


Every Javascript function can access a special variable in its own scope - arguments. This variable contains a list of all arguments passed to the function.
The arguments object is not an array. Although syntactically it has the same features as an array, for example it has a length property. But it does not inherit from Array.prototype, in fact, it is an object.
Therefore, we cannot directly use some array methods such as push, pop or slice on arguments. So in order to use these methods, we need to convert it to a real array.
Convert to array
The following code will return an array containing all elements of the arguments object.
Array.prototype.slice.call(arguments);
Since the conversion is very slow, this is not recommended in performance-critical programs.
Pass parameters
The following is a recommended way to pass the arguments object from one function to another.
function foo() {
bar.apply(null, arguments);
}
function bar(a, b, c) {
// do stuff here
}
Another clever method is to use call and apply at the same time to quickly create an unbound outer method.
function Foo() {}
Foo.prototype.method = function(a, b, c) {
console.log(this, a, b, c);
};
// Create an unbound version of "method"
// It takes the parameters: this, arg1, arg2...argN
Foo.method = function() {
// Result: Foo.prototype.method.call(this, arg1, arg2... argN)
Function.call.apply(Foo.prototype.method, arguments);
};
The relationship between function parameters and arguments attribute
Thearguments object creates getter and setter methods for both its own properties and the formal parameters of its functions.
Therefore, modifying the formal parameters of a function will affect the property values of the corresponding arguments object, and vice versa.
function foo(a, b, c) {
arguments[0] = 2;
a; // 2
b = 4;
arguments[1]; // 4
var d = c;
d = 9;
c; // 3
}
foo(1, 2, 3);
Performance issues
arguments will not be created in only two situations, one is declared as a local variable inside the function, and the other is used as a formal parameter of the function. Otherwise, the arguments object is always created.
Since getter and setter methods are always created with the arguments object, using arguments itself has little impact on performance.
However, there is one situation that seriously affects the performance of Javascript, and that is the use of arguments.callee.
function foo() {
arguments.callee; // do something with this function object
arguments.callee.caller; // and the calling function object
}
function bigLoop() {
for(var i = 0; i foo(); // Would normally be inlined...
}
}
In the above code, the foo function is no longer a simple inline extension, because it needs to know both itself and its caller. This not only negates the performance gain brought by inline expansion, but also destroys the encapsulation of the function, because the function itself may need to depend on a specific calling context.
Therefore, it is recommended that you try not to use arguments.callee.
The above is all about the Javascript arguments object. Do you guys understand it thoroughly? Simply put
arguments refers to the parameter object of the function (referring to the actual parameters passed in)
arguments.length refers to the length of the parameter object of the function
arguments[i] refers to the value of the i-th parameter (the first one is 0)
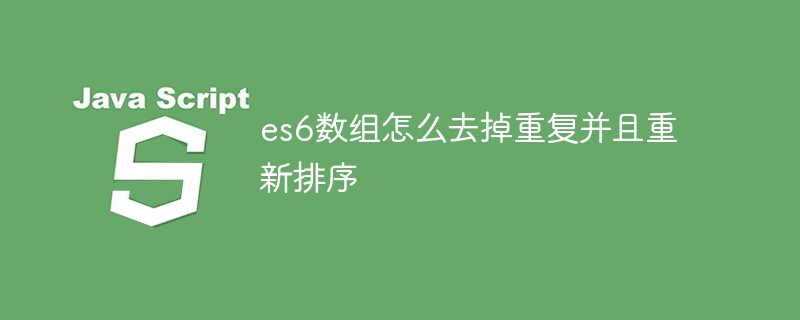
去掉重复并排序的方法:1、使用“Array.from(new Set(arr))”或者“[…new Set(arr)]”语句,去掉数组中的重复元素,返回去重后的新数组;2、利用sort()对去重数组进行排序,语法“去重数组.sort()”。
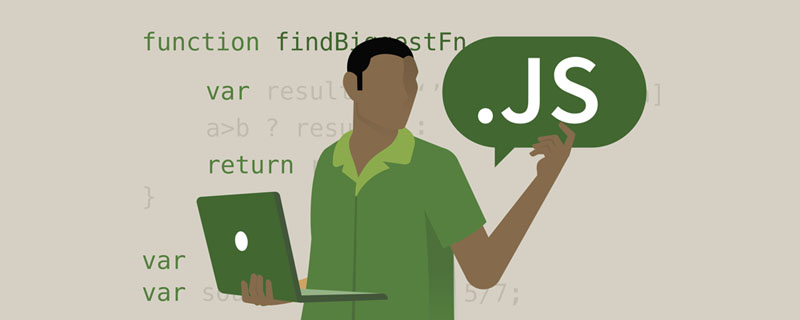
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于Symbol类型、隐藏属性及全局注册表的相关问题,包括了Symbol类型的描述、Symbol不会隐式转字符串等问题,下面一起来看一下,希望对大家有帮助。
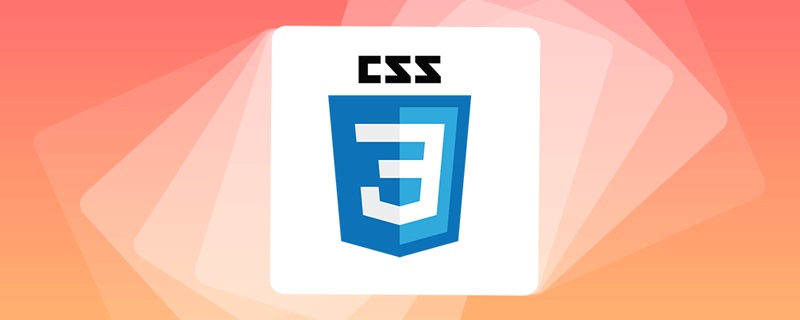
怎么制作文字轮播与图片轮播?大家第一想到的是不是利用js,其实利用纯CSS也能实现文字轮播与图片轮播,下面来看看实现方法,希望对大家有所帮助!
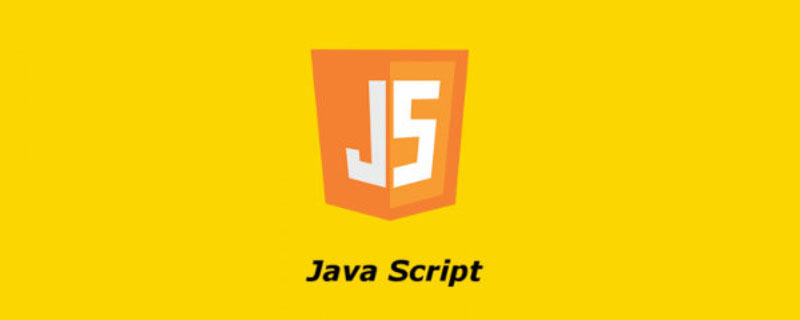
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于对象的构造函数和new操作符,构造函数是所有对象的成员方法中,最早被调用的那个,下面一起来看一下吧,希望对大家有帮助。
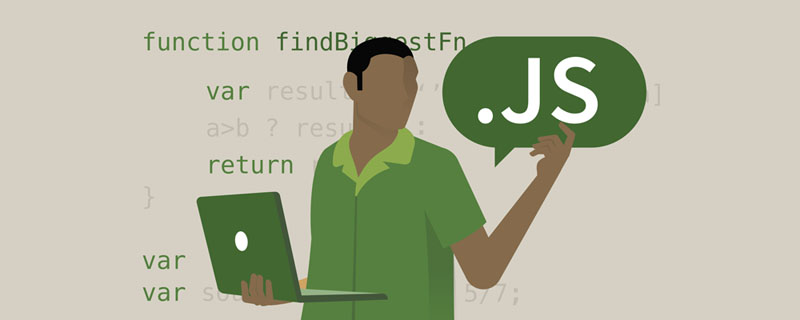
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于面向对象的相关问题,包括了属性描述符、数据描述符、存取描述符等等内容,下面一起来看一下,希望对大家有帮助。
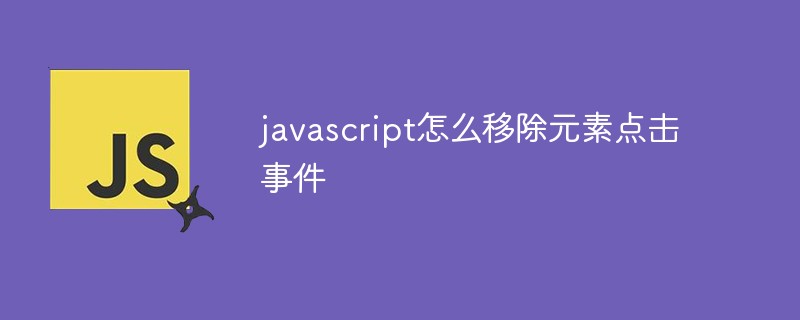
方法:1、利用“点击元素对象.unbind("click");”方法,该方法可以移除被选元素的事件处理程序;2、利用“点击元素对象.off("click");”方法,该方法可以移除通过on()方法添加的事件处理程序。
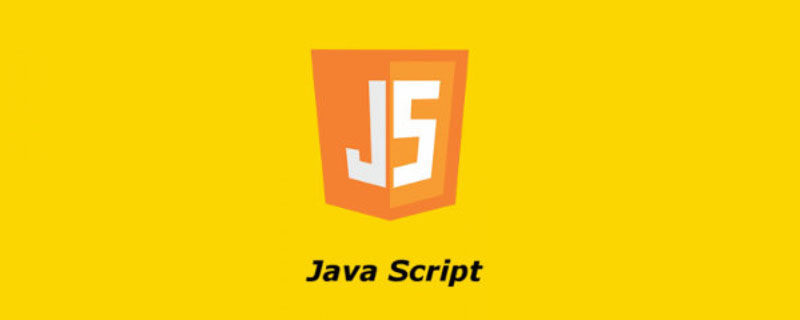
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于BOM操作的相关问题,包括了window对象的常见事件、JavaScript执行机制等等相关内容,下面一起来看一下,希望对大家有帮助。
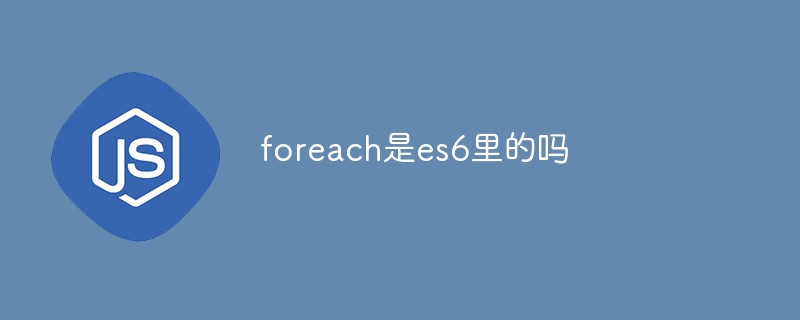
foreach不是es6的方法。foreach是es3中一个遍历数组的方法,可以调用数组的每个元素,并将元素传给回调函数进行处理,语法“array.forEach(function(当前元素,索引,数组){...})”;该方法不处理空数组。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
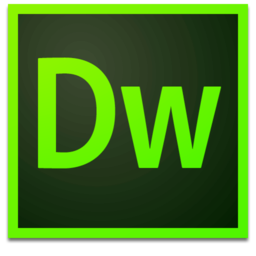
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
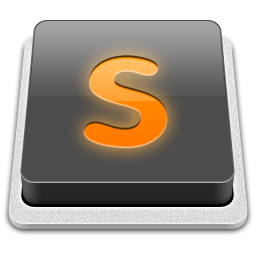
SublimeText3 Mac version
God-level code editing software (SublimeText3)
