


The core of JavaScript is ECMAScript. Similar to other languages, ECMAScript strings are immutable, that is, their values cannot be changed.
Consider the following code:
var str = "hello ";
str = "world";In fact, the steps performed by this code behind the scenes are as follows:
1. Create the character that stores "hello " string.
2. Create a string to store "world".
3. Create a string to store the connection result.
4. Copy the current content of str to the result.
5. Copy "world" into the result.
6. Update str so that it points to the result.
Steps 2 to 6 are performed every time string concatenation is completed, making this operation very resource intensive. If this process is repeated hundreds, or even thousands, of times, it can cause performance problems. The solution is to use an Array object to store the string and then use the join() method (parameter is an empty string) to create the final string. Imagine replacing the previous code with the following code:
var arr = new Array();
arr[0] = "hello ";
arr[1] = "world";
var str = arr.join("");
In this way, no matter how many strings are introduced into the array, it will not be a problem, because the join operation only occurs when the join() method is called. At this point, the steps to perform are as follows:
1. Create the strings to store the results
2. Copy each string to the appropriate location in the result
While this solution is good, there is a better way. The problem is, this code doesn't reflect exactly what it's intended to do. To make it easier to understand, you can wrap the functionality with the StringBuffer class:
function StringBuffer () {
this._strings_ = new Array();
}
StringBuffer.prototype.append = function(str) {
this._strings_.push(str);
};
StringBuffer.prototype.toString = function() {
return this._strings_.join("");
};
The first thing to pay attention to in this code is strings Attributes are meant to be private attributes. It has only two methods, namely append() and toString() methods. The append() method has a parameter, which appends the parameter to the string array. The toString() method calls the join method of the array and returns the actual concatenated string. To concatenate a set of strings using StringBuffer objects, you can use the following code:
var buffer = new StringBuffer ();
buffer.append("hello ");
buffer.append("world");
var result = buffer.toString();
Based on the above implementation, let’s compare the running time, that is, use " " to connect strings and our encapsulated tools one by one. The following code can be used to test the performance of StringBuffer objects and traditional string concatenation methods. Enter the code in the chrome console and run:
var d1 = new Date();
var str = "";
for (var i=0; i str = "text";
}
var d2 = new Date();
console.log("Concatenation with plus: "
(d2.getTime() - d1.getTime()) " milliseconds");
var buffer = new StringBuffer();
d1 = new Date();
for (var i=0; i buffer.append("text") ;
}
var result = buffer.toString();
d2 = new Date();
console.log("Concatenation with StringBuffer: "
(d2.getTime() - d1.getTime()) " milliseconds");
This code performs two tests for string concatenation, the first using the plus sign and the second using the StringBuffer class. Each operation concatenates 10,000 strings. The date values d1 and d2 are used to determine how long it takes to complete the operation. Please note that when creating a Date object without parameters, the current date and time are assigned to the object. To calculate how long the join operation took, subtract the millisecond representation of the date (using the return value of the getTime() method). This is a common way to measure JavaScript performance. The results of this test can help you compare the efficiency of using the StringBuffer class versus using the plus sign.
The results of the above example are as follows:
Then some people may say that the String object in JavaScript also encapsulates a concat() method. We will also use the concat() method below to do the same thing. Enter the following code in the consoel:
var d1 = new Date();
var str = "";
for (var i=0; i str.concat("text");
}
var d2 = new Date();
console.log("Concatenation with plus: "
(d2.getTime() - d1.getTime()) " milliseconds");
We can see that it is done 10000 times The time it takes to concatenate characters is:
It can be concluded that when it comes to a certain number of string connections, we can improve performance by encapsulating a StringBuffer object (function) similar to Java in Javascript to perform operations.
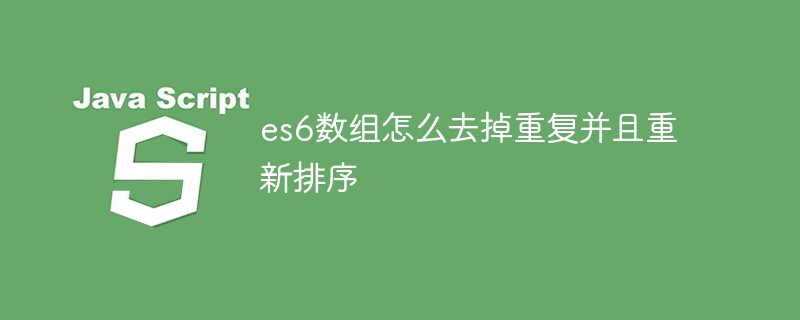
去掉重复并排序的方法:1、使用“Array.from(new Set(arr))”或者“[…new Set(arr)]”语句,去掉数组中的重复元素,返回去重后的新数组;2、利用sort()对去重数组进行排序,语法“去重数组.sort()”。
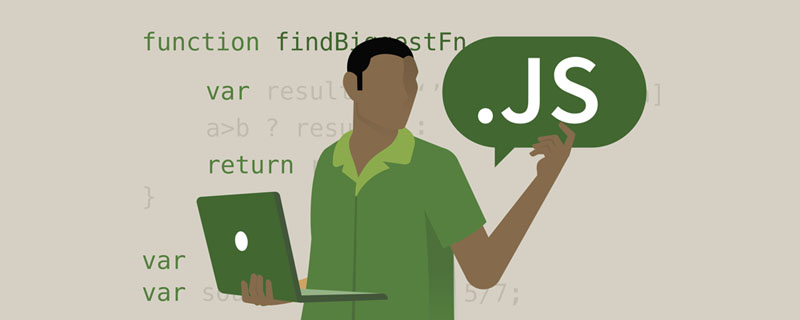
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于Symbol类型、隐藏属性及全局注册表的相关问题,包括了Symbol类型的描述、Symbol不会隐式转字符串等问题,下面一起来看一下,希望对大家有帮助。
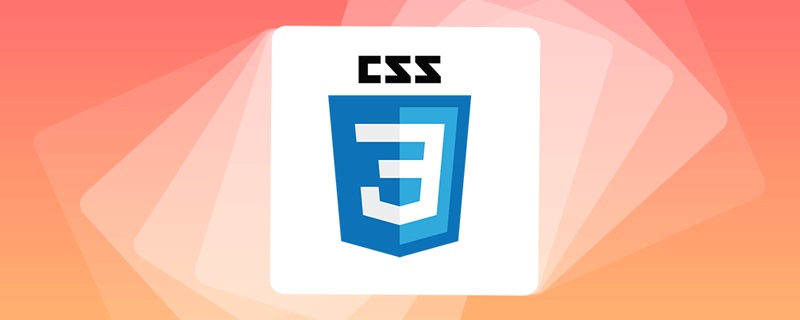
怎么制作文字轮播与图片轮播?大家第一想到的是不是利用js,其实利用纯CSS也能实现文字轮播与图片轮播,下面来看看实现方法,希望对大家有所帮助!
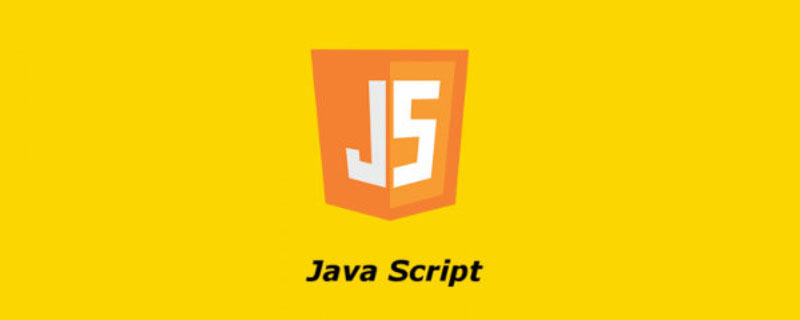
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于对象的构造函数和new操作符,构造函数是所有对象的成员方法中,最早被调用的那个,下面一起来看一下吧,希望对大家有帮助。
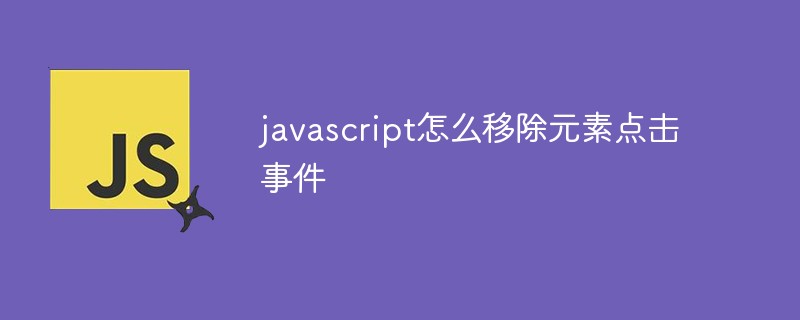
方法:1、利用“点击元素对象.unbind("click");”方法,该方法可以移除被选元素的事件处理程序;2、利用“点击元素对象.off("click");”方法,该方法可以移除通过on()方法添加的事件处理程序。
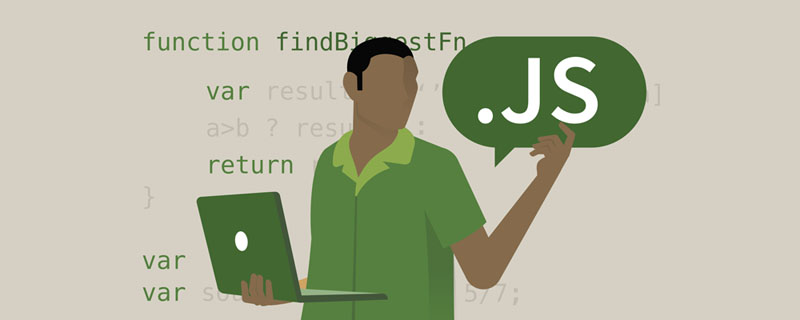
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于面向对象的相关问题,包括了属性描述符、数据描述符、存取描述符等等内容,下面一起来看一下,希望对大家有帮助。
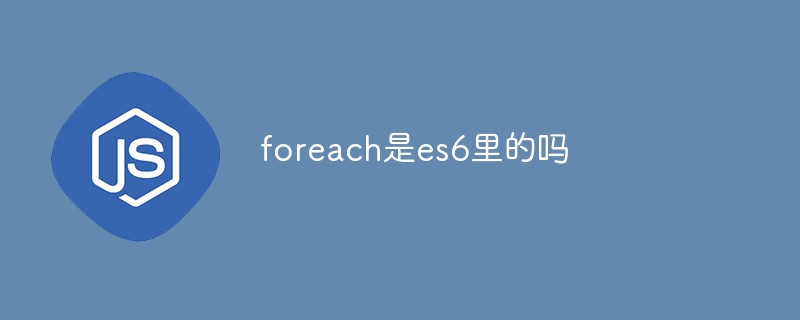
foreach不是es6的方法。foreach是es3中一个遍历数组的方法,可以调用数组的每个元素,并将元素传给回调函数进行处理,语法“array.forEach(function(当前元素,索引,数组){...})”;该方法不处理空数组。
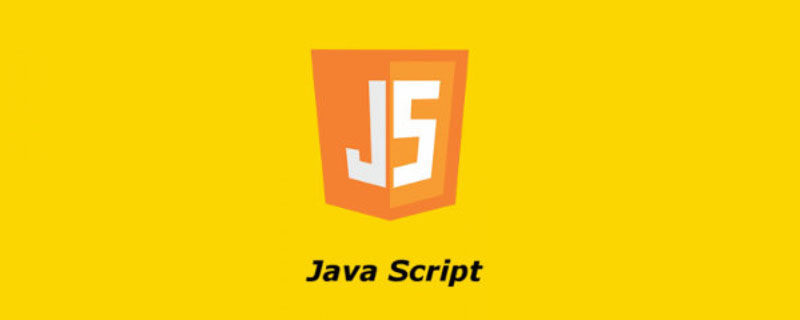
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于BOM操作的相关问题,包括了window对象的常见事件、JavaScript执行机制等等相关内容,下面一起来看一下,希望对大家有帮助。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
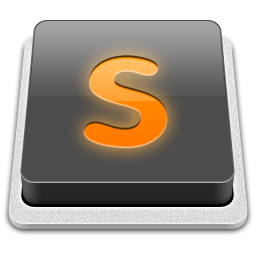
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
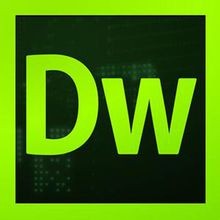
Dreamweaver CS6
Visual web development tools
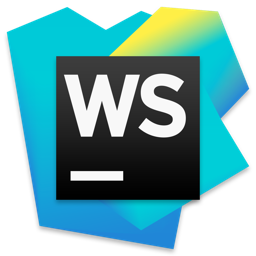
WebStorm Mac version
Useful JavaScript development tools