


We usually sort out the randomly drawn cards in order from smallest to largest (I remember when I was a kid, I couldn’t catch two decks of cards). This essay is just to get familiar with this function. Related knowledge such as sorting arrays in js.
Knowledge points used:
1. Create objects in factory mode
2.js array sort() method
var testArr = [1, 3, 4, 2] ;
testArr.sort(function (a,b) {
return a - b;
})
alert(testArr.toString());//1,2,3,4
testArr.sort(function (a, b) {
return b- a;
})
alert(testArr.toString());//4,3,2,1
3.js-Math.radom() random number
Math.random();//0-1 The obtained random number is greater than or equal to 0 and less than 1
4.js Array splice usage
//The first parameter is the starting position of insertion
//The second parameter is the number of elements to be deleted from the starting position
//The third parameter is the element to be inserted from the starting position
//Example
var testArr = [1, 3, 4, 2];
testArr.splice(1, 0, 8);
alert(testArr.toString());//1,8,3,4,2
var testArr1 = [1, 3, 4, 2];
testArr1.splice(1, 1, 8);
alert(testArr1.toString());//1,8,3, 4,2
5.js array shift usage
// Take out the first element in the array and return it, the array Delete the first element
//Example
var testArr = [1, 3, 4, 2];
var k= testArr.shift();
alert(testArr.toString()) ;//3,4,2
alert(k);//1
With these basic knowledge, we can start playing cards. Assume that one person draws the cards. The hole card is random. Every time we draw a card, we must insert it into the cards in our hand to ensure the order. From childhood to adulthood!
Step 1: First we have to write a method to produce playing card objects:
/*Factory mode creates various cards
* number:The number on the card
*type:The suit of the card
*/
var Cards = (function () {
var Card = function (number, type) {
this.number = number;
this.type = type;
}
return function (number, type) {
return new Card(number, type);
}
})()
Step 2: Create playing cards, shuffle and store
var RadomCards = [];//Random card storage array
var MyCards = [];//Storage drawn cards
//Suit 0-Spade 1-Club 2-Diamond 3-Heart 4-Big Ghost 5-Little Ghost
//Numbers 0-13 represent ghosts, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, J ,Q,K;
function CreateCompeleteCard() {
var index = 2;
var arr = [];
for (var i = 0; i If (i == 0) {
arr[0] = new Cards(i, 4);
arr[1] = new Cards(i, 5);
} else {
for (var j = 0; j arr[index] = new Cards(i, j);
index ;
}
RadomCards = SortCards(arr);
Show();//Display the current card on the page
}
//Shuffle the cards
function SortCards(arr) {
arr .sort(function (a, b) {
return 0.5 - Math.random();
Step 3: Start drawing cards. When drawing cards, we must first determine the insertion position, and then insert the new cards into the designated position to form a new neat sequence
The code is as follows:
//How to draw cards
function GetCards(CardObj) {
var k = InCardsIndex(MyCards, CardObj);//Consider the insertion position
MyCards.splice(k, 0 , CardObj); // Insert to form a new sequence
}
/*[Get the position where the card should be inserted]
*arr: The card currently in hand
*obj: The newly drawn card
*/
function InCardsIndex(arr, obj) {
var len = arr && arr.length || 0;
if (len == 0) {
return 0;
Return 0;
}
} else {
var backi = -1;
for (var i = 0; i
if (obj. number if (backi == -1) {
Okay! Use the button on the html to start to draw cards, and click one card at a time! and show it
Copy code
The code is as follows:
var html = "";
lenNew for (var i = 0; i var html = "Upload html and css code
style="clear: both">
The card I drew:
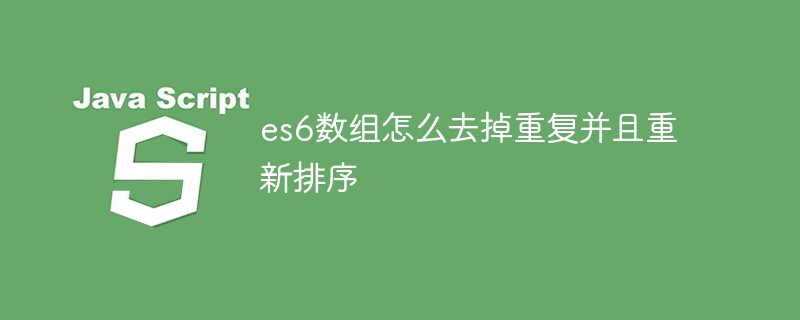
去掉重复并排序的方法:1、使用“Array.from(new Set(arr))”或者“[…new Set(arr)]”语句,去掉数组中的重复元素,返回去重后的新数组;2、利用sort()对去重数组进行排序,语法“去重数组.sort()”。
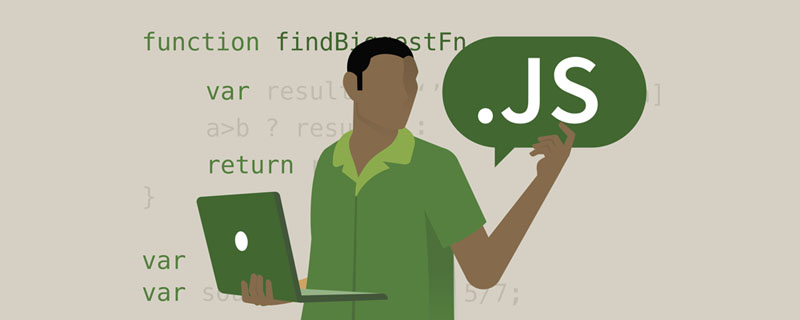
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于Symbol类型、隐藏属性及全局注册表的相关问题,包括了Symbol类型的描述、Symbol不会隐式转字符串等问题,下面一起来看一下,希望对大家有帮助。
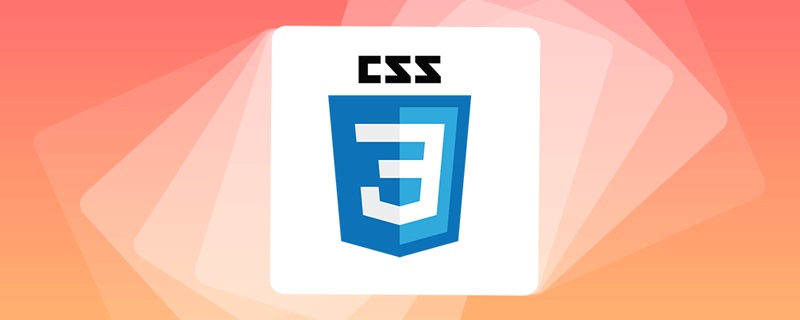
怎么制作文字轮播与图片轮播?大家第一想到的是不是利用js,其实利用纯CSS也能实现文字轮播与图片轮播,下面来看看实现方法,希望对大家有所帮助!
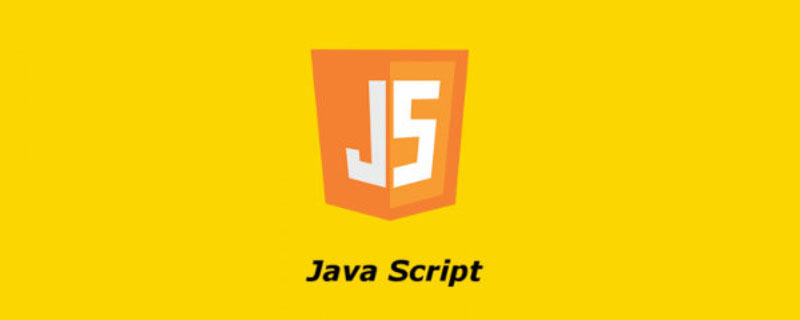
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于对象的构造函数和new操作符,构造函数是所有对象的成员方法中,最早被调用的那个,下面一起来看一下吧,希望对大家有帮助。
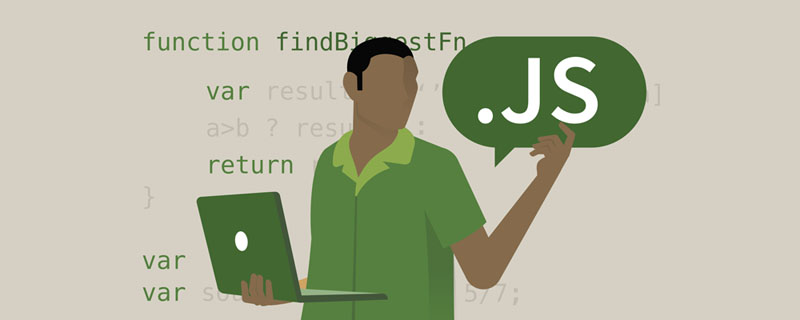
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于面向对象的相关问题,包括了属性描述符、数据描述符、存取描述符等等内容,下面一起来看一下,希望对大家有帮助。
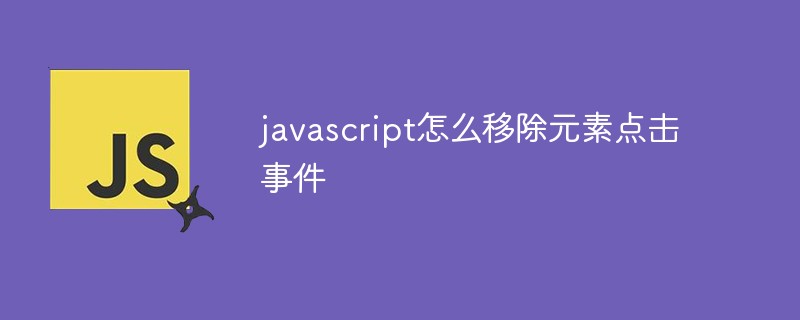
方法:1、利用“点击元素对象.unbind("click");”方法,该方法可以移除被选元素的事件处理程序;2、利用“点击元素对象.off("click");”方法,该方法可以移除通过on()方法添加的事件处理程序。
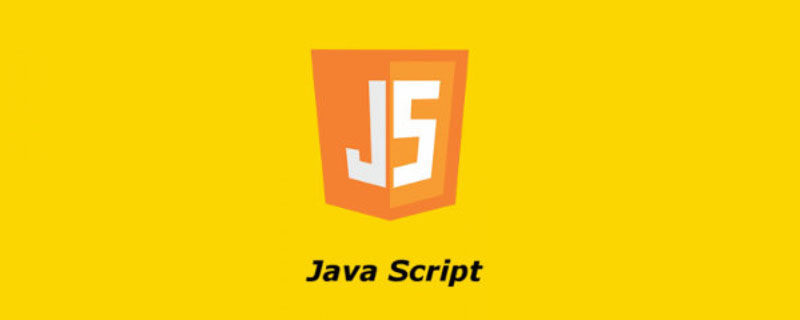
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于BOM操作的相关问题,包括了window对象的常见事件、JavaScript执行机制等等相关内容,下面一起来看一下,希望对大家有帮助。
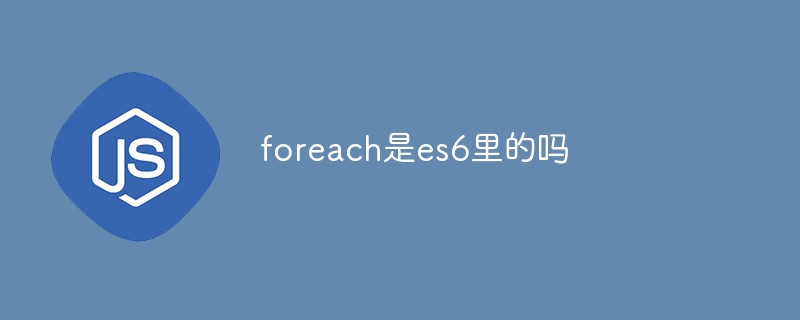
foreach不是es6的方法。foreach是es3中一个遍历数组的方法,可以调用数组的每个元素,并将元素传给回调函数进行处理,语法“array.forEach(function(当前元素,索引,数组){...})”;该方法不处理空数组。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
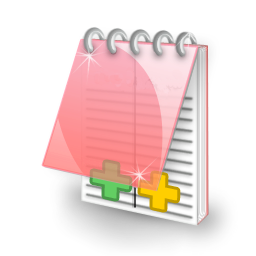
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 Linux new version
SublimeText3 Linux latest version
