


Info
At last week’s meeting, colleagues said that they are now using java to write function tests, which has produced a lot of redundant code and the entire project has become bloated. Now we urgently need a simple template project to quickly build a function test.
Later I went back and thought about it, why do we have to use java to do function test?
Node.js should be a good choice, and it has natural support for json, so I went back and searched on github, and there was indeed a related project: testosterone, so I came up with this blog.
Server
If you want to demo, you must have a corresponding server to support it.
Here we choose Express as the server.
First we create a server folder and create a new package.json.
{
"name": "wine-cellar ",
"description": "Wine Cellar Application",
"version": "0.0.1",
"private": true,
"dependencies": {
"express ": "3.x"
}
}
Next run command
npm install
Express is now installed.
We implement several simple get post methods for experiment
var express = require('express')
, app = express();
app.use(express.bodyParser());
app.get('/hello', function(req, res) {
res.send("hello world");
});
app.get('/ ', function (req, res) {
setTimeout(function () {
res.writeHead(200, {'Content-Type': 'text/plain'});
res.end() ;
}, 200);
});
app.get('/hi', function (req, res) {
if (req.param('hello') !== undefined) {
res.writeHead(200, {'Content -Type': 'text/plain'});
res.end('Hello!');
} else {
res.writeHead(500, {'Content-Type': 'text/ plain'});
res.end('use post instead');
}
});
app.post('/hi', function (req, res) {
setTimeout(function () {
res.writeHead(200, {'Content-Type': 'text/plain'} );
res.end(req.param('message') || 'message');
}, 100);
});
app.get('/user', function(req, res) {
res.send(
[
{name:'jack'},
{name: 'tom'}
]
);
});
app.get('/user/:id', function(req, res) {
res.send({
id: 1,
name: "node js",
description: "I am node js"
});
});
app.post('/user/edit', function (req, res) {
setTimeout(function () {
res.send({
id:req.param('id' ),
status:1
});
}, 100);
});
app.listen(3000);
console.log('Listening on port 3000 ...');
testosterone
After setting up the server, it’s natural to start testing.
The interfaces of this project are very elegantly named and can be directly loaded into the code.
First, test the basic functions
var testosterone = require('testosterone')({port: 3000})
, assert = testosterone.assert;
testosterone
.get('/hello',function(res){
assert.equal(res.statusCode, 200);
})
.get('/hi',function(res){
assert.equal(res.statusCode, 500);
})
.post('/hi', {data: {message: 'hola'}}, {
status: 200
,body: 'hola'
});
Then do a simple test on the get post of the user simulated above.
var testosterone = require('testosterone')({port : 3000})
, assert = testosterone.assert;
testosterone
.get('/user', function (res) {
var expectRes = [
{name:'jack'},
{name:'tom'}
];
assert.equal(res.statusCode, 200);
assert.equal(JSON.stringify(JSON.parse(res.body)),JSON.stringify(expectRes));
})
.get('/user/1', function (res) {
var user = JSON.parse(res.body);
assert.equal(res.statusCode, 200);
assert.equal(user.name, "node js");
assert.equal(user.description, "I am node js");
})
Next, if you want to use give when then to describe each test case, you can do this:
var testosterone = require('testosterone')({port : 3000, title: 'test user api'})
, add = testosterone.add
, assert = testosterone.assert;
testosterone
.add(
'GIVEN a user id to /user/{id} n'
'WHEN it have response user n'
'THEN it should return user json',
function (cb) {
testosterone.get('/user/1', cb(function (res) {
var expectRes = {
id: 1,
name: "node js",
description: "I am node js"
};
assert.equal(res.statusCode, 200);
assert.equal(JSON.stringify(JSON.parse(res.body)), JSON.stringify(expectRes));
}));
})
.add(
'GIVEN a POST a user info to /user/edit n'
'WHEN find modify success n'
'THEN it should resturn status 1',
function (cb) {
testosterone.post('/user/edit', {data: {id: 1, name: "change name"}}, cb(function (res) {
var res = JSON.parse(res.body);
assert.equal(res.status, 1);
}));
}
)
.run(function () {
require('sys').print('done!');
});
Conclusion
Through the above code, it can be seen that testosterone is indeed much simpler and more elegant than Java's lengthy http header settings.
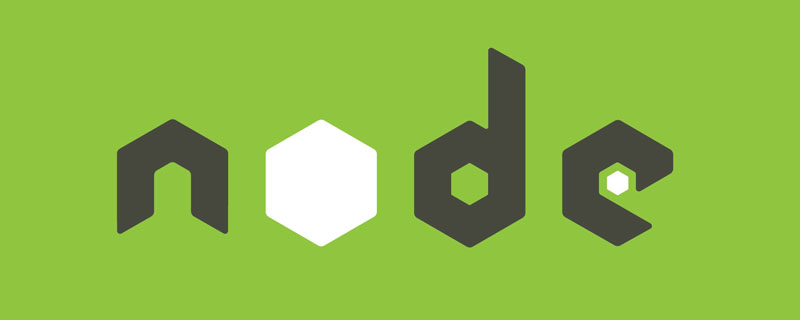
Vercel是什么?本篇文章带大家了解一下Vercel,并介绍一下在Vercel中部署 Node 服务的方法,希望对大家有所帮助!

gm是基于node.js的图片处理插件,它封装了图片处理工具GraphicsMagick(GM)和ImageMagick(IM),可使用spawn的方式调用。gm插件不是node默认安装的,需执行“npm install gm -S”进行安装才可使用。
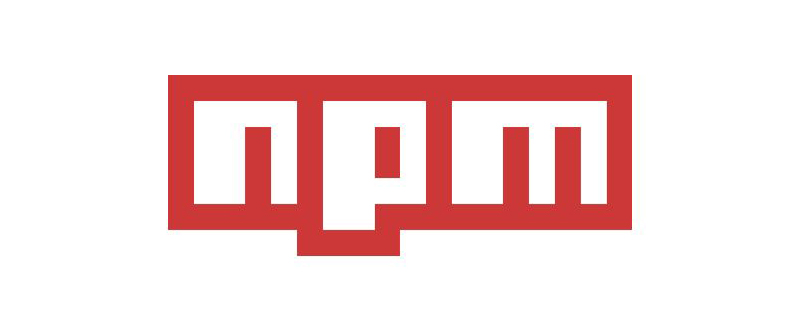
本篇文章带大家详解package.json和package-lock.json文件,希望对大家有所帮助!
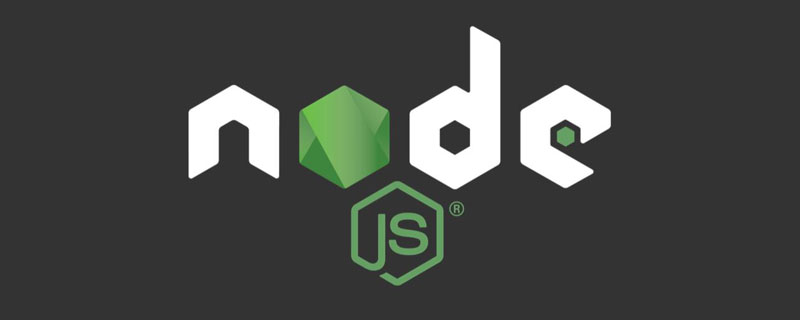
如何用pkg打包nodejs可执行文件?下面本篇文章给大家介绍一下使用pkg将Node.js项目打包为可执行文件的方法,希望对大家有所帮助!
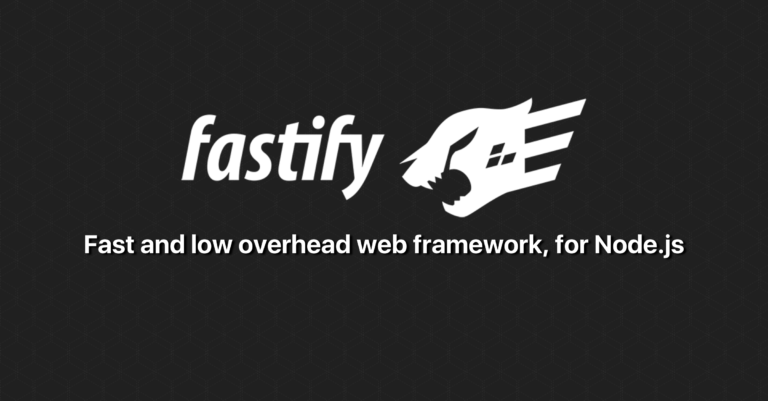
本篇文章给大家分享一个Nodejs web框架:Fastify,简单介绍一下Fastify支持的特性、Fastify支持的插件以及Fastify的使用方法,希望对大家有所帮助!
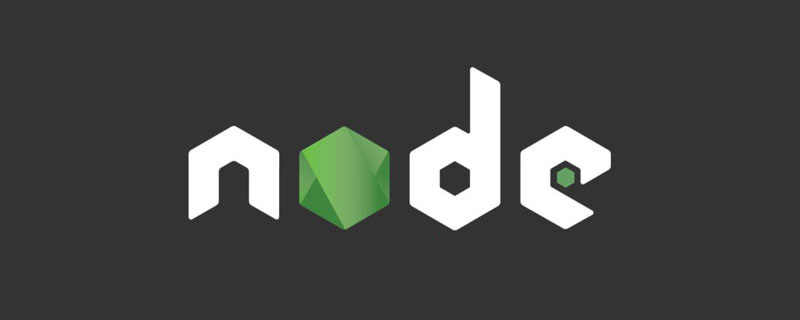
node怎么爬取数据?下面本篇文章给大家分享一个node爬虫实例,聊聊利用node抓取小说章节的方法,希望对大家有所帮助!

本篇文章给大家分享一个Node实战,介绍一下使用Node.js和adb怎么开发一个手机备份小工具,希望对大家有所帮助!
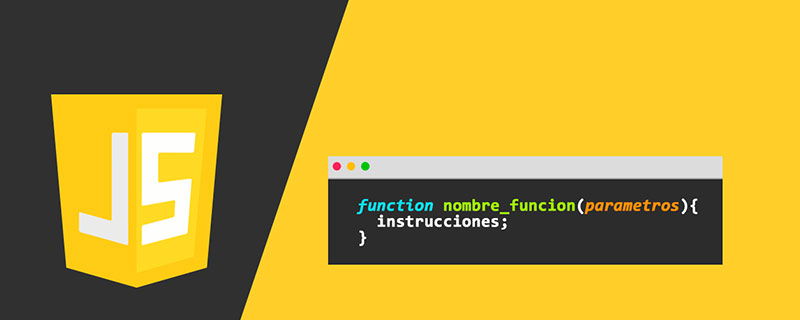
先介绍node.js的安装,再介绍使用node.js构建一个简单的web服务器,最后通过一个简单的示例,演示网页与服务器之间的数据交互的实现。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Zend Studio 13.0.1
Powerful PHP integrated development environment
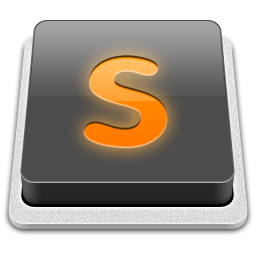
SublimeText3 Mac version
God-level code editing software (SublimeText3)
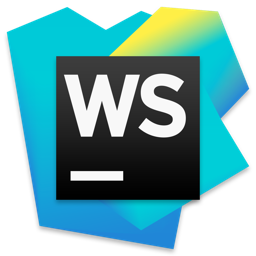
WebStorm Mac version
Useful JavaScript development tools