php面向对象的简单总结 $this $parent self
虽然接触php比较长时间,但有时在使用一些基础东西的时候还会有些不确定,有些疑惑。面向对象涉及到的比较多,大概总结整理一下php的属性、对象,以及访问方式$this $parent self 的使用场景。
1. PHP类属性定义和访问方式:
1
2 class testClass {
3 const tConst = 1;
4 public $tVar = 2; //或 public $tVar; 前面需要有变量修饰符
5 static $tSta = 3;
6
7 public function __construct(){
8 echo $this->tConst; //无错误,无输出
9 echo self::tConst; //正确输出 1
10
11 echo $this->tVar; // 注意tVar前不能有$符号
12 echo self::$tVar; // Access to undeclared static property: testClass::$tVar
13 echo self::tVar; // tVar前需要加上$符号,Undefined class constant 'tVar'
14
15 echo $this->tSta; //无错误,无输出
16 echo self::$tSta; //正确输出 3
17
18 }
19 }
20
21 $otestClass = new testClass();
总结几点:
在实例化对象时 $otestClass = new testClass(); 其中testClass()中的()可以省略,当构造函数有显式声明需要参数时,需要在这里传入参数
如果被引用的变量或者方法被声明成const(定义常量)或者static(声明静态),那么就必须使用操作符::
如果被引用的变量或者方法没有被声明成const或者static,那么就必须使用操作符->
从类的内部访问const或者static变量或者方法,使用自引用的self
从类的内部访问不为const或者static变量或者方法,使用自引用的$this
2. $this-> 究竟指向哪?
1 class testClass {
2 var $nick = '';
3
4 public function __construct($nick){
5 $this->nick = $nick;
6 }
7
8 public function display(){
9 echo $this->nick;
10 }
11 }
12
13 $otestClass1 = new testClass('frank');
14 $otestClass1->display(); //echo $otestClass1->nick; 和此结果相同
上面例子中,$this->display(); 其实就是 $otestClass1->nick,因此$this究竟指向哪是由所实例化的对象决定的,指向当前对象实例的指针。包括变量、方法都是如此
$this->方法() 的例子:
1 class baseClass{
2 public function testFunc(){
3 echo "\n" . 'I`m boss';
4 }
5 }
6
7 class parentClass extends baseClass{
8 public function testFunc(){
9 echo "\n" . 'I`m the up';
10 }
11 }
12
13 class testClass extends parentClass{
14 var $nick = '';
15
16 public function __construct($nick){
17 $this->nick = $nick;
18 }
19
20 public function display(){
21 echo $this->nick;
22 $this->testFunc();
23 }
24 }
25
26 $otestClass1 = new testClass('frank');
27 $otestClass1->display();
这样的代码最后的输出结果是什么呢?关键是看testFunc()方法。
如果在类中用$this调用一个当前类中不存在的方法或变量,它会依次去父类寻找,直到找不到再报错
基于第一条,如果找到了需要的方法或变量,就会停止寻找,如果其上级父类中还有同样的,则选择当前找到的
所以上面代码输出为:
frank
I`m the up
3. 关于parent::
parent是对父类的引用
1
2 class A {
3 public $a = 'aa';
4 public function callFunc(){
5 echo 'parent';
6 }
7 }
8
9 class B extends A{
10 public function __construct(){
11 parent::callFunc();
12 echo "\n" . $this->a;
13 }
14 }
15
16 $oB = new B;
比如,上面的代码中,在class B中,若要调用其父类A中的callFunc()方法,就需要使用parent::callFunc(),但A类中此方法必须是public修饰的,否则会提示 Fatal error: Call to private method A::callfunc() from context 'B'...
另外需要注意的是,对于父类中的属性$a,调用的时候用$this,用parent就访问不到了。若$a在A类中是这样定义的:public static $a,则访问的时候就需要parent::$a
注意,parent不具备传递性,它只能代表当前类的父类,若此例子A类继承base类,在base类中定义baseFunc()方法,那么在B类中使用parent::baseFunc()是错误的,只能在A类中这样使用。
4. self::又指向哪里?
简单的说,self和某具体实例没有关系,它只指向当前类,不会受某具体类对象的影响
1 class testClass{
2 public static $count = 0;
3 public $num = 0;
4
5 function __construct(){
6 self::$count++;
7 $this->num++;
8 }
9
10 function display(){
11 echo self::$count . "\n";
12 echo $this->num . "\n";
13 }
14 }
15
16 $oTest1 = new testClass;
17 $oTest1->display(); //输出: 1 1
18
19 $oTest2 = new testClass;
20 $oTest2->display(); //输出: 2 1
上面例子中self::$cout始终指向该类本身,而$num是单独存在于各个具体实例中的。
5. 总结:
$this 指向当前的实例
$parent 是父类的引用
self 对当前类本身的一个引用

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
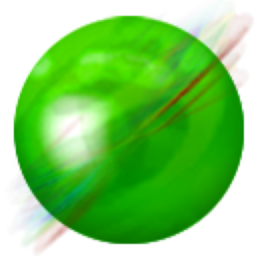
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Notepad++7.3.1
Easy-to-use and free code editor

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
