How jQuery parses Json string (Json format/Json object)_jquery
Json data is a commonly used small data exchange in real time. It can be parsed using jquery or js. Next, I will introduce the jquery method of parsing json strings.
1. jQuery parses Json data format:
Using this method, you must set parameters in the Ajax request:
1 dataType: "json"
Get through the callback function Return the data and parse it to get the value we want. See the source code:
jQuery.ajax({
url: full_url,
dataType: "json",
success: function(results) {
alert(result.name);
} }) ;
Normally, you can return JSON data from the background, and leave the frontend to jQuery, haha! !
Jquery asynchronous request sets the type (usually this configuration attribute) to "json", or uses the $.getJSON() method to obtain the server return, then there is no need
eval() method, because at this time you get The result is already a json object. You only need to call the object directly. Here, the $.getJSON method is used as an example.
Example 1
The code is as follows:
{
root:
[
{name :'1',value:'0'},
{name:'6101',value:'Beijing'},
{name:'6102',value:'Tianjin'},
{name:'6103',value:'Shanghai City'},
{name:'6104',value:'Chongqing City'},
{name:'6105',value:'Weinan City' },
{name:'6106',value:'Yan'an City'},
{name:'6107',value:'Hanzhong City'},
{name:'6108',value: 'Yulin City'},
{name:'6109',value:'Ankang City'},
{name:'6110',value:'Shangluo City'}
]
}" ;
//The data returned here is already a json object
//Others below The operation is the same as the first case
$.each(data.root,function(idx,item){
if(idx==0){
return true;//Same as countinue, return false the same as break
}
alert("name:" item.name ",value:" item.value);
});
});
2. jQuery parses Json objects: jQuery provides another method "parseJSON", which requires a standard JSON string and returns the generated JavaScript object. Let’s look at
and see the syntax:
data = $.parseJSON(string);
and see how it is used in actual development:
url: dataURL, success: function(results) {
var parsedJson = jQuery.parseJSON(results);
alert(parsedJson.name);
}
});
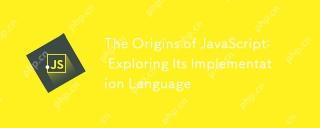
JavaScript originated in 1995 and was created by Brandon Ike, and realized the language into C. 1.C language provides high performance and system-level programming capabilities for JavaScript. 2. JavaScript's memory management and performance optimization rely on C language. 3. The cross-platform feature of C language helps JavaScript run efficiently on different operating systems.
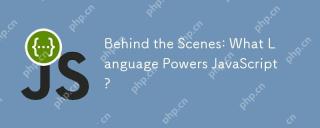
JavaScript runs in browsers and Node.js environments and relies on the JavaScript engine to parse and execute code. 1) Generate abstract syntax tree (AST) in the parsing stage; 2) convert AST into bytecode or machine code in the compilation stage; 3) execute the compiled code in the execution stage.
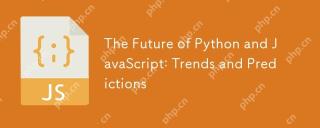
The future trends of Python and JavaScript include: 1. Python will consolidate its position in the fields of scientific computing and AI, 2. JavaScript will promote the development of web technology, 3. Cross-platform development will become a hot topic, and 4. Performance optimization will be the focus. Both will continue to expand application scenarios in their respective fields and make more breakthroughs in performance.
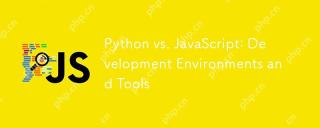
Both Python and JavaScript's choices in development environments are important. 1) Python's development environment includes PyCharm, JupyterNotebook and Anaconda, which are suitable for data science and rapid prototyping. 2) The development environment of JavaScript includes Node.js, VSCode and Webpack, which are suitable for front-end and back-end development. Choosing the right tools according to project needs can improve development efficiency and project success rate.
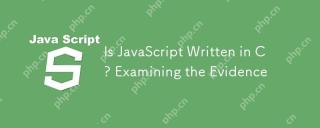
Yes, the engine core of JavaScript is written in C. 1) The C language provides efficient performance and underlying control, which is suitable for the development of JavaScript engine. 2) Taking the V8 engine as an example, its core is written in C, combining the efficiency and object-oriented characteristics of C. 3) The working principle of the JavaScript engine includes parsing, compiling and execution, and the C language plays a key role in these processes.
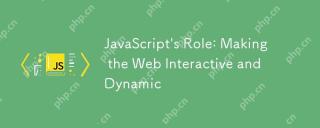
JavaScript is at the heart of modern websites because it enhances the interactivity and dynamicity of web pages. 1) It allows to change content without refreshing the page, 2) manipulate web pages through DOMAPI, 3) support complex interactive effects such as animation and drag-and-drop, 4) optimize performance and best practices to improve user experience.
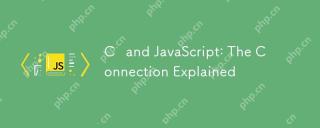
C and JavaScript achieve interoperability through WebAssembly. 1) C code is compiled into WebAssembly module and introduced into JavaScript environment to enhance computing power. 2) In game development, C handles physics engines and graphics rendering, and JavaScript is responsible for game logic and user interface.
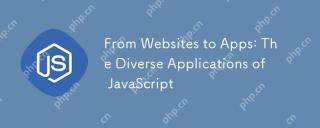
JavaScript is widely used in websites, mobile applications, desktop applications and server-side programming. 1) In website development, JavaScript operates DOM together with HTML and CSS to achieve dynamic effects and supports frameworks such as jQuery and React. 2) Through ReactNative and Ionic, JavaScript is used to develop cross-platform mobile applications. 3) The Electron framework enables JavaScript to build desktop applications. 4) Node.js allows JavaScript to run on the server side and supports high concurrent requests.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
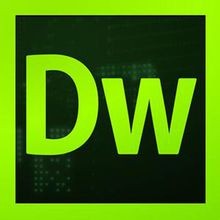
Dreamweaver CS6
Visual web development tools
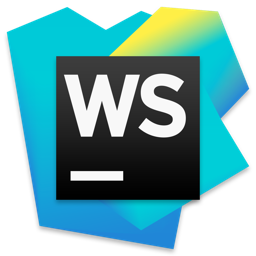
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor
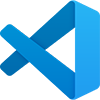
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
