


JS random colors are used in many places: for example, you can see that many tag connections are colorful. Then you need to get to this. Start below:
There are two methods in total. One is to prepare a set of beautiful candidate colors, and the other is to randomly generate colors .
Implementation 1
var getRandomColor = function(){
return '#'
(function(color){
return (color = '0123456789abcdef'[Math.floor(Math.random()*16)])
&& (color .length == 6) ? color: arguments.callee(color);
})('');
}
Randomly generate 6 characters and string them together. Closures calling themselves and the ternary operator make the program more introverted. Beginners should learn this writing method.
Implementation 2
var getRandomColor = function(){
return (function(m,s,c){
return (c ? arguments.callee(m,s,c-1) : '#')
s[m.floor(m.random() * 16)]
})(Math,'0123456789abcdef',5)
}
Extract the Math object, the string used to generate the hex color value, and use the Three parameters to determine whether to continue calling itself.
Achieve 3
The following is the quoted content:
Array.prototype.map = function(fn, thisObj) {
var scope = thisObj || window;
var a = [];
for ( var i=0, j=this.length; i a.push (fn.call(scope, this[i], i, this));
}
return a;
};
var getRandomColor = function(){
return '#' '0123456789abcdef'.split('').map(function(v,i,a){
return i>5 ? null : a[Math.floor(Math.random()*16)] }).join ('');
}
This requires us to do some expansion of the array. Map will return an array, and then we use join to string its elements into characters.
Implementation 4
The following is the quoted content:
var getRandomColor = function(){
return '#' Math.floor(Math.random()*16777215).toString(16);
}
This implementation is amazing, although it has a little bug. We know that the hex color value is from #000000 to #ffffff, and the following six digits are hexadecimal numbers, equivalent to "0x000000" to "0xffffff". The idea of this implementation is to convert the maximum value of hex ffffff to decimal first, perform randomization and then convert back to hexadecimal. Let's take a look at how to get the value 16777215.
The following is the quoted content:
Implement 5
The following is the quoted content:
var getRandomColor = function(){
return '#' (Math.random()*0xffffff}
Basically implement the improvement of 4, using the left shift operator to convert 0xffffff into an integer. This way you don’t have to remember 16777215. Since the precedence of the left shift operator is not as good as the multiplication sign, the left shift is done after randomization, and Math.floor is not even used.
Implementation 6
The following is the quoted content:
var getRandomColor = function(){
return '#' (function(h){
return new Array(7-h.length).join("0") h
})((Math.random()*0x1000000}
Fix the bug in the above version (cannot generate pure white and insufficient hex digits). 0x1000000 is equivalent to 0xffffff 1, ensuring that 0xffffff will be drawn. In the closure, we deal with the problem that the hex value is less than 5 digits and directly fill in the remaining digits with zeros.
Implementation 7
The following is the quoted content:
var getRandomColor = function(){
return '#' ('00000' (Math.random()*0x1000000}
This time, zeros are padded in front, and even recursion detection is omitted.
Let’s try it in practice:
以下は引用内容です:
初级饼图
&それ;p>初级23232饼图
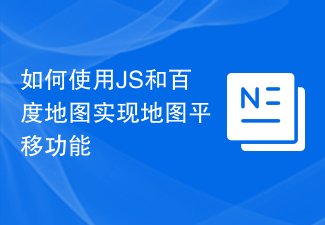
如何使用JS和百度地图实现地图平移功能百度地图是一款广泛使用的地图服务平台,在Web开发中经常用于展示地理信息、定位等功能。本文将介绍如何使用JS和百度地图API实现地图平移功能,并提供具体的代码示例。一、准备工作使用百度地图API前,首先需要在百度地图开放平台(http://lbsyun.baidu.com/)上申请一个开发者账号,并创建一个应用。创建完成
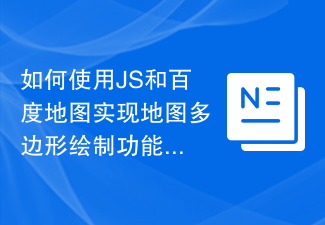
如何使用JS和百度地图实现地图多边形绘制功能在现代网页开发中,地图应用已经成为常见的功能之一。而地图上绘制多边形,可以帮助我们将特定区域进行标记,方便用户进行查看和分析。本文将介绍如何使用JS和百度地图API实现地图多边形绘制功能,并提供具体的代码示例。首先,我们需要引入百度地图API。可以利用以下代码在HTML文件中导入百度地图API的JavaScript
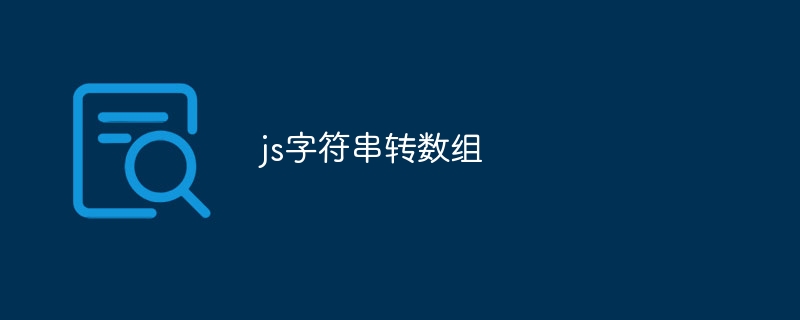
js字符串转数组的方法:1、使用“split()”方法,可以根据指定的分隔符将字符串分割成数组元素;2、使用“Array.from()”方法,可以将可迭代对象或类数组对象转换成真正的数组;3、使用for循环遍历,将每个字符依次添加到数组中;4、使用“Array.split()”方法,通过调用“Array.prototype.forEach()”将一个字符串拆分成数组的快捷方式。
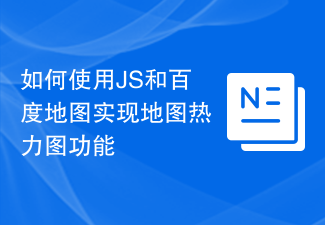
如何使用JS和百度地图实现地图热力图功能简介:随着互联网和移动设备的迅速发展,地图成为了一种普遍的应用场景。而热力图作为一种可视化的展示方式,能够帮助我们更直观地了解数据的分布情况。本文将介绍如何使用JS和百度地图API来实现地图热力图的功能,并提供具体的代码示例。准备工作:在开始之前,你需要准备以下事项:一个百度开发者账号,并创建一个应用,获取到相应的AP
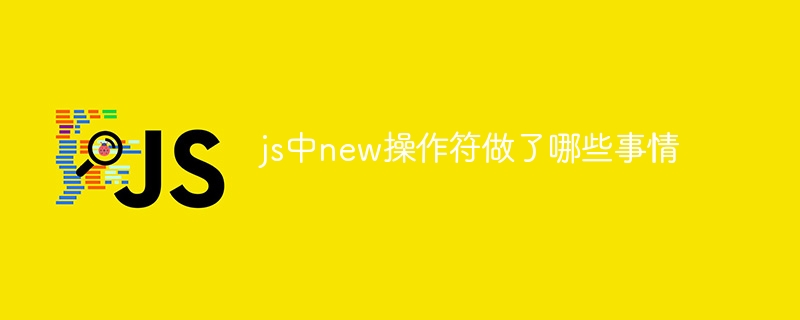
js中new操作符做了:1、创建一个空对象,这个新对象将成为函数的实例;2、将新对象的原型链接到构造函数的原型对象,这样新对象就可以访问构造函数原型对象中定义的属性和方法;3、将构造函数的作用域赋给新对象,这样新对象就可以通过this关键字来引用构造函数中的属性和方法;4、执行构造函数中的代码,构造函数中的代码将用于初始化新对象的属性和方法;5、如果构造函数中没有返回等等。
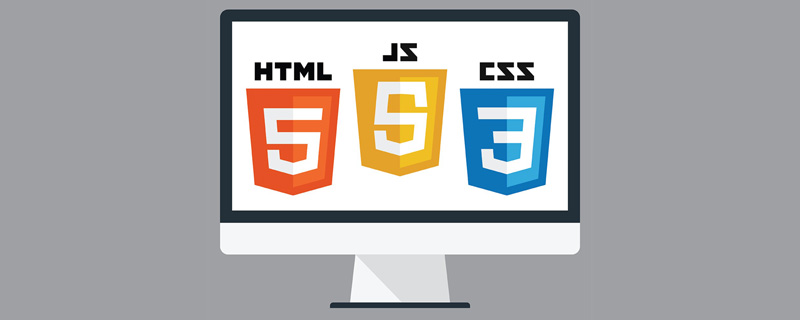
这篇文章主要为大家详细介绍了js实现打字小游戏,文中示例代码介绍的非常详细,具有一定的参考价值,感兴趣的小伙伴们可以参考一下。
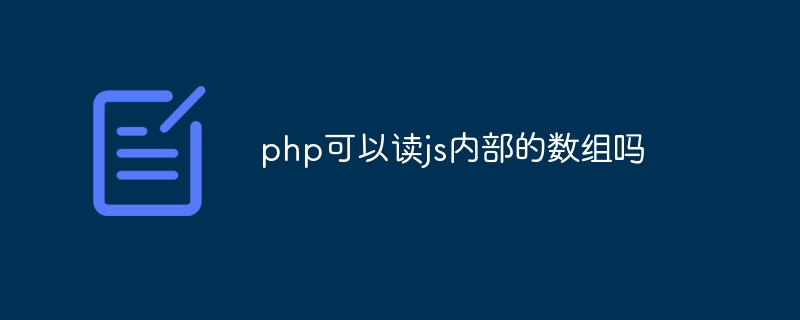
php在特定情况下可以读js内部的数组。其方法是:1、在JavaScript中,创建一个包含需要传递给PHP的数组的变量;2、使用Ajax技术将该数组发送给PHP脚本。可以使用原生的JavaScript代码或者使用基于Ajax的JavaScript库如jQuery等;3、在PHP脚本中,接收传递过来的数组数据,并进行相应的处理即可。
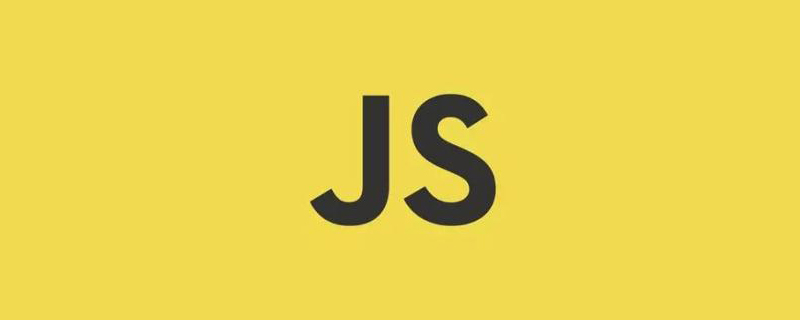
js全称JavaScript,是一种具有函数优先的轻量级,直译式、解释型或即时编译型的高级编程语言,是一种属于网络的高级脚本语言;JavaScript基于原型编程、多范式的动态脚本语言,并且支持面向对象、命令式和声明式,如函数式编程。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
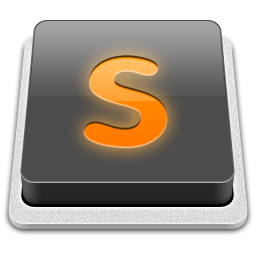
SublimeText3 Mac version
God-level code editing software (SublimeText3)

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Atom editor mac version download
The most popular open source editor
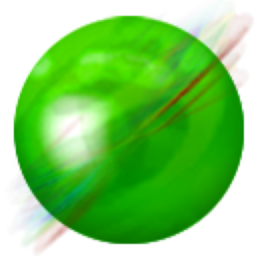
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
