


Ways of inheritance
ECMAScript has more than one way to implement inheritance. This is because the inheritance mechanism in JavaScript is not explicitly specified but implemented through imitation. This means that not all inheritance details are entirely handled by the interpreter. As the developer, you have the right to decide the inheritance method that works best for you. The most primitive inheritance implementation method is object impersonation. This method will be introduced below.
Object impersonation
The core of object impersonation to implement inheritance actually relies on using the this keyword in a function environment. The principle is as follows: the constructor uses the this keyword to assign values to all properties and methods (that is, using the constructor method of class declaration). Because a constructor is just a function, you can make the ClassA constructor a method of ClassB and then call it. ClassB will receive the properties and methods defined in the constructor of ClassA. For example, define ClassA and ClassB in the following way:
function ClassA(sColor) {
this.color = sColor;
this.sayColor = function () {
alert(this.color);
};
}
function ClassB(sColor) {
}
The keyword this refers to the object currently created by the constructor. But in this method, this points to the object it belongs to. The principle is to use ClassA as a regular function to establish the inheritance mechanism, rather than as a constructor. The inheritance mechanism can be implemented by using the constructor ClassB as follows:
function ClassB (sColor) {
this.newMethod = ClassA;
this.newMethod(sColor);
delete this.newMethod;
}
In this code, ClassA is assigned the method newMethod (remember, the function name is just a pointer to it). The method is then called, passing it the parameter sColor of the ClassB constructor. The last line of code removes the reference to ClassA so that it can no longer be called in the future.
All new properties and new methods must be defined after removing the line of code for the new method. Otherwise, the relevant properties and methods of the super class may be overridden:
function ClassB(sColor, sName) {
this.newMethod = ClassA;
this.newMethod(sColor);
delete this.newMethod;
this.name = sName;
this.sayName = function () {
alert(this.name);
};
}
To prove that the previous code is valid, you can run the following example:
var objA = new ClassA("blue");
var objB = new ClassB("red", "John");
objA.sayColor(); //Output "blue"
objB.sayColor(); //Output "red"
objB.sayName(); //Output "John"
Object impersonation can achieve multiple inheritance
Interestingly, object impersonation can support multiple inheritance. For example, if there are two classes ClassX and ClassY, and ClassZ wants to inherit these two classes, you can use the following code:
function ClassZ() {
this.newMethod = ClassX;
this.newMethod();
delete this.newMethod;
this.newMethod = ClassY;
this.newMethod();
delete this.newMethod;
}
There is a drawback here, if there are two classes ClassX For properties or methods with the same name as ClassY, ClassY has high priority. Because it inherits from the later class. Apart from this minor problem, it is easy to implement multiple inheritance mechanisms using object impersonation.
Due to the popularity of this inheritance method, the third version of ECMAScript added two methods to the Function object, namely call() and apply(). Later, many derived methods for implementing inheritance were actually implemented based on call() and apply().
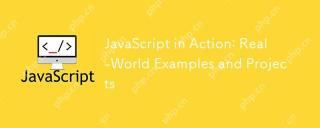
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
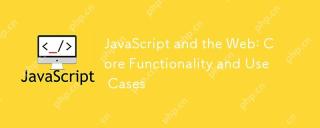
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
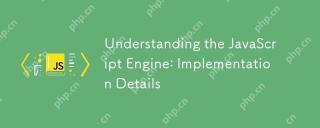
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
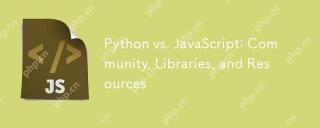
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
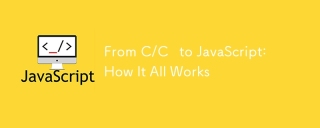
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
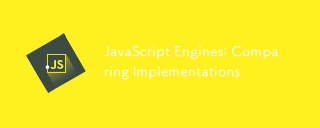
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
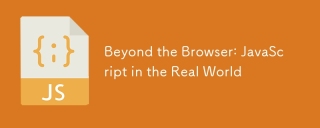
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
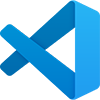
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
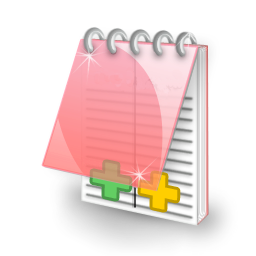
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Chinese version
Chinese version, very easy to use