


Usage examples of Model and Collection in Backbone.js framework_Basic knowledge
Model
Regarding backbone, the most basic thing is the model. This thing is like the database mapping model in back-end development. It is also a model of data objects, and should have the same attributes as the back-end model. (Only properties that need to be manipulated through the front end).
Let's take you step by step through examples to understand what the backbone model is.
First define an html page:
<!DOCTYPE html> <html> <head> <title>the5fire-backbone-model</title> </head> <body> </body> <script src="http://ajax.googleapis.com/ajax/libs/jquery/1.4.4/jquery.min.js"></script> <script src="http://ajax.cdnjs.com/ajax/libs/underscore.js/1.1.4/underscore-min.js"></script> <script src="http://ajax.cdnjs.com/ajax/libs/backbone.js/0.3.3/backbone-min.js"></script> <script> (function ($) { /** *此处填充代码 **/ })(jQuery); </script> </html>
The following code needs to be filled in the function in the script tag of this html.
1. The simplest object
Man = Backbone.Model.extend({ initialize: function(){ alert('Hey, you create me!'); } }); var man = new Man;
This is very simple. There is also a model display in helloworld, and no attributes are defined. Here is a method during initialization, or called a constructor.
2. Two methods of object assignment
The first method is to define it directly and set the default value.
Man = Backbone.Model.extend({ initialize: function(){ alert('Hey, you create me!'); }, defaults: { name:'张三', age: '38' } }); var man = new Man; alert(man.get('name'));
The second type is defined when assigning
Man = Backbone.Model.extend({ initialize: function(){ alert('Hey, you create me!'); } }); man.set({name:'the5fire',age:'10'}); alert(man.get('name'));
Get is used when getting values.
3. Methods in objects
Man = Backbone.Model.extend({ initialize: function(){ alert('Hey, you create me!'); }, defaults: { name:'张三', age: '38' }, aboutMe: function(){ return '我叫' + this.get('name') + ',今年' + this.get('age') + '岁'; } }); var man = new Man; alert(man.aboutMe());
4. Monitor changes in attributes in objects
Man = Backbone.Model.extend({ initialize: function(){ alert('Hey, you create me!'); //初始化时绑定监听 this.bind("change:name",function(){ var name = this.get("name"); alert("你改变了name属性为:" + name); }); }, defaults: { name:'张三', age: '38' }, aboutMe: function(){ return '我叫' + this.get('name') + ',今年' + this.get('age') + '岁'; } }); var man = new Man; man.set({name:'the5fire'}) //触发绑定的change事件,alert。
5. Add validation rules and error prompts for objects
Man = Backbone.Model.extend({ initialize: function(){ alert('Hey, you create me!'); //初始化时绑定监听 this.bind("change:name",function(){ var name = this.get("name"); alert("你改变了name属性为:" + name); }); this.bind("error",function(model,error){ alert(error); }); }, defaults: { name:'张三', age: '38' }, validate:function(attributes){ if(attributes.name == '') { return "name不能为空!"; } }, aboutMe: function(){ return '我叫' + this.get('name') + ',今年' + this.get('age') + '岁'; } }); var man = new Man; man.set({name:''}); //根据验证规则,弹出错误提示。
6. Obtaining and saving objects requires server-side support to test.
First, you need to define a url attribute for the object. When the save method is called, all attributes of the object will be posted to the server.
Man = Backbone.Model.extend({ url:'/save/', initialize: function(){ alert('Hey, you create me!'); //初始化时绑定监听 this.bind("change:name",function(){ var name = this.get("name"); alert("你改变了name属性为:" + name); }); this.bind("error",function(model,error){ alert(error); }); }, defaults: { name:'张三', age: '38' }, validate:function(attributes){ if(attributes.name == '') { return "name不能为空!"; } }, aboutMe: function(){ return '我叫' + this.get('name') + ',今年' + this.get('age') + '岁'; } }); var man = new Man;; man.set({name:'the5fire'}); man.save(); //会发送POST到模型对应的url,数据格式为json{"name":"the5fire","age":38} //然后接着就是从服务器端获取数据使用方法fetch([options]) var man1 = new Man; //第一种情况,如果直接使用fetch方法,那么他会发送get请求到你model的url中, //你在服务器端可以通过判断是get还是post来进行对应的操作。 man1.fetch(); //第二种情况,在fetch中加入参数,如下: man1.fetch({url:'/getmans/'}); //这样,就会发送get请求到/getmans/这个url中, //服务器返回的结果样式应该是对应的json格式数据,同save时POST过去的格式。 //不过接受服务器端返回的数据方法是这样的: man1.fetch({url:'/getmans/',success:function(model,response){ alert('success'); //model为获取到的数据 alert(model.get('name')); },error:function(){ //当返回格式不正确或者是非json数据时,会执行此方法 alert('error'); }});
Note: The above codes are only codes that can be executed normally, but there will be examples on the server side later.
One more thing to add here is that all asynchronous operations on the server are completed through the Backbone.sync method. When this method is called, a parameter will be automatically passed and a corresponding request will be sent to the server based on the parameters. For example, if you save, Backbone will determine whether your object is new. If it is newly created, the parameter is create. If it is an existing object that has only been changed, then the parameter is update. If you call the fetch method, then The parameter is read. If it is destroy, then the parameter is delete. It is also called CRUD ("create", "read", "update", or "delete"), and the request types corresponding to these four parameters are POST, GET, PUT, and DELETE. You can perform corresponding CRUD operations on the server based on this request type.
Note:
Regarding url and urlRoot, if you set the url, then your CRUD will send the corresponding request to this url, but another problem is the delete request. The request is sent, but no data is sent, then you are The server does not know which object (record) should be deleted, so here is another concept of urlRoot. After you set urlRoot, when you send PUT and DELETE requests, the requested URL address is: /baseurl/[model.id ], so that you can update or delete the corresponding object (record) on the server side by extracting the value behind the url.
Collection
Collection is an ordered collection of model objects. The concept is very simple to understand. You will find it simpler after looking at a few examples.
1. Examples of book and bookshelf
Book = Backbone.Model.extend({ defaults : { // 感谢网友蓝色动力指正改为defaults title:'default' }, initialize: function(){ //alert('Hey, you create me!'); } }); BookShelf = Backbone.Collection.extend({ model : Book }); var book1 = new Book({title : 'book1'}); var book2 = new Book({title : 'book2'}); var book3 = new Book({title : 'book3'}); //var bookShelf = new BookShelf([book1, book2, book3]); //注意这里面是数组,或者使用add var bookShelf = new BookShelf; bookShelf.add(book1); bookShelf.add(book2); bookShelf.add(book3); bookShelf.remove(book3); //基于underscore这个js库,还可以使用each的方法获取collection中的数据 bookShelf.each(function(book){ alert(book.get('title')); });
It’s very simple, no explanation
2. Use fetch to get data from the server
First define the url in the Bookshelf above. Note that there is no urlRoot attribute in the collection. Or you define the value of url directly in the fetch method, as follows:
bookShelf.fetch({url:'/getbooks/',success:function(collection,response){ collection.each(function(book){ alert(book.get('title')); }); },error:function(){ alert('error'); }});
It also defines two methods that accept return values. I think the specific meaning is easy to understand. If the data in the correct format is returned, the success method will be called. If the data in the wrong format is returned, the error method will be called. Of course, the error method also looks Add the same formal parameters as the success method.
The corresponding return format of BookShelf is as follows: [{'title':'book1'},{'title':'book2'}....]
3. reset method
This method needs to be coordinated with the fetch above. After the collection fetches the data, it will call the reset method, so you need to define the reset method in the collection or bind the reset method. Here is a demo using binding:
bookShelf.bind('reset',showAllBooks); showAllBooks = function(){ bookShelf.each(function(book){ ​//将book数据渲染到页面。 }); }
The binding step must be performed before fetch.
The complete code for collection is given below, which requires server-side support. The server-side construction will be written later.
<!DOCTYPE html> <html> <head> <title>the5fire-backbone-collection</title> </head> <body> </body> <script src="http://ajax.googleapis.com/ajax/libs/jquery/1.4.4/jquery.min.js"></script> <script src="http://ajax.cdnjs.com/ajax/libs/underscore.js/1.1.4/underscore-min.js"></script> <script src="http://ajax.cdnjs.com/ajax/libs/backbone.js/0.3.3/backbone-min.js"></script> <script> (function ($) { //collection是一个简单的models的有序集合 //1、一个简单的例子 Book = Backbone.Model.extend({ defaults : { // 感谢网友蓝色动力指正改为defaults title:'default' }, initialize: function(){ //alert('Hey, you create me!'); } }); BookShelf = Backbone.Collection.extend({ model : Book }); var book1 = new Book({title : 'book1'}); var book2 = new Book({title : 'book2'}); var book3 = new Book({title : 'book3'}); //var bookShelf = new BookShelf([book1, book2, book3]); //注意这里面是数组,或者使用add var bookShelf = new BookShelf; bookShelf.add(book1); bookShelf.add(book2); bookShelf.add(book3); bookShelf.remove(book3); /* for(var i=0; i<bookShelf.models.length; i++) { alert(bookShelf.models[i].get('title')); } */ //基于underscore这个js库,还可以使用each的方法获取collection中的数据 bookShelf.each(function(book){ alert(book.get('title')); }); //2、使用fetch从服务器端获取数据,使用reset渲染 bookShelf.bind('reset', showAllBooks); bookShelf.fetch({url:'/getbooks/',success:function(collection,response){ collection.each(function(book){ alert(book.get('title')); }); },error:function(){ alert('error'); }}); showAllBooks = function(){ bookShelf.each(function(book){ ​//将book数据渲染到页面。 }); } //上述代码仅仅均为可正常执行的代码,不过关于服务器端的实例在后面会有。 })(jQuery); </script> </html>
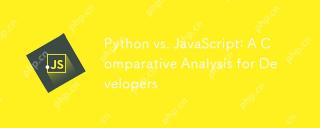
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
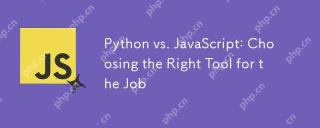
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
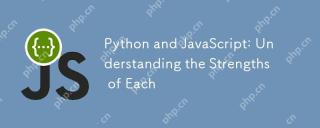
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
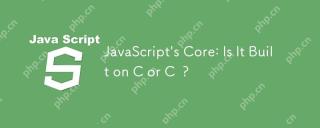
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
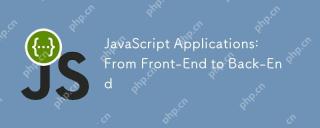
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
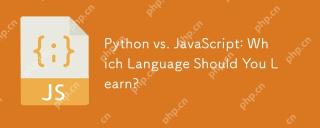
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
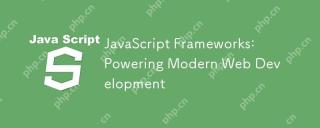
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
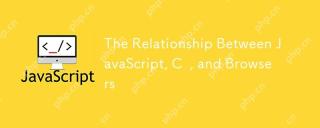
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
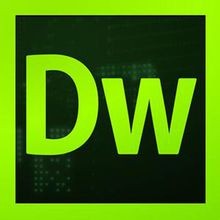
Dreamweaver CS6
Visual web development tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Atom editor mac version download
The most popular open source editor
