


JavaScript input email automatically prompts example code_javascript skills
I originally wanted to share my previous comments on artTemplate source code analysis, but after a year, I couldn’t find them, so I had to try it myself after analyzing the principles of the template engine at that time
I would like to share the template engine I wrote down with you as a souvenir. I remember that I compared several template engines at that time.
The js template engine mentioned here uses native javascript syntax, so it is very similar to php's native template engine.
What is the role of front-end template engine?
1. It can make front-end development simpler. There is no need to use operators to splice strings in order to generate a DOM structure. Instead, only one element (the HTML template inside) or a variable (which stores the template) is needed. Or a template file
2. Easy to maintain and reduce coupling. If your DOM structure changes, you do not need to change the logic code, but only need to change the corresponding template (file)
3. It can be cached. If your template is a file similar to .tpl, it can be loaded by the browser and still saved. Speaking of .tpl files, you can do more than cache, you can also do it through the module loader
By using .tpl as a module, you can load files on demand. Wouldn’t it save bandwidth and speed up the page?
4. Wait etc
What is the principle of front-end template engine?
The principle is very simple: object (data) + template (containing variables) -> string (html)
How to implement the front-end template engine?
By parsing the template, convert the template into a function according to the lexicon, and then call the function and pass the object (data) to output a string (html)
(Of course, the specific details depend on the code)
Like this:
var tpl = 'i am , years old'; // % Lexical, marked as variable
var obj = {
name : 'lovesueee' ,
Age : 24
};
var fn = Engine.compile(tpl); // Compile into function
var str = fn(obj); // Render out the string
Example:
<script><BR> var trs = [<BR> {name:"隐形杀手",age:29,sex:"男"},<BR> {name:"索拉",age:22,sex:"男"},<BR> {name:"fesyo",age:23,sex:"女"},<BR> {name:"恋妖壶",age:18,sex:"男"},<BR> {name:"竜崎",age:25,sex:"男"},<BR> {name:"你不懂的",age:30,sex:"女"}<BR> ] <P> // var html = ice("tpl",{<BR> // trs: trs,<BR> // href: "http://images.jb51.net/type4.jpg"<BR> // },{<BR> // title: function(){<BR> // return "<p>这是使用视图helper输出的代码片断"<BR> // }<BR> // });<BR> var elem = document.getElementById('tpl');<BR> var tpl = elem.innerHTML; <P> var html = ice(tpl,{<BR> trs: trs,<BR> href: "http://images.jb51.net/type4.jpg"<BR> },{<BR> title: function(){<BR> return "<p>这是使用视图helper输出的代码片断"<BR> }<BR> });<BR> console.log(html);<BR> $("#content").html(html);<br><br></script>
简单的实现:
(function (win) {
// 模板引擎路由函数
var ice = function (id, content) {
return ice[
typeof content === 'object' ? 'render' : 'compile'
].apply(ice, arguments);
};
ice.version = '1.0.0';
// 模板配置
var iConfig = {
openTag : ' closeTag : '%>'
};
var isNewEngine = !!String.prototype.trim;
// 模板缓存
var iCache = ice.cache = {};
// 辅助函数
var iHelper = {
include : function (id, data) {
return iRender(id, data);
},
print : function (str) {
return str;
}
};
// 原型继承
var iExtend = Object.create || function (object) {
function Fn () {};
Fn.prototype = object;
return new Fn;
};
// 模板编译
var iCompile = ice.compile = function (id, tpl, options) {
var cache = null;
id && (cache = iCache[id]);
if (cache) {
return cache;
}
// [id | tpl]
if (typeof tpl !== 'string') {
var elem = document.getElementById(id);
options = tpl;
if (elem) {
// [id, options]
options = tpl;
tpl = elem.value || elem.innerHTML;
} else {
//[tpl, options]
tpl = id;
id = null;
}
}
options = options || {};
var render = iParse(tpl, options);
id && (iCache[id] = render);
return render;
};
// 模板渲染
var iRender = ice.render = function (id, data, options) {
return iCompile(id, options)(data);
};
var iForEach = Array.prototype.forEach ?
function(arr, fn) {
arr.forEach(fn)
} :
function(arr, fn) {
for (var i = 0; i fn(arr[i], i, arr)
}
};
// 模板解析
var iParse = function (tpl, options) {
var html = [];
var js = [];
var openTag = options.openTag || iConfig['openTag'];
var closeTag = options.closeTag || iConfig['closeTag'];
// 根据浏览器采取不同的拼接字符串策略
var replaces = isNewEngine
?["var out='',line=1;", "out+=", ";", "out+=html[", "];", "this.result=out;"]
: ["var out=[],line=1;", "out.push(", ");", "out.push(html[", "]);", "this.result=out.join('');"];
// 函数体
var body = replaces[0];
iForEach(tpl.split(openTag), function(val, i) {
if (!val) {
return;
}
var parts = val.split(closeTag);
var head = parts[0];
var foot = parts[1];
var len = parts.length;
// html
if (len === 1) {
body += replaces[3] + html.length + replaces[4];
html.push(head);
} else {
if (head ) {
// code
// 去除空格
head = head
.replace(/^\s+|\s+$/g, '')
.replace(/[\n\r]+\s*/, '')
// 输出语句
if (head.indexOf('=') === 0) {
head = head.substring(1).replace(/^[\s]+|[\s;]+$/g, '');
body += replaces[1] + head + replaces[2];
} else {
body += head;
}
body = 'line =1;';
js.push(head);
}
// html
if (foot) {
_foot = foot.replace(/^[nr] s*/g, '');
if (!_foot) {
return;
}
body = replaces[3] html.length replaces[4];
html.push(foot);
}
}
});
body = "var Render=function(data){ice.mix(this, data);try{"
body
replaces[5]
"}catch(e){ice.log('rend error : ', line, 'line');ice.log('invalid statement : ', js[line-1]);throw e;}};"
"var proto=Render.prototype=iExtend(iHelper);"
"ice.mix(proto, options);"
"return function(data){return new Render(data).result;};";
var render = new Function('html', 'js', 'iExtend', 'iHelper', 'options', body);
return render(html, js, iExtend, iHelper, options);
};
ice.log = function () {
if (typeof console === 'undefined') {
return;
}
var args = Array.prototype.slice.call(arguments);
console.log.apply && console.log.apply(console, args);
};
// 合并对象
ice.mix = function (target, source) {
for (var key in source) {
if (source.hasOwnProperty(key)) {
target[key] = source[key];
}
}
};
// 注册函数
ice.on = function (name, fn) {
iHelper[name] = fn;
};
// 清除缓存
ice.clearCache = function () {
iCache = {};
};
// 更改配置
ice.set = function (name, value) {
iConfig[name] = value;
};
// 暴露接口
if (typeof module !== 'undefined' && module.exports) {
module.exports = template;
} else {
win.ice = ice;
}
})(window);
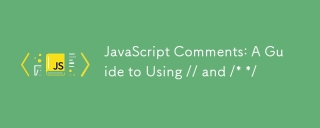
JavaScriptusestwotypesofcomments:single-line(//)andmulti-line(//).1)Use//forquicknotesorsingle-lineexplanations.2)Use//forlongerexplanationsorcommentingoutblocksofcode.Commentsshouldexplainthe'why',notthe'what',andbeplacedabovetherelevantcodeforclari
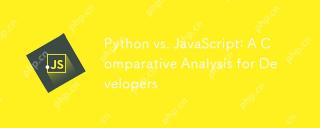
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
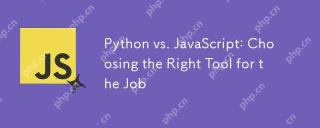
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
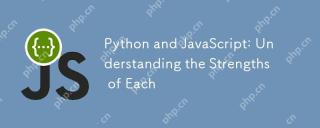
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
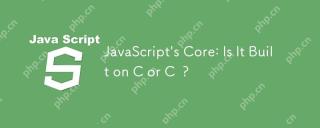
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
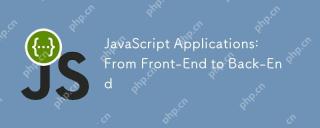
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
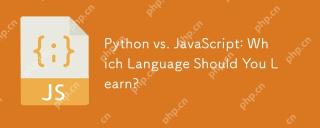
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
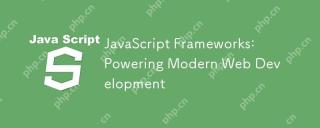
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
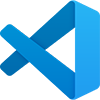
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Atom editor mac version download
The most popular open source editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
