题目:
<code>经理有三个女儿,年龄相加为13。 三个女儿的年龄相乘为经理的年龄,经理的一个手下知道 经理的年龄,但是不知道其三个女儿的年龄。 经理告诉手下有一个女儿头发是黑色的,手下立即知道了三个女儿的年龄。 请问三个女儿的年龄分别是多少?为什么? </code>
计算:
<br>function getAge($sum) { $ageLimit = 121; // 最大年龄121岁 $ageFrist = 18; //假设最小生育年龄 18岁 $posible = []; for ($c1 = 1; $c1 <= $sum; $c1++) { for ($c2 = 1; $c2 <= $sum; $c2++) { for ($c3 = 1; $c3 <= $sum; $c3++) { if ($c1 + $c2 + $c3 == $sum && $c1 * $c2 * $c3 < $ageLimit && $c1 * $c2 * $c3 - max($c1, $c2, $c3) >= $ageFrist) { $arr = [$c1, $c2, $c3]; asort($arr); $age = implode('-', $arr); if (!in_array($age, $posible)) { $posible[] = $age; } } } } } return $posible; }
输出:
<code>var_dump(getAge(13)); /** array (size=12) 0 => string '1-3-9' (length=5) 1 => string '1-4-8' (length=5) 2 => string '1-5-7' (length=5) 3 => string '1-6-6' (length=5) 4 => string '2-2-9' (length=5) 5 => string '2-3-8' (length=5) 6 => string '2-4-7' (length=5) 7 => string '2-5-6' (length=5) 8 => string '3-3-7' (length=5) 9 => string '3-4-6' (length=5) 10 => string '3-5-5' (length=5) 11 => string '4-4-5' (length=5) **/ </code>
以上输出答案错误。如何解答本题?
回复内容:
题目:
<code>经理有三个女儿,年龄相加为13。 三个女儿的年龄相乘为经理的年龄,经理的一个手下知道 经理的年龄,但是不知道其三个女儿的年龄。 经理告诉手下有一个女儿头发是黑色的,手下立即知道了三个女儿的年龄。 请问三个女儿的年龄分别是多少?为什么? </code>
计算:
<br>function getAge($sum) { $ageLimit = 121; // 最大年龄121岁 $ageFrist = 18; //假设最小生育年龄 18岁 $posible = []; for ($c1 = 1; $c1 <= $sum; $c1++) { for ($c2 = 1; $c2 <= $sum; $c2++) { for ($c3 = 1; $c3 <= $sum; $c3++) { if ($c1 + $c2 + $c3 == $sum && $c1 * $c2 * $c3 < $ageLimit && $c1 * $c2 * $c3 - max($c1, $c2, $c3) >= $ageFrist) { $arr = [$c1, $c2, $c3]; asort($arr); $age = implode('-', $arr); if (!in_array($age, $posible)) { $posible[] = $age; } } } } } return $posible; }
输出:
<code>var_dump(getAge(13)); /** array (size=12) 0 => string '1-3-9' (length=5) 1 => string '1-4-8' (length=5) 2 => string '1-5-7' (length=5) 3 => string '1-6-6' (length=5) 4 => string '2-2-9' (length=5) 5 => string '2-3-8' (length=5) 6 => string '2-4-7' (length=5) 7 => string '2-5-6' (length=5) 8 => string '3-3-7' (length=5) 9 => string '3-4-6' (length=5) 10 => string '3-5-5' (length=5) 11 => string '4-4-5' (length=5) **/ </code>
以上输出答案错误。如何解答本题?
做这种题我向来不行, 但是 太明显了, 你漏了几个条件:
经理的一个手下知道 经理的年龄,但是不知道其三个女儿的年龄。经理告诉手下有一个女儿头发是黑色的,手下立即知道了三个女儿的年龄。 -> 说明 针对 经理的年龄(三女儿年龄乘积), 女儿的年龄有多种选择.
经理告诉手下有一个女儿头发是黑色的 -> 应该是 "经理告诉手下只有一个女儿头发是黑色的", 说明 其他两个是小小孩, 头发不黑? (逻辑对否? 网上看到的...)
<?php // 用一个数组来保存可能性 $list = array(); // 列出所有可能性,年龄按从小到大试 for ($i = 1; $i < 13; $i++) { $rest = 13 - $i; for ($j = $i; $j <= $rest / 2; $j++) { $k = $rest - $j; $product = $i * $j * $k; array_push($list, array($i, $j, $k, $product)); } } // 按经理年龄排序 usort($list, function($a, $b) { return $a[3] - $b[3]; }); // 先看看所有可能性 foreach ($list as list($i, $j, $k, $p)) { echo "$i, $j, $k = $p\n"; } // 按年龄排除不可能的 $map = array(); foreach ($list as $t) { if ($t[0] + $t[1] + $t[2] + 14 < $t[3]) { $key = "$t[3]"; if (array_key_exists($key, $map)) { array_push($map[$key], $t); } else { $map[$key] = array($t); } } } // 找出不唯一的(因为唯一就不需要黑头发条件) $map = array_filter($map, function($v, $k) { return count($v) > 1; }, 1); // 二维转一维 $list = array(); foreach ($map as $k => $v) { $list = array_merge($list, $v); } // 找出年龄中只有一个大于2岁的(黑头发) // 关于多少岁头发变黑,只有找度娘了 $list = array_filter($list, function($t) { $temp = array_filter($t, function($v) { return $v > 2; }); return count($temp) == 2; }); // 输出结果 if (count($list) == 1) { echo "found " . json_encode($list[0]); } else { echo "not found"; } ?>
所有输出(最后一行是结果)
1, 1, 11 = 11 1, 2, 10 = 20 1, 3, 9 = 27 1, 4, 8 = 32 1, 5, 7 = 35 2, 2, 9 = 36 1, 6, 6 = 36 2, 3, 8 = 48 2, 4, 7 = 56 2, 5, 6 = 60 3, 3, 7 = 63 3, 4, 6 = 72 3, 5, 5 = 75 4, 4, 5 = 80 found [2,2,9,36]
我的二杆子 PHP 写得太恼火了,还是写 JS 顺手,哈哈!
python 代码
<code>#!/usr/bin/python # -*- coding:utf-8 -*- if __name__ == '__main__': s1 = [tuple(sorted([x, y, z])) for x in range(1,13) for y in range(1, 13) for z in range(1, 13) if x + y + z == 13 and 50 > x * y * z > 18] s2 = set(s1) result = [i for i in s2 if 35 > i[0] * i[1] * i[2] - max(i) > 18] print result</code>
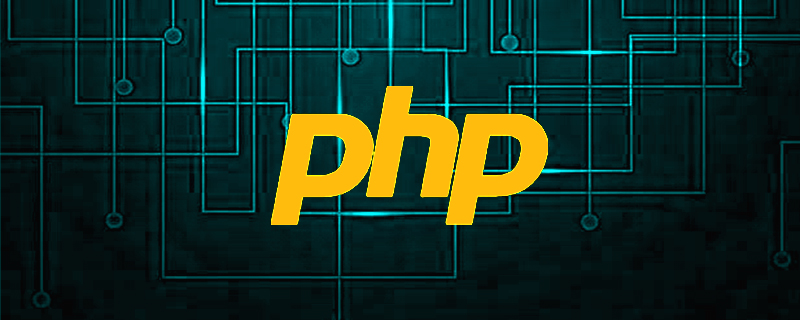
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
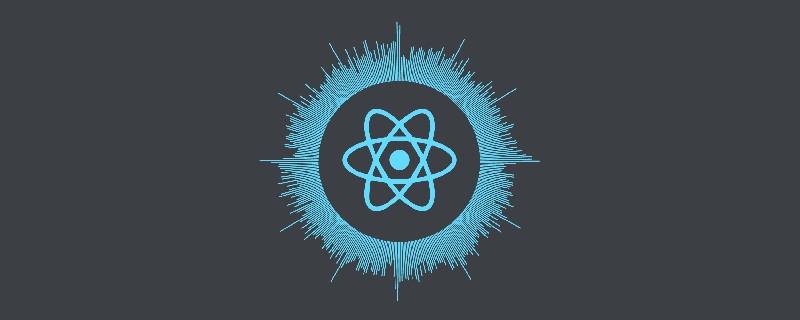
php中文网作为知名编程学习网站,为您整理了一些React面试题,帮助前端开发人员准备和清除React面试障碍。
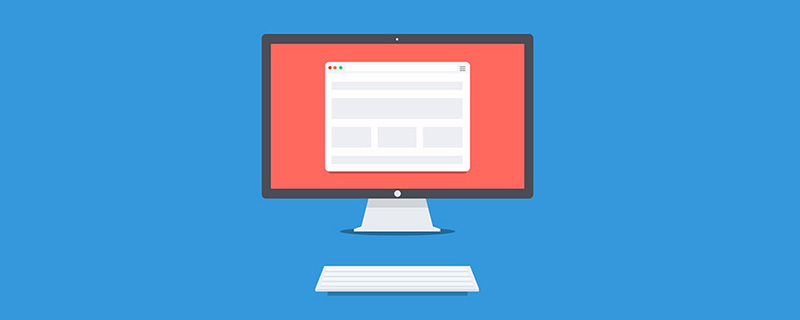
本篇文章给大家总结一些值得收藏的精选Web前端面试题(附答案)。有一定的参考价值,有需要的朋友可以参考一下,希望对大家有所帮助。
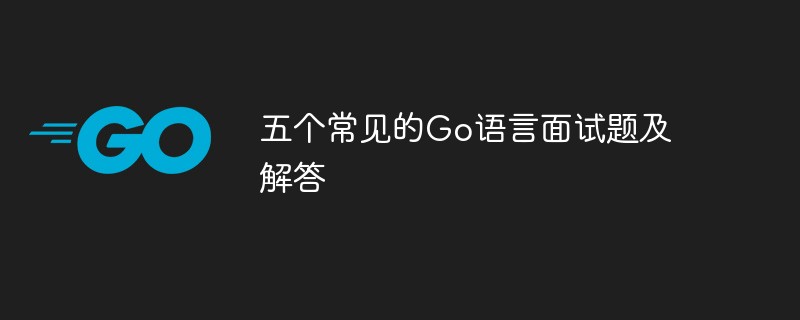
作为近年来备受热捧的一门编程语言,Go语言已经成为众多公司与企业的面试热点。对于Go语言初学者而言,在面试过程中遇到相关问题时,如何回答是一个值得探讨的问题。下面列举五个常见的Go语言面试题及解答,供初学者参考。请介绍一下Go语言的垃圾回收机制是如何工作的?Go语言的垃圾回收机制基于标记-清除算法和三色标记算法。当Go程序中的内存空间不够用时,Go垃圾回收器
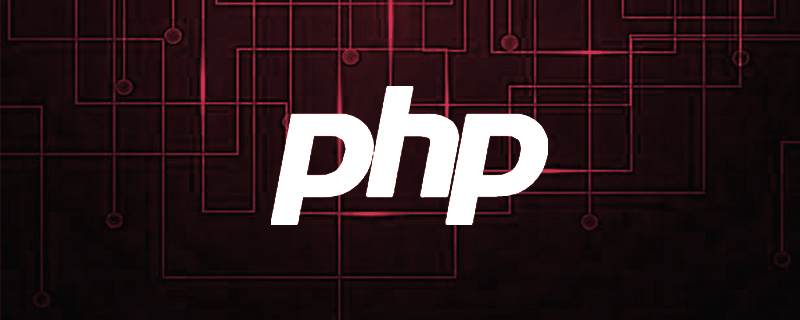
php判断有没有小数点的方法:1、使用“strpos(数字字符串,'.')”语法,如果返回小数点在字符串中第一次出现的位置,则有小数点;2、使用“strrpos(数字字符串,'.')”语句,如果返回小数点在字符串中最后一次出现的位置,则有。
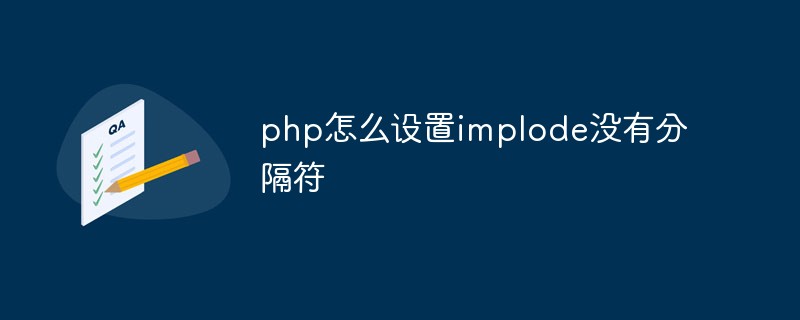
在PHP中,可以利用implode()函数的第一个参数来设置没有分隔符,该函数的第一个参数用于规定数组元素之间放置的内容,默认是空字符串,也可将第一个参数设置为空,语法为“implode(数组)”或者“implode("",数组)”。

转化方法:1、使用“mb_substr($url,stripos($url,"?")+1)”获取url的参数部分;2、使用“parse_str("参数部分",$arr)”将参数解析到变量中,并传入指定数组中,变量名转为键名,变量值转为键值。
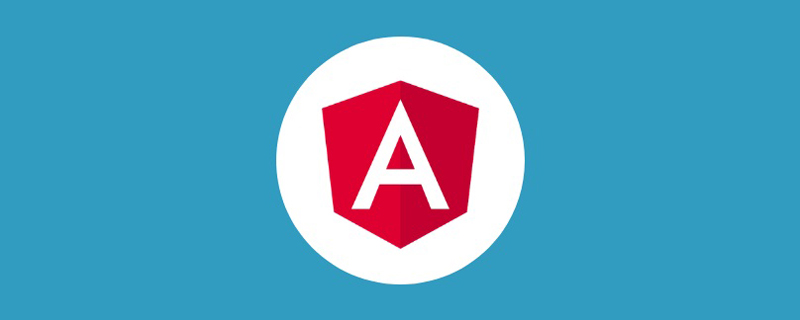
本篇文章给大家分享50个必须掌握的Angular面试题,会从初学者-中级-高级三个部分来解析这50个面试题,带大家吃透它们!


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
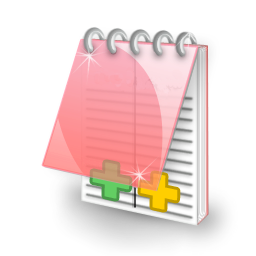
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
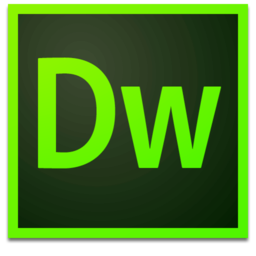
Dreamweaver Mac version
Visual web development tools

Atom editor mac version download
The most popular open source editor

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
