1.null and undefined
* Obtain a property from an object. If neither the object nor the objects in its prototype chain have the property, the value of the property will be undefined.
* If a function does not explicitly return a value to its caller through return, its return value is undefined. There is a special case when using new.
* A function in JavaScript can declare any number of formal parameters. When the function is actually called, if the number of parameters passed in is less than the declared formal parameters, the value of the extra formal parameters will be undefined.
* null is an empty object, pay attention to the difference from empty objects ({}).
To put it simply, for all variables, as long as the initial value has not been specified after declaration, then it is undefined. If the Object type is used to express the concept of null reference, then it is represented by null.
2.if expression
* Null is always false (false)
* Undefined is always false (false)
* Number 0, -0 or NaN is false, other values are true
* String When the string is empty, it is false, and other values are true
* Object is always true (true)
3.Array
Arrays in JavaScript are very different from those in common programming languages, such as Java or C/C. An object in JavaScript is an unordered associative array, and Array is implemented by taking advantage of this feature of objects in JavaScript. In JavaScript, Array is actually an object, but its property names are integers. There are many additional properties (such as length) and methods (such as splice) to conveniently operate arrays.
4.new operator
JavaScript does not have the concept of a class in Java, but uses a constructor to create objects. New objects can be created using constructors in new expressions. Objects created by a constructor have an implicit reference to the constructor's prototype.
5.prototype
Prototype is the core concept of javascript prototypal inheritance. You must have seen the usage of Array.prototype.push.call() in a certain javascript class library, so in the final analysis, prototype is an object. We can add some useful methods to the native class through prototype, and we can also implement inheritance through prototype. If you are more interested in prototype, you can access the prototype chain of the specified object through __proto__ in ff.
6.scope chain
Execution context is an abstract concept used in the ECMAScript specification to describe the execution of JavaScript code. All JavaScript code runs within an execution context. When function is called in the current execution context, a new execution context will be entered. When the function call ends, it will return to the original execution context. If an exception is thrown during a function call and is not caught, it may exit from multiple execution contexts. During the function calling process, other functions may also be called to enter a new execution context. This forms an execution context stack.
Note: If you are more interested in the scope chain, you can access the function's scope chain through the __parent__ attribute in ff. Unfortunately, ff's js engine SpiderMonkey does not have perfect support for this attribute, and internal functions appear. An error will occur, so it is recommended to use Rhino (http://developer.mozilla.org/en/docs/Rhino).
Note: What needs to be mentioned here is the impact of function expressions and function declarations on the scope chain.
7.closure
Closure is also a commonly used feature of js. Generally, when we execute a function in java, all internal variables will be recycled. However, in javascript, we can use certain methods to make the executed function The internal variables still exist and are accessible, thus forming a closure.
Of course, everything has two sides. While closures bring us benefits, they can also cause a lot of troubles, such as memory leaks if we are not careful, so we need to apply them reasonably. these technologies. If you want to learn closures in depth, it is recommended to check out several js libraries (prototype, jquery, mootools), which contain some very classic closure applications, such as the bind method, etc., which I will not go into details here.
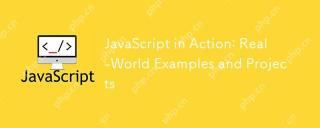
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
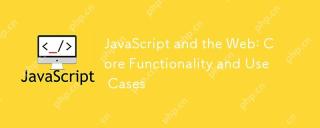
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
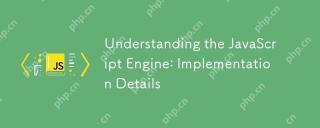
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
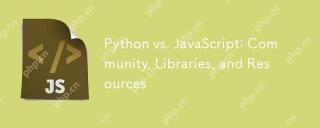
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
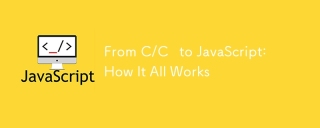
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
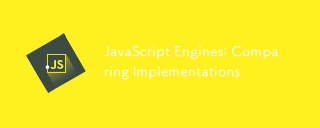
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
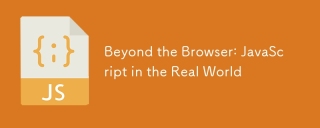
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
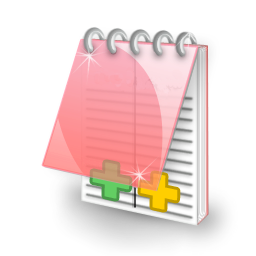
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 English version
Recommended: Win version, supports code prompts!

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool