package tk.dong.connection.util;import java.io.IOException;import java.io.InputStream;import java.io.PrintWriter;import java.sql.Array;import java.sql.Blob;import java.sql.CallableStatement;import java.sql.Clob;import java.sql.Connection;i
package tk.dong.connection.util; import java.io.IOException; import java.io.InputStream; import java.io.PrintWriter; import java.sql.Array; import java.sql.Blob; import java.sql.CallableStatement; import java.sql.Clob; import java.sql.Connection; import java.sql.DatabaseMetaData; import java.sql.DriverManager; import java.sql.NClob; import java.sql.PreparedStatement; import java.sql.SQLClientInfoException; import java.sql.SQLException; import java.sql.SQLFeatureNotSupportedException; import java.sql.SQLWarning; import java.sql.SQLXML; import java.sql.Savepoint; import java.sql.Statement; import java.sql.Struct; import java.util.LinkedList; import java.util.Map; import java.util.Properties; import java.util.concurrent.Executor; import java.util.logging.Logger; import javax.sql.DataSource; //这是同过增强Connection类[重写了close的方法]实现的从连接池取出连接并放回连接 public class JdbcPool implements DataSource { // 创建连接池,用的是LinkList, private static LinkedList<Connection> connections = new LinkedList<Connection>(); // 通过静态初始化块创建,当程序进行初始化的时候就会触发 static { // 将连接数据库的配置文件读入到流中 InputStream inStream = JdbcPool.class.getClassLoader().getResourceAsStream( "jdbc.properties"); Properties properties = new Properties(); try { properties.load(inStream); // 获取连接数据库的驱动(根据property属性文件中的属性获取驱动) Class.forName(properties.getProperty("driverClassName")); for (int i = 0; i < 10; i++) { // 获取conn连接连接对象 Connection conn = DriverManager.getConnection( properties.getProperty("url"), properties.getProperty("user"), properties.getProperty("pass")); // 这是在装入连接池的时候进行的操作处理,将connection中的close进行改写 MyConnection myConnection = new MyConnection(conn, connections); // 将处理后的连接对象存入连接池 connections.add(myConnection); System.out.println("连接池已经加入了::::::::::" + connections.size() + "::::::::::个链接对象"); } } catch (IOException e) { // TODO Auto-generated catch block e.printStackTrace(); } catch (ClassNotFoundException e) { // TODO Auto-generated catch block e.printStackTrace(); } catch (SQLException e) { // TODO Auto-generated catch block e.printStackTrace(); } } // 这两个是从连接池中获取连接的方法 @Override public Connection getConnection() throws SQLException { //生命连接对象 Connection conn=null; if(connections.size()>0){ //取出链表中的第一个对象赋值给临时的连接对象 conn=connections.removeFirst(); System.out.println("又一个连接对象被拿走:::::::连接池还有"+connections.size()+"个连接对象"); } return conn; } @Override public Connection getConnection(String username, String password) throws SQLException { // TODO Auto-generated method stub return null; } @Override public PrintWriter getLogWriter() throws SQLException { // TODO Auto-generated method stub return null; } @Override public void setLogWriter(PrintWriter out) throws SQLException { // TODO Auto-generated method stub } @Override public void setLoginTimeout(int seconds) throws SQLException { // TODO Auto-generated method stub } @Override public int getLoginTimeout() throws SQLException { // TODO Auto-generated method stub return 0; } @Override public Logger getParentLogger() throws SQLFeatureNotSupportedException { // TODO Auto-generated method stub return null; } @Override public <T> T unwrap(Class<T> iface) throws SQLException { // TODO Auto-generated method stub return null; } @Override public boolean isWrapperFor(Class<?> iface) throws SQLException { // TODO Auto-generated method stub return false; } } // 增强的Connection 连接对类 // 装饰器模式 // 1.首先看需要被增强对象继承了什么接口或父类,编写一个类也去继承这些接口或父类。 class MyConnection implements Connection { // 2.在类中定义一个变量,变量类型即需增强对象的类型。 // 用来接收连接池 private LinkedList<Connection> connections; // 用来接收连接对象 private Connection conn; // 构造器为 public MyConnection(Connection conn, LinkedList<Connection> connections) { this.conn = conn; this.connections = connections; } // 我只用到这个方法所以我只写这个方法 @Override public void close() throws SQLException { // 将不用的连接再次放入连接池 connections.add(conn); System.out.println("有一个连接对象用完了,已经返回连接池,现在连接池中还有==="+connections.size()); } // 下面的方法用不到,所以就不写内容了 @Override public <T> T unwrap(Class<T> iface) throws SQLException { // TODO Auto-generated method stub return null; } @Override public boolean isWrapperFor(Class<?> iface) throws SQLException { // TODO Auto-generated method stub return false; } @Override public Statement createStatement() throws SQLException { // TODO Auto-generated method stub return null; } @Override public PreparedStatement prepareStatement(String sql) throws SQLException { // TODO Auto-generated method stub return null; } @Override public CallableStatement prepareCall(String sql) throws SQLException { // TODO Auto-generated method stub return null; } @Override public String nativeSQL(String sql) throws SQLException { // TODO Auto-generated method stub return null; } @Override public void setAutoCommit(boolean autoCommit) throws SQLException { // TODO Auto-generated method stub } @Override public boolean getAutoCommit() throws SQLException { // TODO Auto-generated method stub return false; } @Override public void commit() throws SQLException { // TODO Auto-generated method stub } @Override public void rollback() throws SQLException { // TODO Auto-generated method stub } @Override public boolean isClosed() throws SQLException { // TODO Auto-generated method stub return false; } @Override public DatabaseMetaData getMetaData() throws SQLException { // TODO Auto-generated method stub return null; } @Override public void setReadOnly(boolean readOnly) throws SQLException { // TODO Auto-generated method stub } @Override public boolean isReadOnly() throws SQLException { // TODO Auto-generated method stub return false; } @Override public void setCatalog(String catalog) throws SQLException { // TODO Auto-generated method stub } @Override public String getCatalog() throws SQLException { // TODO Auto-generated method stub return null; } @Override public void setTransactionIsolation(int level) throws SQLException { // TODO Auto-generated method stub } @Override public int getTransactionIsolation() throws SQLException { // TODO Auto-generated method stub return 0; } @Override public SQLWarning getWarnings() throws SQLException { // TODO Auto-generated method stub return null; } @Override public void clearWarnings() throws SQLException { // TODO Auto-generated method stub } @Override public Statement createStatement(int resultSetType, int resultSetConcurrency) throws SQLException { // TODO Auto-generated method stub return null; } @Override public PreparedStatement prepareStatement(String sql, int resultSetType, int resultSetConcurrency) throws SQLException { // TODO Auto-generated method stub return null; } @Override public CallableStatement prepareCall(String sql, int resultSetType, int resultSetConcurrency) throws SQLException { // TODO Auto-generated method stub return null; } @Override public Map<String, Class<?>> getTypeMap() throws SQLException { // TODO Auto-generated method stub return null; } @Override public void setTypeMap(Map<String, Class<?>> map) throws SQLException { // TODO Auto-generated method stub } @Override public void setHoldability(int holdability) throws SQLException { // TODO Auto-generated method stub } @Override public int getHoldability() throws SQLException { // TODO Auto-generated method stub return 0; } @Override public Savepoint setSavepoint() throws SQLException { // TODO Auto-generated method stub return null; } @Override public Savepoint setSavepoint(String name) throws SQLException { // TODO Auto-generated method stub return null; } @Override public void rollback(Savepoint savepoint) throws SQLException { // TODO Auto-generated method stub } @Override public void releaseSavepoint(Savepoint savepoint) throws SQLException { // TODO Auto-generated method stub } @Override public Statement createStatement(int resultSetType, int resultSetConcurrency, int resultSetHoldability) throws SQLException { // TODO Auto-generated method stub return null; } @Override public PreparedStatement prepareStatement(String sql, int resultSetType, int resultSetConcurrency, int resultSetHoldability) throws SQLException { // TODO Auto-generated method stub return null; } @Override public CallableStatement prepareCall(String sql, int resultSetType, int resultSetConcurrency, int resultSetHoldability) throws SQLException { // TODO Auto-generated method stub return null; } @Override public PreparedStatement prepareStatement(String sql, int autoGeneratedKeys) throws SQLException { // TODO Auto-generated method stub return null; } @Override public PreparedStatement prepareStatement(String sql, int[] columnIndexes) throws SQLException { // TODO Auto-generated method stub return null; } @Override public PreparedStatement prepareStatement(String sql, String[] columnNames) throws SQLException { // TODO Auto-generated method stub return null; } @Override public Clob createClob() throws SQLException { // TODO Auto-generated method stub return null; } @Override public Blob createBlob() throws SQLException { // TODO Auto-generated method stub return null; } @Override public NClob createNClob() throws SQLException { // TODO Auto-generated method stub return null; } @Override public SQLXML createSQLXML() throws SQLException { // TODO Auto-generated method stub return null; } @Override public boolean isValid(int timeout) throws SQLException { // TODO Auto-generated method stub return false; } @Override public void setClientInfo(String name, String value) throws SQLClientInfoException { // TODO Auto-generated method stub } @Override public void setClientInfo(Properties properties) throws SQLClientInfoException { // TODO Auto-generated method stub } @Override public String getClientInfo(String name) throws SQLException { // TODO Auto-generated method stub return null; } @Override public Properties getClientInfo() throws SQLException { // TODO Auto-generated method stub return null; } @Override public Array createArrayOf(String typeName, Object[] elements) throws SQLException { // TODO Auto-generated method stub return null; } @Override public Struct createStruct(String typeName, Object[] attributes) throws SQLException { // TODO Auto-generated method stub return null; } @Override public void setSchema(String schema) throws SQLException { // TODO Auto-generated method stub } @Override public String getSchema() throws SQLException { // TODO Auto-generated method stub return null; } @Override public void abort(Executor executor) throws SQLException { // TODO Auto-generated method stub } @Override public void setNetworkTimeout(Executor executor, int milliseconds) throws SQLException { // TODO Auto-generated method stub } @Override public int getNetworkTimeout() throws SQLException { // TODO Auto-generated method stub return 0; } }测试代码
package tk.dong.connectionPool.test; import java.sql.Connection; import java.sql.SQLException; import org.junit.Test; import tk.dong.connection.util.JdbcPool; public class TestJdbcPool { @Test public void jdbcPool() throws SQLException { // 创建连接池对象 JdbcPool jdbcPool = new JdbcPool(); // 从连接池中获取连接对象 jdbcPool.getConnection(); // 多次获取连接对象 jdbcPool.getConnection(); jdbcPool.getConnection(); Connection conn = jdbcPool.getConnection(); // 归还用完的连接对象 conn.close(); // 再次获取连接对象 jdbcPool.getConnection(); jdbcPool.getConnection(); jdbcPool.getConnection(); jdbcPool.getConnection(); } }测试输出结果
连接池已经加入了::::::::::1::::::::::个链接对象 连接池已经加入了::::::::::2::::::::::个链接对象 连接池已经加入了::::::::::3::::::::::个链接对象 连接池已经加入了::::::::::4::::::::::个链接对象 连接池已经加入了::::::::::5::::::::::个链接对象 连接池已经加入了::::::::::6::::::::::个链接对象 连接池已经加入了::::::::::7::::::::::个链接对象 连接池已经加入了::::::::::8::::::::::个链接对象 连接池已经加入了::::::::::9::::::::::个链接对象 连接池已经加入了::::::::::10::::::::::个链接对象 又一个连接对象被拿走:::::::连接池还有9个连接对象 又一个连接对象被拿走:::::::连接池还有8个连接对象 又一个连接对象被拿走:::::::连接池还有7个连接对象 又一个连接对象被拿走:::::::连接池还有6个连接对象 有一个连接对象用完了,已经返回连接池,现在连接池中还有===7 又一个连接对象被拿走:::::::连接池还有6个连接对象 又一个连接对象被拿走:::::::连接池还有5个连接对象 又一个连接对象被拿走:::::::连接池还有4个连接对象 又一个连接对象被拿走:::::::连接池还有3个连接对象
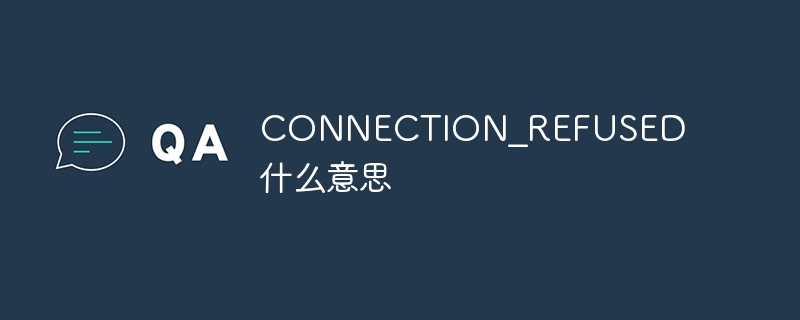
CONNECTION_REFUSED是一种网络连接错误,通常会在试图连接到远程服务器时出现。当客户端设备试图建立一个与服务器的网络连接时,如果服务器拒绝该连接请求,就会返回一个CONNECTION_REFUSED错误。常见的原因包括:服务器未启动、服务器无法接受更多的连接请求、服务器防火墙阻止了该连接等。
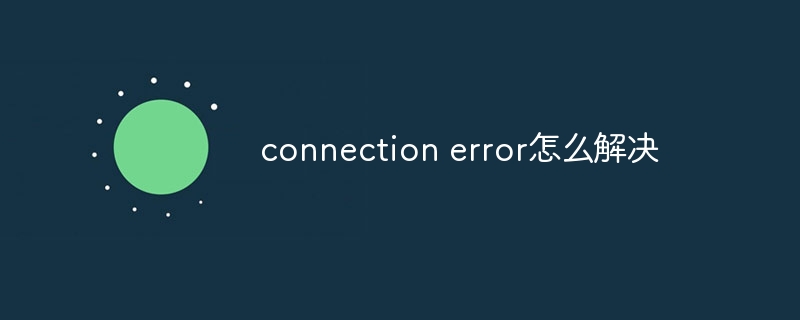
解决方法:1、检查网络连接;2、检查服务器状态;3、清除缓存和Cookie;4、检查防火墙和安全软件设置;5、尝试使用其他网络等等。
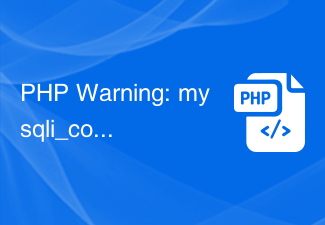
如果你使用PHP连接MySQL数据库时遇到了以下错误提示:PHPWarning:mysqli_connect():(HY000/2002):Connectionrefused那么你可以尝试按照下面的步骤来解决这个问题。确认MySQL服务是否正常运行首先应该检查MySQL服务是否正常运行,如果服务未运行或者启动失败,就可能会导致连接被拒绝的错误。你可
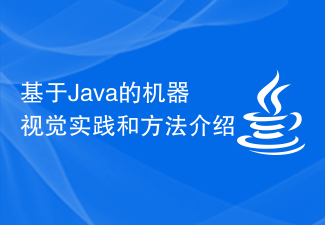
随着科技的不断发展,机器视觉技术在各个领域得到了广泛应用,如工业自动化、医疗诊断、安防监控等。Java作为一种流行的编程语言,其在机器视觉领域也有着重要的应用。本文将介绍基于Java的机器视觉实践和相关方法。一、Java在机器视觉中的应用Java作为一种跨平台的编程语言,具有跨操作系统、易于维护、高度可扩展等优点,对于机器视觉的应用具有一定的优越性。Java
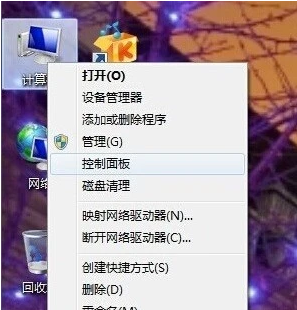
目前有很多屏幕亮度调整软件,我们可以通过使用软件进行快速调整或者通过显示器上自带的亮度功能进行调整。以下是详细的Win7屏幕亮度调整方式,您可以通过教程中的方法进行快速调整即可。Win7系统电脑怎么调节屏幕亮度教程:1、依次点击“计算机—右键—控制面板”,如果没有也可以在搜索框中进行搜索。2、点击控制面板下的“硬件和声音”,或者点击“外观和个性化”都可以。3、点击“NVIDIA控制面板”,有些显卡可能是AMD或者Intel的,请根据实际情况选择。4、调节图示中亮度滑块即可。5、还有一种方法,就是
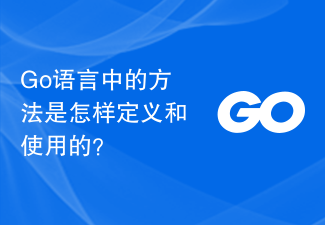
Go语言是近年来备受青睐的编程语言,因其简洁、高效、并发等特点而备受开发者喜爱。其中,方法(Method)也是Go语言中非常重要的概念。接下来,本文就将详细介绍Go语言中方法的定义和使用。一、方法的定义Go语言中的方法是带有接收器(Receiver)的函数,它是一个与某个类型绑定的函数。接收器可以是值类型或者指针类型。用于接收者的参数可以在方法名
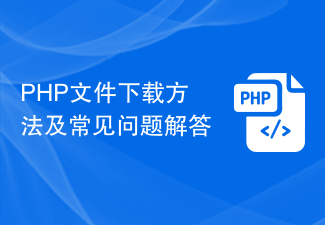
PHP是一个广泛使用的服务器端编程语言,它的许多功能和特性可以将其用于各种任务,包括文件下载。在本文中,我们将了解如何使用PHP创建文件下载脚本,并解决文件下载过程中可能出现的常见问题。一、文件下载方法要在PHP中下载文件,我们需要创建一个PHP脚本。让我们看一下如何实现这一点。创建下载文件的链接通过HTML或PHP在页面上创建一个链接,让用户能够下载文件。
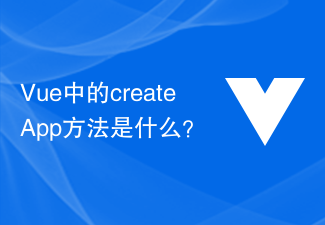
随着前端开发的快速发展,越来越多的框架被用来构建复杂的Web应用程序。Vue.js是流行的前端框架之一,它提供了许多功能和工具来简化开发人员构建高质量的Web应用程序。createApp()方法是Vue.js中的一个核心方法之一,它提供了一种简单的方式来创建Vue实例和应用程序。本文将深入探讨Vue中createApp方法的作用,其如何使用以及使用时需要了解


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
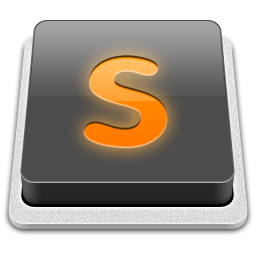
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Atom editor mac version download
The most popular open source editor

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
