


Exception handling to enhance robustness in C concurrent programming involves the following strategy: Use thread-local storage (TLS) to store exception information. Use mutexes to prevent concurrent access to shared data. Through these strategies, exceptions that occur in different threads can be effectively handled to ensure that the application remains stable under unexpected errors.
Enhance robustness through exception handling in C concurrent programming
Concurrent programming involves multiple threads executing in parallel and requires careful attention Exception handling to ensure program robustness. Exceptions can occur in any thread and, if not handled correctly, can cause data corruption, deadlock, or program crash.
Understanding exceptions in C
C exceptions are passed through the keywords try
, catch
and throw
accomplish. try
blocks contain code that may throw exceptions, while catch
blocks are used to handle specific types of exceptions. The throw
statement is used to throw exceptions.
Handling Exceptions in Parallel Threads
In concurrent programming, exception handling becomes more complex because exceptions can occur in any thread. To deal with this problem, the following strategy needs to be adopted:
- Use Thread Local Storage (TLS): Each thread maintains its own TLS area where exception information can be stored. When an exception occurs, the exception information is stored in TLS so that it can be easily accessed when needed.
- Using mutexes: Mutexes are used to synchronize access to shared resources. When handling concurrent exceptions, you can use mutexes to prevent exception handlers from different threads from accessing shared data at the same time.
Practical Case
Consider the following C code example, which uses a thread pool to handle tasks in multiple threads:
#include <thread> #include <vector> #include <mutex> std::mutex m; std::vector<std::thread> threads; void task(int id) { try { // ... 执行任务 } catch (std::exception& e) { std::lock_guard<std::mutex> lock(m); std::cout << "Exception in thread " << id << ": " << e.what() << std::endl; } } int main() { for (int i = 0; i < 10; i++) { threads.emplace_back(task, i); } for (auto& thread : threads) { thread.join(); } return 0; }
In the example Medium:
-
task()
The function is a routine that performs tasks in a child thread and handles exceptions. -
m
is a mutex used to protect access to shared console output. The -
try-catch
block handles the exception in thetask()
function and outputs the error message to the console.
Conclusion
Exception handling in C concurrent programming can significantly enhance the robustness of the program by adopting strategies such as thread-local storage and mutexes. By carefully handling exceptions that may occur, you can ensure that your application continues to run smoothly when unexpected errors occur.
The above is the detailed content of How does exception handling enhance robustness in C++ concurrent programming?. For more information, please follow other related articles on the PHP Chinese website!
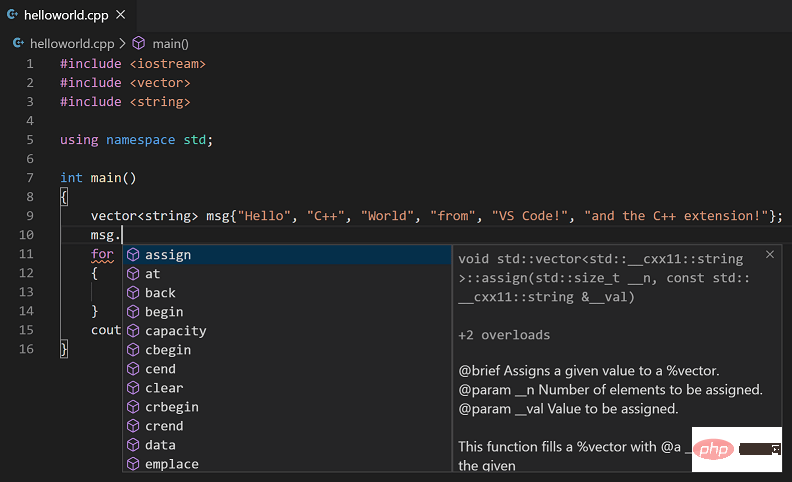
C++是一种广泛使用的面向对象的计算机编程语言,它支持您与之交互的大多数应用程序和网站。你需要编译器和集成开发环境来开发C++应用程序,既然你在这里,我猜你正在寻找一个。我们将在本文中介绍一些适用于Windows11的C++编译器的主要推荐。许多审查的编译器将主要用于C++,但也有许多通用编译器您可能想尝试。MinGW可以在Windows11上运行吗?在本文中,我们没有将MinGW作为独立编译器进行讨论,但如果讨论了某些IDE中的功能,并且是DevC++编译器的首选
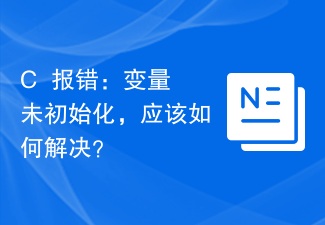
在C++程序开发中,当我们声明了一个变量但是没有对其进行初始化,就会出现“变量未初始化”的报错。这种报错经常会让人感到很困惑和无从下手,因为这种错误并不像其他常见的语法错误那样具体,也不会给出特定的代码行数或者错误类型。因此,下面我们将详细介绍变量未初始化的问题,以及如何解决这个报错。一、什么是变量未初始化错误?变量未初始化是指在程序中声明了一个变量但是没有
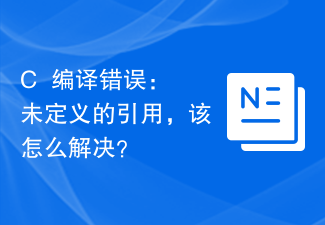
C++是一门广受欢迎的编程语言,但是在使用过程中,经常会出现“未定义的引用”这个编译错误,给程序的开发带来了诸多麻烦。本篇文章将从出错原因和解决方法两个方面,探讨“未定义的引用”错误的解决方法。一、出错原因C++编译器在编译一个源文件时,会将它分为两个阶段:编译阶段和链接阶段。编译阶段将源文件中的源码转换为汇编代码,而链接阶段将不同的源文件合并为一个可执行文
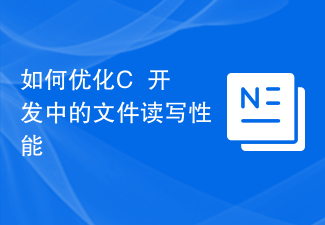
如何优化C++开发中的文件读写性能在C++开发过程中,文件的读写操作是常见的任务之一。然而,由于文件读写是磁盘IO操作,相对于内存IO操作来说会更为耗时。为了提高程序的性能,我们需要优化文件读写操作。本文将介绍一些常见的优化技巧和建议,帮助开发者在C++文件读写过程中提高性能。使用合适的文件读写方式在C++中,文件读写可以通过多种方式实现,如C风格的文件IO
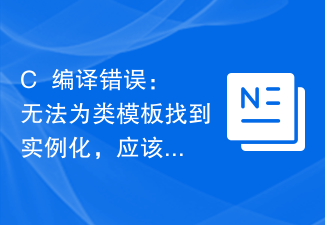
C++是一门强大的编程语言,它支持使用类模板来实现代码的复用,提高开发效率。但是在使用类模板时,可能会遭遇编译错误,其中一个比较常见的错误是“无法为类模板找到实例化”(error:cannotfindinstantiationofclasstemplate)。本文将介绍这个问题的原因以及如何解决。问题描述在使用类模板时,有时会遇到以下错误信息:e
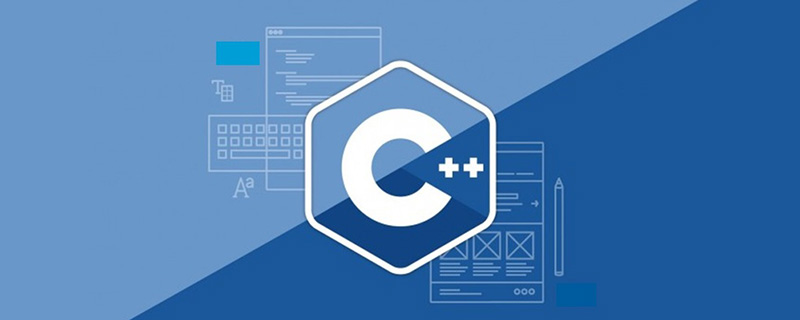
iostream头文件包含了操作输入输出流的方法,比如读取一个文件,以流的方式读取;其作用是:让初学者有一个方便的命令行输入输出试验环境。iostream的设计初衷是提供一个可扩展的类型安全的IO机制。
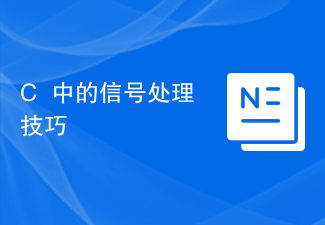
C++是一种流行的编程语言,它强大而灵活,适用于各种应用程序开发。在使用C++开发应用程序时,经常需要处理各种信号。本文将介绍C++中的信号处理技巧,以帮助开发人员更好地掌握这一方面。一、信号处理的基本概念信号是一种软件中断,用于通知应用程序内部或外部事件。当特定事件发生时,操作系统会向应用程序发送信号,应用程序可以选择忽略或响应此信号。在C++中,信号可以
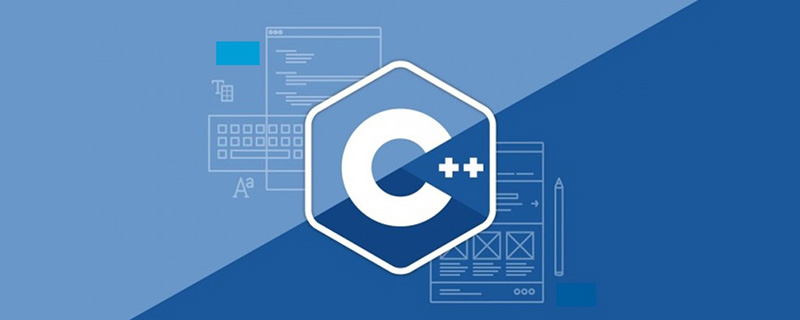
c++初始化数组的方法:1、先定义数组再给数组赋值,语法“数据类型 数组名[length];数组名[下标]=值;”;2、定义数组时初始化数组,语法“数据类型 数组名[length]=[值列表]”。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
