Method description:
Write data to the file asynchronously. If the file already exists, the original content will be replaced.
Grammar:
fs.writeFile(filename, data, [options], [callback(err)])
Since this method belongs to the fs module, the fs module needs to be introduced before use (var fs= require(“fs”) )
Receive parameters:
filename (String) File name
data (String | Buffer) The content to be written can be string or buffer data.
options (Object) option array object, including:
· encoding (string) Optional value, default 'utf8', when data is buffer, the value should be ignored.
· mode (Number) File read and write permissions, default value 438
·flag (String) Default value ‘w’
callback {Function} callback, passing an exception parameter err.
Example:
fs.writeFile('message.txt', 'Hello Node', function (err) {
if (err) throw err;
console.log('It's saved!');
});
Source code:
fs.writeFile = function(path, data, options, callback) {
var callback = maybeCallback(arguments[arguments.length - 1]);
if (util.isFunction(options) || !options) {
Options = { encoding: 'utf8', mode: 438 /*=0666*/, flag: 'w' };
} else if (util.isString(options)) {
Options = { encoding: options, mode: 438, flag: 'w' };
} else if (!util.isObject(options)) {
Throw new TypeError('Bad arguments');
}
assertEncoding(options.encoding);
var flag = options.flag || 'w';
fs.open(path, options.flag || 'w', options.mode, function(openErr, fd) {
If (openErr) {
If (callback) callback(openErr);
} else {
var buffer = util.isBuffer(data) ? data : new Buffer('' data,
options.encoding || 'utf8');
var position = /a/.test(flag) ? null : 0;
writeAll(fd, buffer, 0, buffer.length, position, callback);
}
});
};
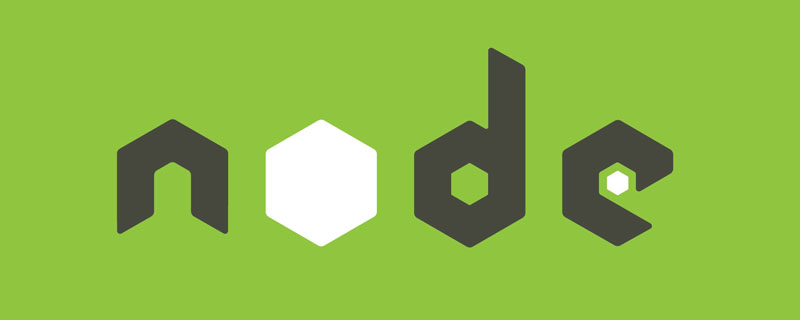
Vercel是什么?本篇文章带大家了解一下Vercel,并介绍一下在Vercel中部署 Node 服务的方法,希望对大家有所帮助!

gm是基于node.js的图片处理插件,它封装了图片处理工具GraphicsMagick(GM)和ImageMagick(IM),可使用spawn的方式调用。gm插件不是node默认安装的,需执行“npm install gm -S”进行安装才可使用。
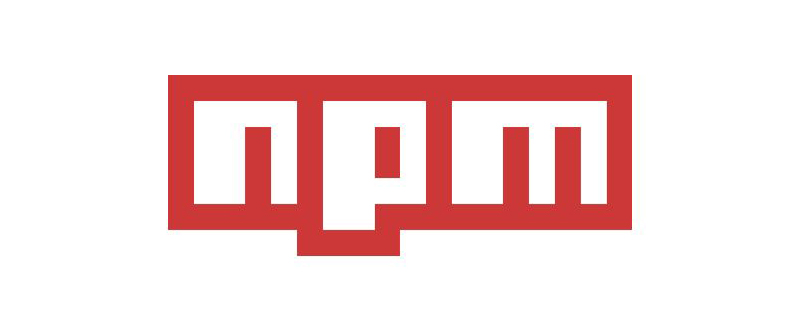
本篇文章带大家详解package.json和package-lock.json文件,希望对大家有所帮助!
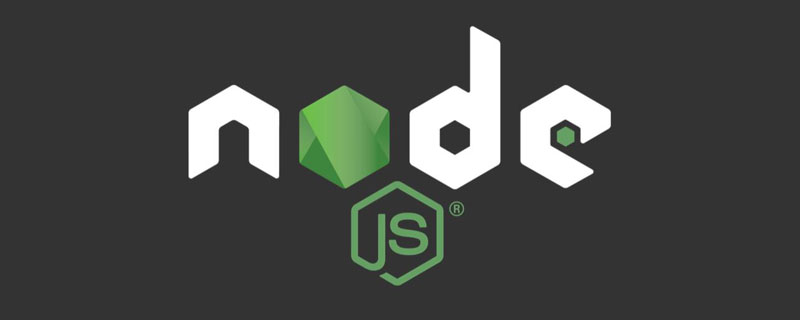
如何用pkg打包nodejs可执行文件?下面本篇文章给大家介绍一下使用pkg将Node.js项目打包为可执行文件的方法,希望对大家有所帮助!
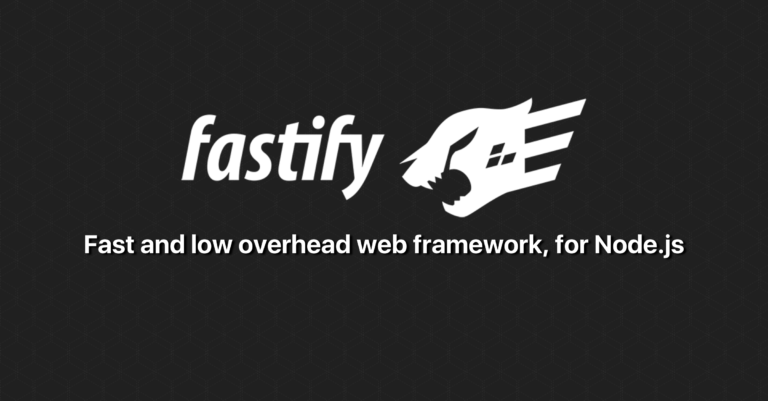
本篇文章给大家分享一个Nodejs web框架:Fastify,简单介绍一下Fastify支持的特性、Fastify支持的插件以及Fastify的使用方法,希望对大家有所帮助!
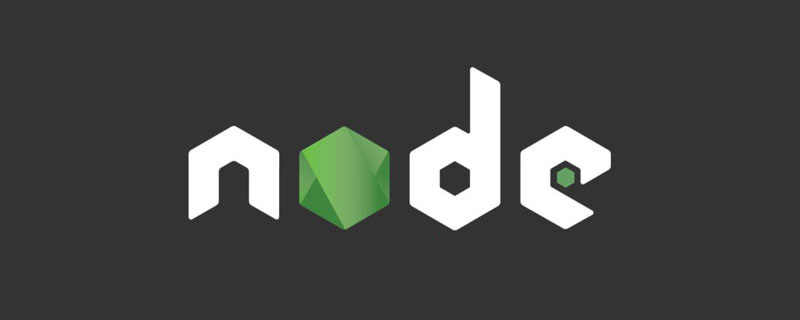
node怎么爬取数据?下面本篇文章给大家分享一个node爬虫实例,聊聊利用node抓取小说章节的方法,希望对大家有所帮助!

本篇文章给大家分享一个Node实战,介绍一下使用Node.js和adb怎么开发一个手机备份小工具,希望对大家有所帮助!
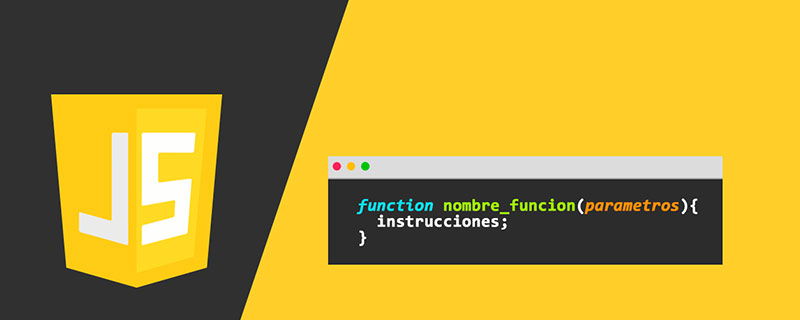
先介绍node.js的安装,再介绍使用node.js构建一个简单的web服务器,最后通过一个简单的示例,演示网页与服务器之间的数据交互的实现。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
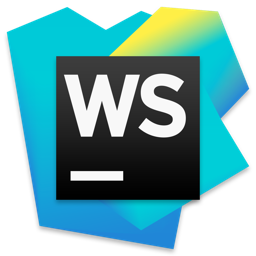
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
