Django Admin management tool
Django provides web-based management tools.
Django automatic management tool is part of django.contrib. You can see it in INSTALLED_APPS in the project's settings.py:
INSTALLED_APPS = ( 'django.contrib.admin', 'django.contrib.auth', 'django.contrib.contenttypes', 'django.contrib.sessions', 'django.contrib.messages', 'django.contrib.staticfiles', )
django.contrib is a huge feature set that is an integral part of the Django code base.
Activation Management Tool
Usually we will automatically set it in urls.py when we generate the project, we only need to remove the comment.
The configuration items are as follows:
from django.contrib import admin admin.autodiscover() # And include this URLpattern... urlpatterns = patterns('', # ... (r'^admin/', include(admin.site.urls)), # ... )
When all this is configured, the Django management tool can be run.
Use management tools
Start the development server, and then access: http://yoursite:8000/admin/ in the browser, you will get the following interface:
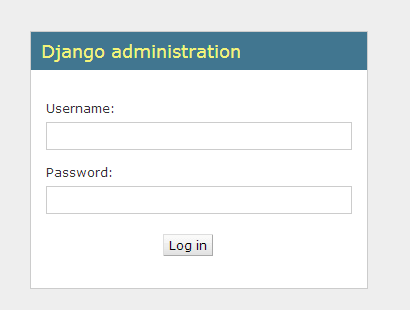
You can create a super user through the command python manage.py createsuperuser, as shown below:
# python manage.py createsuperuser Username (leave blank to use 'root'): admin Email address: admin@w3cschool.cc Password: Password (again): Superuser created successfully. [root@solar HelloWorld]#
Then enter the username and password to log in, The interface is as follows:
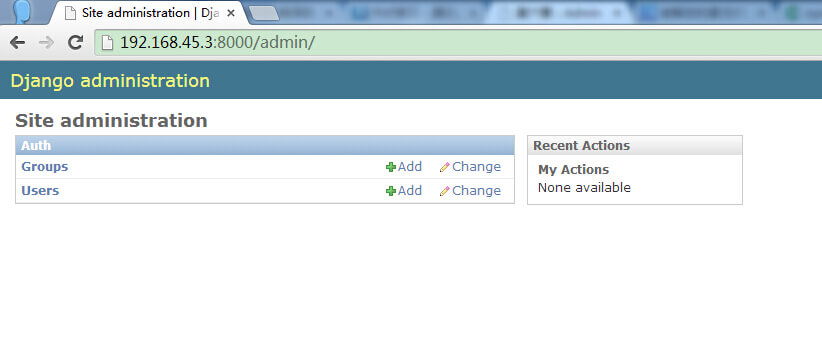
In order for the admin interface to manage a certain data model, we need to register the data model to the admin first. For example, we have previously created the model Test in TestModel. Modify TestModel/admin.py:
from django.contrib import admin from TestModel.models import Test # Register your models here. admin.site.register(Test)
After refreshing, you can see the Testmodel data table:
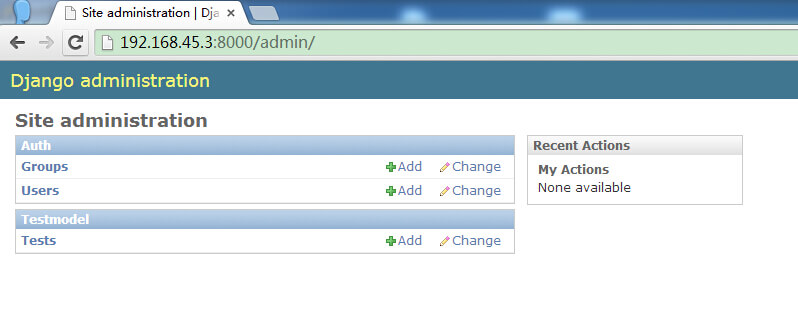
Complex model
The management page has powerful functions. Fully capable of handling more complex data models.
First add a more complex data model to TestModel/models.py:
from django.db import models # Create your models here. class Contact(models.Model): name = models.CharField(max_length=200) age = models.IntegerField(default=0) email = models.EmailField() def __unicode__(self): return self.name class Tag(models.Model): contact = models.ForeignKey(Contact) name = models.CharField(max_length=50) def __unicode__(self): return self.name
There are two tables here. Tag uses Contact as the external key. One Contact can correspond to multiple Tags.
We can also see many attribute types that we have not seen before, such as IntegerField for storing integers.
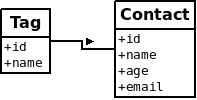
Register multiple models in TestModel/admin.py and display:
from django.contrib import admin from TestModel.models import Test,Contact,Tag # Register your models here. admin.site.register([Test, Contact, Tag])
Refresh the management page, the display results are as follows:
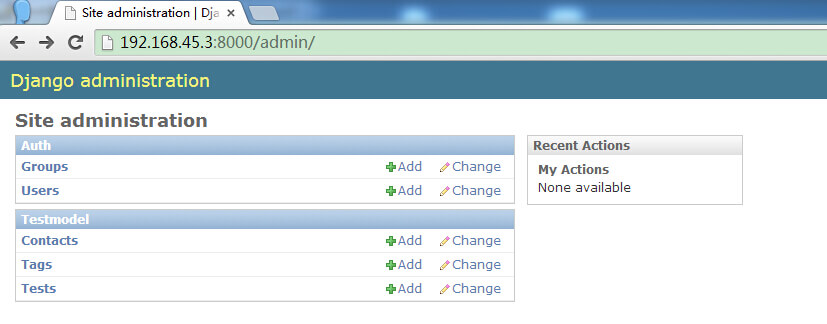
In the above management tools We can perform complex model operations.
Customized form
We can customize the management page to replace the default page. For example, the "add" page above. We want to display only the name and email parts. Modify TestModel/admin.py:
from django.contrib import admin from TestModel.models import Test,Contact,Tag # Register your models here. class ContactAdmin(admin.ModelAdmin): fields = ('name', 'email') admin.site.register(Contact, ContactAdmin) admin.site.register([Test, Tag])
The above code defines a ContactAdmin class to describe the display format of the management page.
The fields attribute inside defines the fields to be displayed.
Since this class corresponds to the Contact data model, we need to register them together when registering. The display effect is as follows:
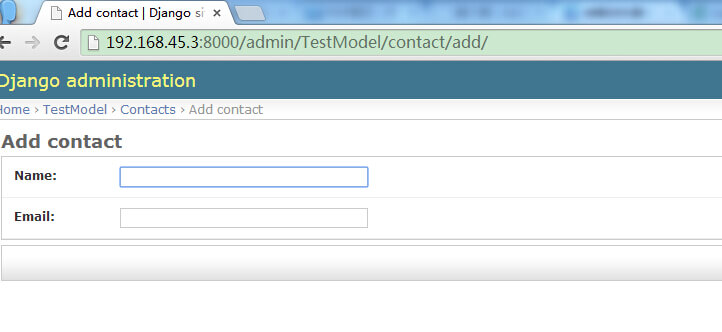
We can also divide the input fields into blocks, and each field can also define its own format. Modify TestModel/admin.py to:
from django.contrib import admin from TestModel.models import Test,Contact,Tag # Register your models here. class ContactAdmin(admin.ModelAdmin): fieldsets = ( ['Main',{ 'fields':('name','email'), }], ['Advance',{ 'classes': ('collapse',), # CSS 'fields': ('age',), }] ) admin.site.register(Contact, ContactAdmin) admin.site.register([Test, Tag])
The above column is divided into two parts: Main and Advance. classes describe the CSS format of the section in which they appear. Let the Advance part be hidden here:
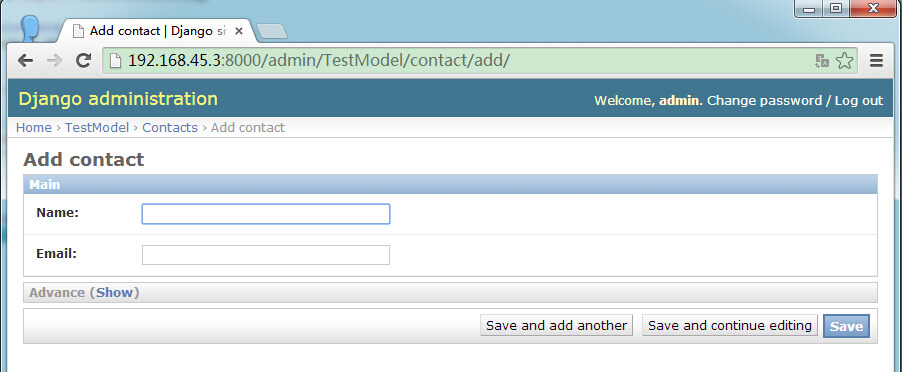
There is a Show button next to the Advance part for expansion. After expansion, you can click Hide to hide it, as shown in the following figure:
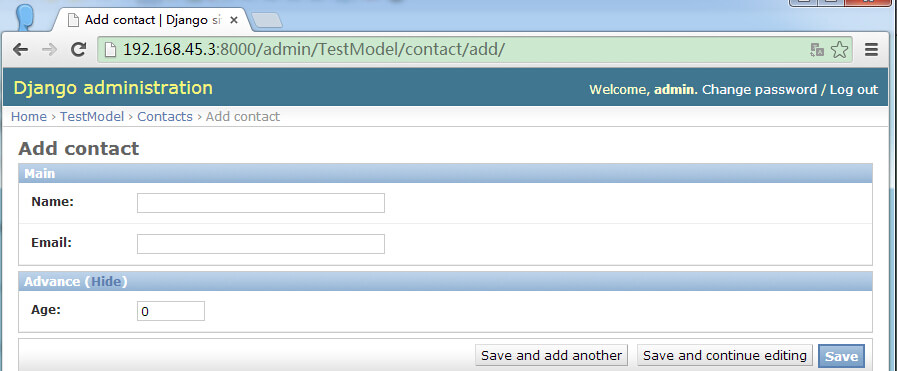
Inline display
The Contact above is the external key of Tag, so there is an external reference relationship.
In the default page display, the two are separated and the subordinate relationship between the two cannot be reflected. We can use inline display to attach the Tag to the Contact edit page.
Modify TestModel/admin.py:
from django.contrib import admin from TestModel.models import Test,Contact,Tag # Register your models here. class TagInline(admin.TabularInline): model = Tag class ContactAdmin(admin.ModelAdmin): inlines = [TagInline] # Inline fieldsets = ( ['Main',{ 'fields':('name','email'), }], ['Advance',{ 'classes': ('collapse',), 'fields': ('age',), }] ) admin.site.register(Contact, ContactAdmin) admin.site.register([Test])
The display effect is as follows:
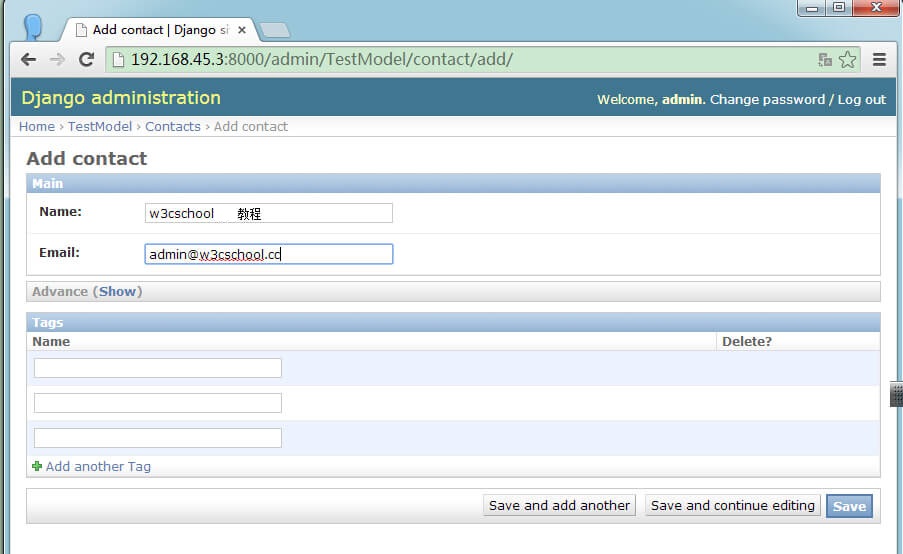
Display of the list page
Enter the number in Contact After recording, the Contact list page looks as follows:
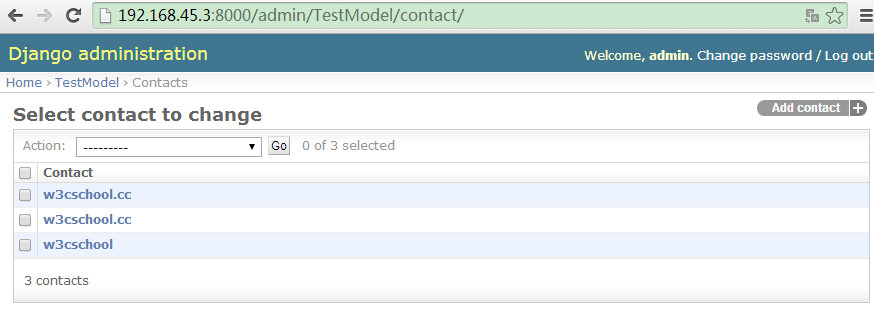
We can also customize the display of this page, such as displaying more columns in the list, just add the list_display attribute in ContactAdmin:
from django.contrib import admin from TestModel.models import Test,Contact,Tag # Register your models here. class ContactAdmin(admin.ModelAdmin): list_display = ('name','age', 'email') # list admin.site.register(Contact, ContactAdmin) admin.site.register([Test, Tag])
Refresh the page and the display effect is as follows:
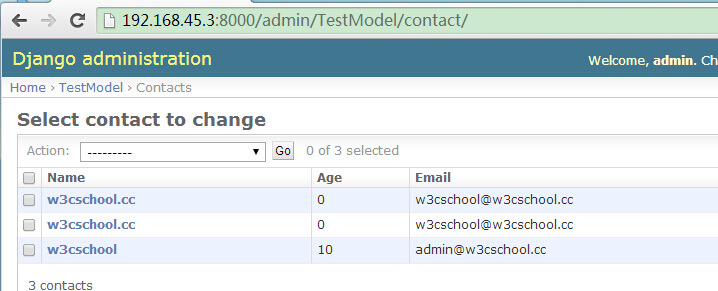
The search function is very useful when managing a large number of records. We can use search_fields to add a search bar to the list page:
from django.contrib import admin from TestModel.models import Test,Contact,Tag # Register your models here. class ContactAdmin(admin.ModelAdmin): list_display = ('name','age', 'email') search_fields = ('name',) admin.site.register(Contact, ContactAdmin) admin.site.register([Test])
In this example, we searched for records with the name w3cschool.cc (domain name of this site), and the results are as follows:
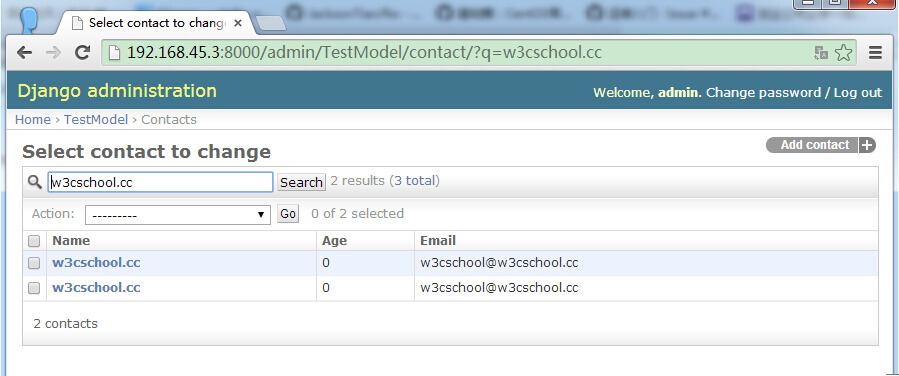
Django Admin management tool also has many practical functions, interested students You can study it in depth.