Coroutine is a lightweight concurrent execution unit. Go language implements coroutine through goroutine. The implementation principle is to adopt the M:N model, and each thread has its own coroutine scheduler to manage the coroutine. The code example creates a coroutine as go func(){}; prints the number of current thread coroutines as runtime.NumGoroutine(); and gives up thread control as runtime.Gosched(). In practical cases, coroutines can be used to process requests or asynchronous tasks concurrently, but attention should be paid to concurrent access issues to shared resources and performance issues caused by excessive creation of coroutines.
Coroutine implementation in Golang function
Coroutine is a lightweight concurrent execution unit that is independent of threads. Can be executed simultaneously in the same thread. In the Go language, coroutines are implemented through goroutines.
Coroutine implementation principle
Go language coroutine is implemented using the M:N model, where M represents the number of operating system threads and N represents the number of coroutines. Each thread has its own coroutine scheduler, which is responsible for managing the coroutines on the thread. When a coroutine blocks, the scheduler removes it from the current thread and continues executing other coroutines in another thread.
Code Example
package main import ( "fmt" "runtime" ) func main() { // 创建一个协程 go func() { fmt.Println("Hello from goroutine") }() // 打印当前线程中执行的协程数量 fmt.Println("Number of goroutines:", runtime.NumGoroutine()) // 等待协程执行完成 runtime.Gosched() }
In the code example, the go
statement creates a coroutine. runtime.NumGoroutine()
The function is used to print the number of coroutines executed in the current thread. runtime.Gosched()
The function gives up control of the current thread and allows other coroutines to execute.
Practical Case
In practical applications, coroutines can be used to concurrently process a large number of requests, asynchronous tasks or parallel calculations. For example, in a web service, you can create a coroutine for each request to process the requests in parallel.
It should be noted that:
- Coroutines are different from threads. They share the same memory space, so you need to pay attention to concurrent access to shared resources.
- Creating too many coroutines may cause performance problems because each coroutine requires certain memory and CPU resources.
The above is the detailed content of How are coroutines implemented in golang functions?. For more information, please follow other related articles on the PHP Chinese website!
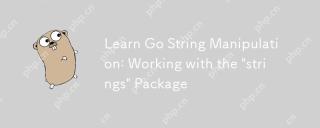
Go's "strings" package provides rich features to make string operation efficient and simple. 1) Use strings.Contains() to check substrings. 2) strings.Split() can be used to parse data, but it should be used with caution to avoid performance problems. 3) strings.Join() is suitable for formatting strings, but for small datasets, looping = is more efficient. 4) For large strings, it is more efficient to build strings using strings.Builder.
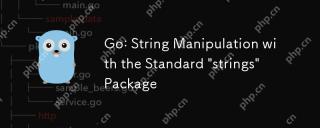
Go uses the "strings" package for string operations. 1) Use strings.Join function to splice strings. 2) Use the strings.Contains function to find substrings. 3) Use the strings.Replace function to replace strings. These functions are efficient and easy to use and are suitable for various string processing tasks.
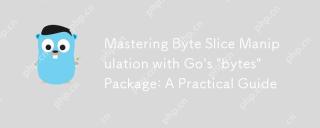
ThebytespackageinGoisessentialforefficientbyteslicemanipulation,offeringfunctionslikeContains,Index,andReplaceforsearchingandmodifyingbinarydata.Itenhancesperformanceandcodereadability,makingitavitaltoolforhandlingbinarydata,networkprotocols,andfileI
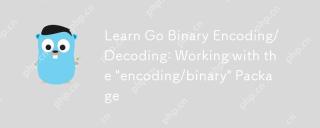
Go uses the "encoding/binary" package for binary encoding and decoding. 1) This package provides binary.Write and binary.Read functions for writing and reading data. 2) Pay attention to choosing the correct endian (such as BigEndian or LittleEndian). 3) Data alignment and error handling are also key to ensure the correctness and performance of the data.
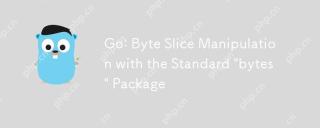
The"bytes"packageinGooffersefficientfunctionsformanipulatingbyteslices.1)Usebytes.Joinforconcatenatingslices,2)bytes.Bufferforincrementalwriting,3)bytes.Indexorbytes.IndexByteforsearching,4)bytes.Readerforreadinginchunks,and5)bytes.SplitNor
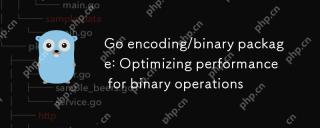
Theencoding/binarypackageinGoiseffectiveforoptimizingbinaryoperationsduetoitssupportforendiannessandefficientdatahandling.Toenhanceperformance:1)Usebinary.NativeEndianfornativeendiannesstoavoidbyteswapping.2)BatchReadandWriteoperationstoreduceI/Oover
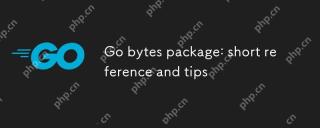
Go's bytes package is mainly used to efficiently process byte slices. 1) Using bytes.Buffer can efficiently perform string splicing to avoid unnecessary memory allocation. 2) The bytes.Equal function is used to quickly compare byte slices. 3) The bytes.Index, bytes.Split and bytes.ReplaceAll functions can be used to search and manipulate byte slices, but performance issues need to be paid attention to.
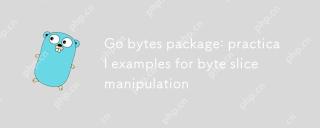
The byte package provides a variety of functions to efficiently process byte slices. 1) Use bytes.Contains to check the byte sequence. 2) Use bytes.Split to split byte slices. 3) Replace the byte sequence bytes.Replace. 4) Use bytes.Join to connect multiple byte slices. 5) Use bytes.Buffer to build data. 6) Combined bytes.Map for error processing and data verification.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
