


PHP exception handling: Use exception handling to improve code reliability
PHP exception handling is a mechanism for handling unexpected situations in your code. Exceptions can be caught and handled through try-catch blocks or set_exception_handler functions. PHP provides the Exception class as the base class for all exceptions and has standard exception classes such as ArithmeticError, DivisionByZeroError, etc. Handling exceptions increases the reliability and robustness of your application and improves the user experience by avoiding crashes and providing meaningful error messages.
PHP Exception Handling: Making the Code More Reliable
Introduction
Exception Handling is an effective way to handle unexpected situations in your code. By catching and handling exceptions, we can make our applications more reliable and robust.
Exception class
PHP provides the Exception
class as the base class for all exceptions, as well as several standard exception classes, such as:
-
ArithmeticError
: Arithmetic error (such as division by zero) -
DivisionByZeroError
: Division by zero -
TypeError
: Type error -
SyntaxError
: Syntax error
Exception handling syntax
There are two main methods Can handle exceptions:
1. try-catch block
try { // 可能会引发异常的代码 } catch (Exception $e) { // 处理异常 }
2. set_exception_handler
set_exception_handler(function (Exception $e) { // 处理异常 });
Actual case
Let's consider a simple PHP function to divide a number:
function divide($dividend, $divisor) { if ($divisor == 0) { // 手动引发 DivisionByZeroError 异常 throw new DivisionByZeroError("Division by zero"); } return $dividend / $divisor; }
We can use a try-catch block to catch and handle the DivisionByZeroError
exception:
try { $result = divide(10, 2); // 继续执行其他代码 } catch (DivisionByZeroError $e) { // 处理 DivisionByZeroError 异常并显示错误消息 echo "Error: Division by zero is not allowed."; }
Conclusion
Exception handling is a powerful feature in PHP that allows us to write more reliable and robust applications. By handling exceptions correctly, we can avoid application crashes and provide meaningful error messages, thereby improving user experience.
The above is the detailed content of PHP exception handling: Use exception handling to improve code reliability. For more information, please follow other related articles on the PHP Chinese website!
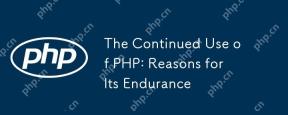
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
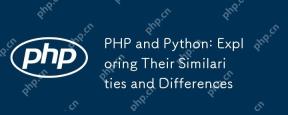
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
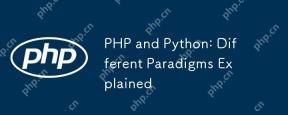
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
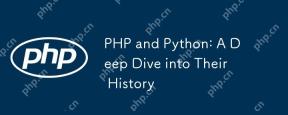
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
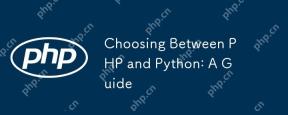
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
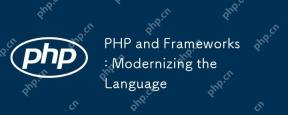
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
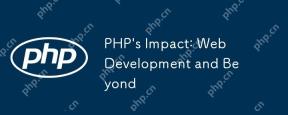
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
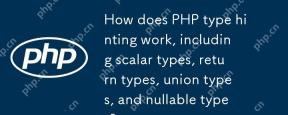
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
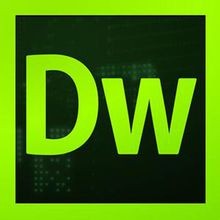
Dreamweaver CS6
Visual web development tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SublimeText3 Chinese version
Chinese version, very easy to use