


Big data processing in C++ technology: How to design optimized data structures to process large data sets?
Big data processing is optimized using data structures in C, including: Array: Used to store elements of the same type, and dynamic arrays can be resized as needed. Hash table: Used for fast lookup and insertion of key-value pairs, even if the data set is large. Binary tree: Used to quickly find, insert and delete elements, such as a binary search tree. Graph data structure: Used to represent connection relationships. For example, an undirected graph can store the relationship between nodes and edges. Optimization considerations: Includes parallel processing, data partitioning, and caching to improve performance.
Big Data Processing in C Technology: Designing Optimized Data Structures
Introduction
Big data processing is a common challenge in C, requiring the use of carefully designed algorithms and data structures to effectively manage and manipulate huge data sets. This article will introduce some optimized big data data structures and practical use cases.
Array
Array is a simple and efficient data structure that stores elements of the same data type. When dealing with big data, you can use dynamic arrays (such as std::vector
) to dynamically increase or decrease their size to meet changing needs.
Example:
std::vector<int> numbers; // 添加元素 numbers.push_back(10); numbers.push_back(20); // 访问元素 for (const auto& num : numbers) { std::cout << num << " "; }
Hash table
A hash table is a method used to quickly find and insert elements. Key-value pair data structure. When dealing with big data, hash tables (such as std::unordered_map
) can efficiently find data based on key values, even if the data set is very large.
Example:
std::unordered_map<std::string, int> word_counts; // 插入元素 word_counts["hello"]++; // 查找元素 auto count = word_counts.find("hello");
Binary tree
A binary tree is a tree data structure in which each node has at most two child node. Binary search trees (such as std::set
) allow fast finding, insertion, and deletion of elements, even if the data set is large.
Example:
std::set<int> numbers; // 插入元素 numbers.insert(10); numbers.insert(20); // 查找元素 auto found = numbers.find(10);
Graph data structure
The graph data structure is a non-linear data structure in which the elements are Represented in the form of nodes and edges. When processing big data, graph data structures (such as std::unordered_map<int std::vector>></int>
) can be used to represent complex connection relationships.
Example:
std::unordered_map<int, std::vector<int>> graph; // 添加边 graph[1].push_back(2); graph[1].push_back(3); // 遍历图 for (const auto& [node, neighbors] : graph) { std::cout << node << ": "; for (const auto& neighbor : neighbors) { std::cout << neighbor << " "; } std::cout << std::endl; }
Other optimization considerations
In addition to choosing the right data structure, you can also use the following Ways to further optimize big data processing:
- Parallel processing: Use multi-threads or multi-processors to process data in parallel.
- Data Partitioning: Divide large data sets into smaller chunks so that multiple chunks can be processed simultaneously.
- Cache: Store frequently accessed data in fast-access memory to reduce the latency of read/write operations.
The above is the detailed content of Big data processing in C++ technology: How to design optimized data structures to process large data sets?. For more information, please follow other related articles on the PHP Chinese website!
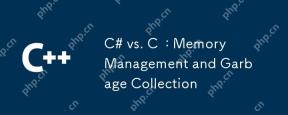
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
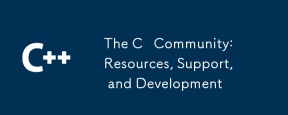
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
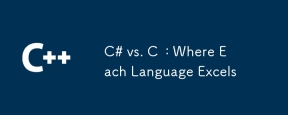
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.
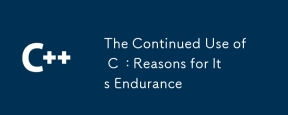
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
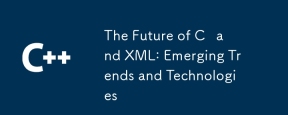
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
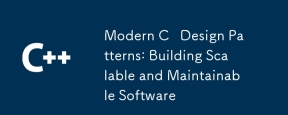
The modern C design model uses new features of C 11 and beyond to help build more flexible and efficient software. 1) Use lambda expressions and std::function to simplify observer pattern. 2) Optimize performance through mobile semantics and perfect forwarding. 3) Intelligent pointers ensure type safety and resource management.
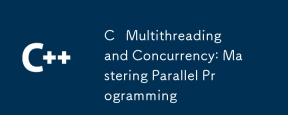
C The core concepts of multithreading and concurrent programming include thread creation and management, synchronization and mutual exclusion, conditional variables, thread pooling, asynchronous programming, common errors and debugging techniques, and performance optimization and best practices. 1) Create threads using the std::thread class. The example shows how to create and wait for the thread to complete. 2) Synchronize and mutual exclusion to use std::mutex and std::lock_guard to protect shared resources and avoid data competition. 3) Condition variables realize communication and synchronization between threads through std::condition_variable. 4) The thread pool example shows how to use the ThreadPool class to process tasks in parallel to improve efficiency. 5) Asynchronous programming uses std::as


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
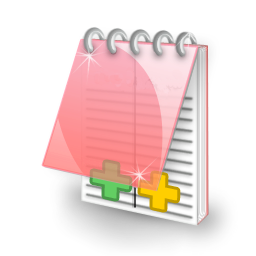
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
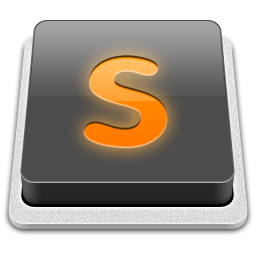
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.