


The most basic function of JavaScript in web pages is to monitor or respond to user actions, which is very useful. Some of the user's actions are very frequent, and some are very rare. Some listener functions execute lightning fast, while others are heavy-handed and can drag the browser to death. Take the resize event of the browser window as an example. This event will be triggered once for every scale change in the size of the browser window. If the listener is large, your browser will soon be overwhelmed.
Obviously, we cannot allow the browser to be brought down, but we cannot delete the delete listener. However, we can limit the frequency of function calls and weaken the impact of event function execution. Instead of triggering the listener function once for each size change of the window, we can now set the minimum interval for triggering the listener function to be greater than a certain number of milliseconds, so that it maintains a reasonable calling channel and ensures that the user experience is not destroyed. There is a good js tool library called Underscore.js, which has a simple method that allows you to easily create listeners that reduce the frequency of event function triggers.
JavaScript code
The code of the frequency reduction listener is very simple:
//Create listener
var updateLayout = _.debounce(function(e) {
// Does all the layout updating here
}, 500); // Run every 500 milliseconds at least
// Add the event listener
window.addEventListener("resize", updateLayout, false);
...The bottom layer of this Underscore.js code actually uses interval to check the frequency of event function calls:
// Returns a function, that, as long as it continues to be invoked, will not
// be triggered. The function will be called after it stops being called for
// N milliseconds. If `immediate` is passed, trigger the function on the
// leading edge, instead of the trailing.
_.debounce = function(func, wait, immediate) {
var timeout;
return function() {
var context = this, args = arguments;
var later = function() {
timeout = null;
If (!immediate) func.apply(context, args);
};
var callNow = immediate && !timeout;
clearTimeout(timeout);
timeout = setTimeout(later, wait);
if (callNow) func.apply(context, args);
};
};
The code is not particularly complicated, but it is a blessing not to have to write it myself. This debounce function does not depend on other Underscore.js functions, so you can add this method to your favorite js tool library, such as jQuery or MooTools, very easily:
// MooTools
Function.implement({
debounce: function(wait, immediate) {
var timeout,
func = this;
return function() {
var context = this, args = arguments;
var later = function() {
Timeout = null;
If (!immediate) func.apply(context, args);
};
var callNow = immediate && !timeout;
ClearTimeout(timeout);
Timeout = setTimeout(later, wait);
If (callNow) func.apply(context, args);
};
}
});
// Use it!
window.addEvent("resize", myFn.debounce(500));
As mentioned above, the resize event of the window is the most common place to use the frequency reduction operation. Another common place is to give automatic completion prompts based on the user's key input. I love collecting code snippets like this that can easily make your website more efficient. It is also recommended that you study Underscore.js, which provides a large number of very useful functions.
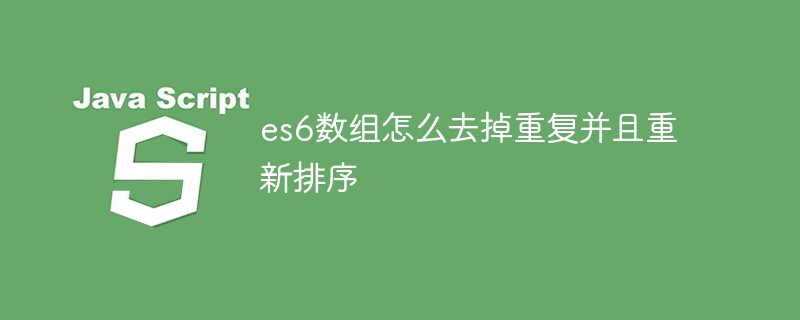
去掉重复并排序的方法:1、使用“Array.from(new Set(arr))”或者“[…new Set(arr)]”语句,去掉数组中的重复元素,返回去重后的新数组;2、利用sort()对去重数组进行排序,语法“去重数组.sort()”。
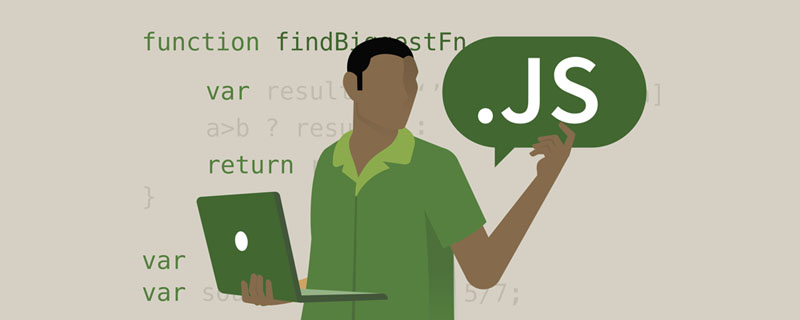
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于Symbol类型、隐藏属性及全局注册表的相关问题,包括了Symbol类型的描述、Symbol不会隐式转字符串等问题,下面一起来看一下,希望对大家有帮助。
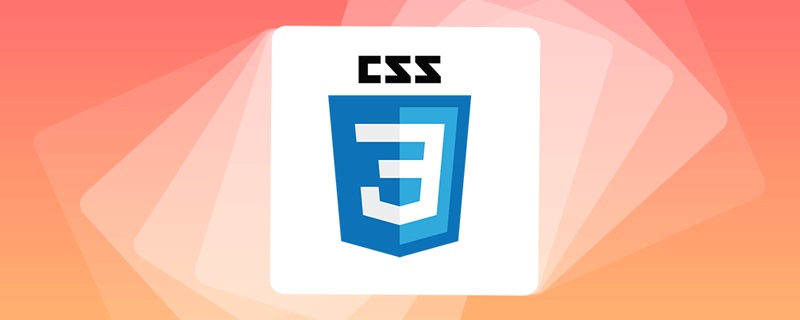
怎么制作文字轮播与图片轮播?大家第一想到的是不是利用js,其实利用纯CSS也能实现文字轮播与图片轮播,下面来看看实现方法,希望对大家有所帮助!
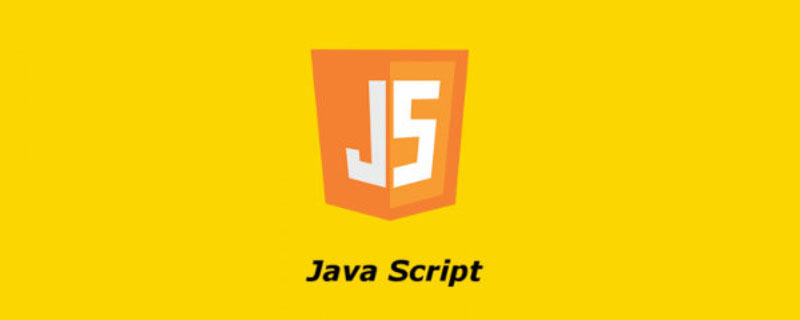
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于对象的构造函数和new操作符,构造函数是所有对象的成员方法中,最早被调用的那个,下面一起来看一下吧,希望对大家有帮助。
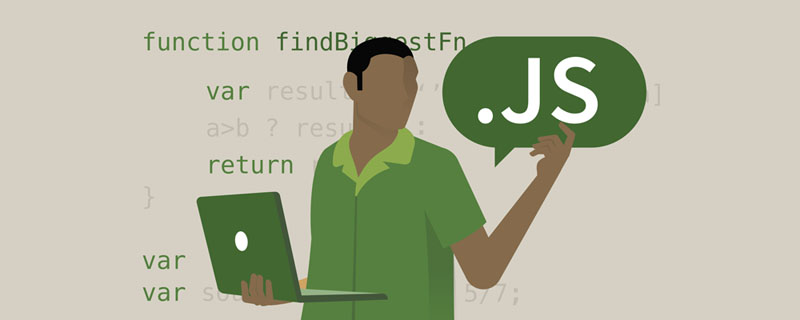
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于面向对象的相关问题,包括了属性描述符、数据描述符、存取描述符等等内容,下面一起来看一下,希望对大家有帮助。
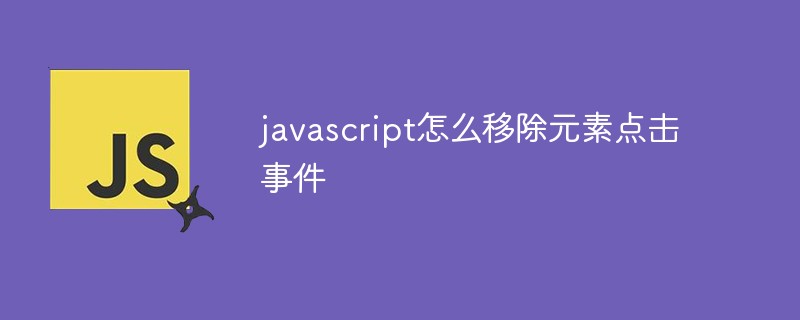
方法:1、利用“点击元素对象.unbind("click");”方法,该方法可以移除被选元素的事件处理程序;2、利用“点击元素对象.off("click");”方法,该方法可以移除通过on()方法添加的事件处理程序。
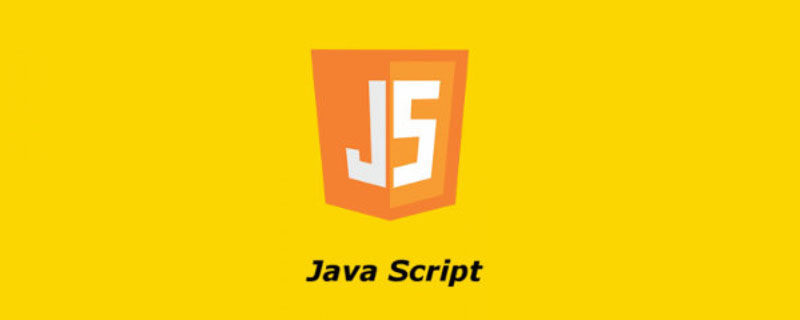
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于BOM操作的相关问题,包括了window对象的常见事件、JavaScript执行机制等等相关内容,下面一起来看一下,希望对大家有帮助。
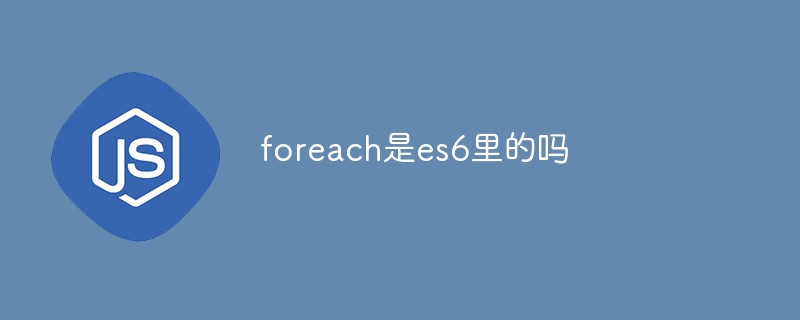
foreach不是es6的方法。foreach是es3中一个遍历数组的方法,可以调用数组的每个元素,并将元素传给回调函数进行处理,语法“array.forEach(function(当前元素,索引,数组){...})”;该方法不处理空数组。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
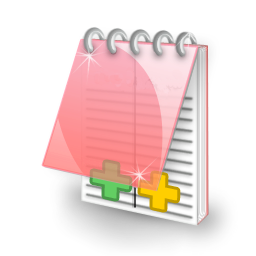
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
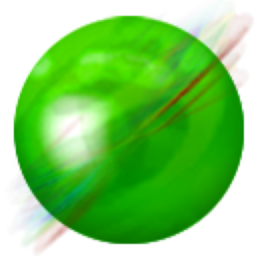
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
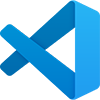
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
