important object-oriented programming (OOP) principle. Dependencies between classes are reduced, making the code easier to maintain and extend.
Advantages of loose coupling
- Flexibility: Loose coupling allows classes to be easily modified or replaced without affecting other classes .
- Reusability: Loosely coupled classes can be reused more easily because they have fewer dependencies on other classes.
- Testability: Loose coupling makes it easier to test individual classes because they interact less with other classes.
How to use loose coupling
The key to achieving loose coupling is to use abstract interfaces and dependency injection.
Abstract interface
Abstract interface defines a set of methods, and the classes that implement these methods provide concrete implementations. This allows client code to depend on interfaces rather than concrete classes, thus achieving loose coupling.
Dependency Injection
Dependency injection is a way of creating objects in which instances of the object's dependencies are provided outside the code instead of being created inside the object. This helps loose coupling because the object is not dependent on the specific way in which the instances it depends on were created.
Practical Case
Consider a shopping cart application where the Cart
class is responsible for tracking the items in the user's shopping cart. To use loose coupling, we can create a Product
interface to represent the items in the shopping cart, and a Cart
class that depends on the Product
interface:
// Product 接口 public interface Product { String getName(); double getPrice(); } // Cart 类 public class Cart { private List<Product> products = new ArrayList<>(); public void addProduct(Product product) { products.add(product); } public double getTotalPrice() { double total = 0; for (Product product : products) { total += product.getPrice(); } return total; } }
In this example, the Cart
class relies on the Product
interface rather than any specific Product
implementation. This allows us to easily replace different Product
implementations without affecting the behavior of the Cart
class.
The above is the detailed content of How to use loose coupling in object-oriented programming?. For more information, please follow other related articles on the PHP Chinese website!
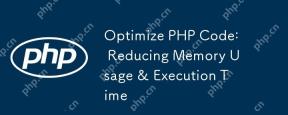
TooptimizePHPcodeforreducedmemoryusageandexecutiontime,followthesesteps:1)Usereferencesinsteadofcopyinglargedatastructurestoreducememoryconsumption.2)LeveragePHP'sbuilt-infunctionslikearray_mapforfasterexecution.3)Implementcachingmechanisms,suchasAPC
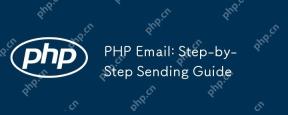
PHPisusedforsendingemailsduetoitsintegrationwithservermailservicesandexternalSMTPproviders,automatingnotificationsandmarketingcampaigns.1)SetupyourPHPenvironmentwithawebserverandPHP,ensuringthemailfunctionisenabled.2)UseabasicscriptwithPHP'smailfunct
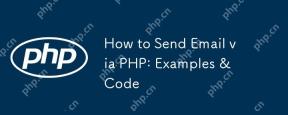
The best way to send emails is to use the PHPMailer library. 1) Using the mail() function is simple but unreliable, which may cause emails to enter spam or cannot be delivered. 2) PHPMailer provides better control and reliability, and supports HTML mail, attachments and SMTP authentication. 3) Make sure SMTP settings are configured correctly and encryption (such as STARTTLS or SSL/TLS) is used to enhance security. 4) For large amounts of emails, consider using a mail queue system to optimize performance.
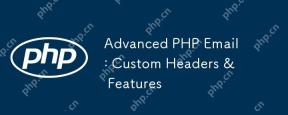
CustomheadersandadvancedfeaturesinPHPemailenhancefunctionalityandreliability.1)Customheadersaddmetadatafortrackingandcategorization.2)HTMLemailsallowformattingandinteractivity.3)AttachmentscanbesentusinglibrarieslikePHPMailer.4)SMTPauthenticationimpr
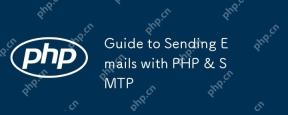
Sending mail using PHP and SMTP can be achieved through the PHPMailer library. 1) Install and configure PHPMailer, 2) Set SMTP server details, 3) Define the email content, 4) Send emails and handle errors. Use this method to ensure the reliability and security of emails.
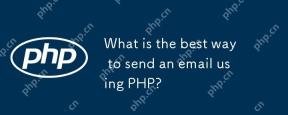
ThebestapproachforsendingemailsinPHPisusingthePHPMailerlibraryduetoitsreliability,featurerichness,andeaseofuse.PHPMailersupportsSMTP,providesdetailederrorhandling,allowssendingHTMLandplaintextemails,supportsattachments,andenhancessecurity.Foroptimalu
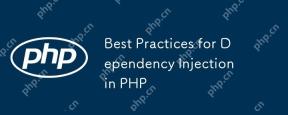
The reason for using Dependency Injection (DI) is that it promotes loose coupling, testability, and maintainability of the code. 1) Use constructor to inject dependencies, 2) Avoid using service locators, 3) Use dependency injection containers to manage dependencies, 4) Improve testability through injecting dependencies, 5) Avoid over-injection dependencies, 6) Consider the impact of DI on performance.
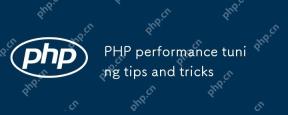
PHPperformancetuningiscrucialbecauseitenhancesspeedandefficiency,whicharevitalforwebapplications.1)CachingwithAPCureducesdatabaseloadandimprovesresponsetimes.2)Optimizingdatabasequeriesbyselectingnecessarycolumnsandusingindexingspeedsupdataretrieval.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 Chinese version
Chinese version, very easy to use

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
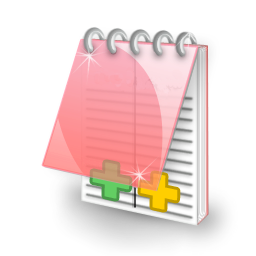
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
