Original text: Model-View-Controller (MVC) with JavaScript
Author: Alex@Net
Translation: MVC pattern of JavaScript
Translator: justjavac
This article introduces the implementation of the model-view-controller pattern in JavaScript.
I love JavaScript because it is one of the most flexible languages in the world. In JavaScript, programmers can choose the programming style according to their taste: procedural or object-oriented. If you are a heavy user, JavaScript can handle it with ease: process-oriented, object-oriented, aspect-oriented. Using JavaScript developers can even use functional programming techniques.
In this article, my goal is to write a simple JavaScript component to show everyone the power of JavaScript. This component is an editable list of items (select tag in HTML): the user can select an item and delete it, or add new items to the list. The component will be composed of three classes, corresponding to the model-view-controller of the MVC design pattern.
This article is just a simple guide. If you wish to use it in a real project, you will need to make appropriate modifications. I believe you have everything you need to create and run JavaScript programs: a brain, hands, a text editor (like Notepad), and a browser (like my favorite, Chrome).
Since our code will use the MVC pattern, I will briefly introduce this design pattern here. The English name of the MVC pattern is Model-View-Controller pattern. As the name suggests, its main parts are:
•Model(), used to store data used in the program;
•View, used to present data in different forms;
•Controller (Controller), updated the model.
In Wikipedia’s definition of MVC architecture, it consists of the following three parts:
Model - "Data model" (Model) is used to encapsulate data related to the business logic of the application and how to process the data. The "model" has direct access to the data. The "model" does not depend on the "view" and "controller", that is, the model does not care how it will be displayed or how it will be manipulated.
View - The view layer enables the purposeful display of data, usually a user interface element. There is generally no procedural logic in views. In MVC in web applications, the html page that displays dynamic data is usually called a view.
Controller (Controller) - handles and responds to events, usually user operations, and monitors changes on the model, and then modifies the view.
The data of the component is a list of items, in which one particular item can be selected and deleted. So, the model of the component is very simple - it is stored in an array property and selected item property; and here it is:
We will implement a data list component based on MVC, and items in the list can be selected and deleted. Therefore, the component model is very simple - it only requires two properties:
1. The array _items is used to store all elements
2. The ordinary variable _selectedIndex is used to store the selected element index
The code is as follows:
/**
* Model.
*
* The model stores all elements and notifies the Observer when the state changes.
*/
function ListModel(items) {
this._items = items; // 所有元素
this._selectedIndex = -1; // 被选择元素的索引
this.itemAdded = new Event(this);
this.itemRemoved = new Event(this);
this.selectedIndexChanged = new Event(this);
}
ListModel.prototype = {
getItems : function () {
return [].concat(this._items);
},
addItem : function (item) {
this._items.push(item);
this.itemAdded.notify({item : item});
},
removeItemAt : function (index) {
var item;
item = this._items[index];
this._items.splice(index, 1);
this.itemRemoved.notify({item : item});
if (index === this._selectedIndex) {
this.setSelectedIndex(-1);
}
},
getSelectedIndex : function () {
return this._selectedIndex;
},
setSelectedIndex : function (index) {
var previousIndex;
previousIndex = this._selectedIndex;
this._selectedIndex = index;
this.selectedIndexChanged.notify({previous : previousIndex});
}
};
Event 是一个简单的实现了观察者模式(Observer pattern)的类:
function Event(sender) {
this._sender = sender;
this._listeners = [];
}
Event.prototype = {
attach : function (listener) {
this._listeners.push(listener);
},
notify : function (args) {
var index;
for (index = 0; index this._listeners[index](this._sender, args);
}
}
};
View 类需要定义控制器类,以便与它交互。 虽然这个任务可以有许多不同的接口(interface),但我更喜欢最简单的。 我希望我的项目是在一个 ListBox 控件和它下面的两个按钮:“加号”按钮添加项目,“减”删除所选项目。 组件所提供的“选择”功能则需要 select 控件的原生功能的支持。
一个 View 类被绑定在一个 Controller 类上, 其中「…控制器处理用户输入事件,通常是通过一个已注册的回调函数」(wikipedia.org)。
下面是 View 和 Controller 类:
/**
* View.
*
* The view displays model data and triggers UI events.
* The controller is used to handle these user interaction events
*/
function ListView(model, elements) {
this._model = model;
this._elements = elements;
this.listModified = new Event(this);
this.addButtonClicked = new Event(this);
this.delButtonClicked = new Event(this);
var _this = this;
// 绑定模型监听器
this._model.itemAdded.attach(function () {
_this.rebuildList();
});
this._model.itemRemoved.attach(function () {
_this.rebuildList();
});
// 将监听器绑定到 HTML 控件上
this._elements.list.change(function (e) {
_this.listModified.notify({ index : e.target.selectedIndex });
});
this._elements.addButton.click(function () {
_this.addButtonClicked.notify();
});
this._elements.delButton.click(function () {
_this.delButtonClicked.notify();
});
}
ListView.prototype = {
show : function () {
this.rebuildList();
},
rebuildList : function () {
var list, items, key;
list = this._elements.list;
list.html('');
items = this._model.getItems();
for (key in items) {
if (items.hasOwnProperty(key)) {
list.append($(''));
}
}
this._model.setSelectedIndex(-1);
}
};
/**
* Controller.
*
* The controller responds to user operations and calls the change function on the model.
*/
function ListController(model, view) {
this._model = model;
this._view = view;
var _this = this;
this._view.listModified.attach(function (sender, args) {
_this.updateSelected(args.index);
});
this._view.addButtonClicked.attach(function () {
_this.addItem();
});
this._view.delButtonClicked.attach(function () {
_this.delItem();
});
}
ListController.prototype = {
addItem : function () {
var item = window.prompt('Add item:', '');
if (item) {
this._model.addItem(item);
}
},
delItem : function () {
var index;
index = this._model.getSelectedIndex();
if (index !== -1) {
this._model.removeItemAt(this._model.getSelectedIndex());
}
},
updateSelected : function (index) {
this._model.setSelectedIndex(index);
}
};
当然,Model, View, Controller 类应当被实例化。
下面是一个使用此 MVC 的完整代码:
$(function () {
var model = new ListModel(['PHP', 'JavaScript']),
view = new ListView(model, {
'list' : $('#list'),
'addButton' : $('#plusBtn'),
'delButton' : $('#minusBtn')
}),
controller = new ListController(model, view);
view.show();
});
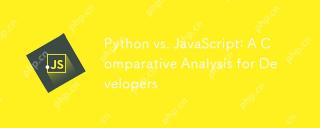
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
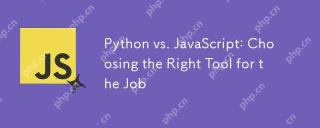
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
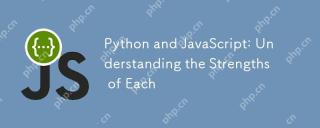
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
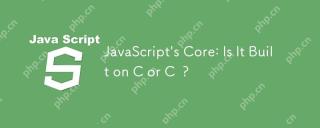
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
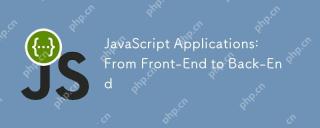
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
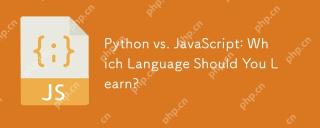
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
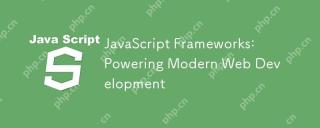
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
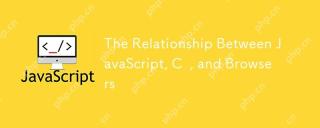
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
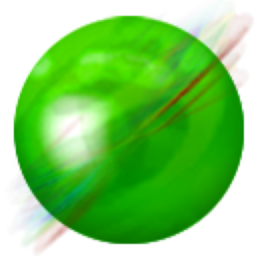
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
