通过微信微小店API控制,微信订单处理。使其用户可以在会员卡中查看订单信息。
1. [文件]
weixiaodian.php
<?php class wXd { public $AppID = ""; public $AppSecret = ""; public $OutPut = ""; public $AccessToken = ""; public $ID = ""; public $HandleAT = array(); public $Logistics = array(); public function __construct($ID = '0'){ $this->ID = $ID; $this->sLogisticsList(); } public function cUrlRequest($url,$data = null){ $curl = curl_init(); curl_setopt($curl, CURLOPT_URL, $url); curl_setopt($curl, CURLOPT_SSL_VERIFYPEER, FALSE); curl_setopt($curl, CURLOPT_SSL_VERIFYHOST, FALSE); if (!empty($data)){ curl_setopt($curl, CURLOPT_POST, 1); curl_setopt($curl, CURLOPT_POSTFIELDS, $data); } curl_setopt($curl, CURLOPT_RETURNTRANSFER, 1); $output = curl_exec($curl); curl_close($curl); return $output; } //获取ACCESSTOKEN public function sAcessToken(){ $this->HandleAT = $this->gAccessToken(); if($this->HandleAT->expire_time < time()){ $appid = $this->AppID; $appsecret = $this->AppSecret; $url = "https://api.weixin.qq.com/cgi-bin/token?grant_type=client_credential&appid=".$appid."&secret=".$appsecret; $result = https_request($url); //echo '<pre class="brush:php;toolbar:false">'; var_dump($result);die; $jsoninfo = json_decode($result, true); $access_token = $jsoninfo["access_token"]; $this->pAccessToken($access_token); return $access_token; } else{ return $this->HandleAT->access_token; } } //保存ACCESSTOKEN public function pAccessToken($accesstoken){ $Path = $_SERVER['DOCUMENT_ROOT']."/jSon_file/access_token_".$this->ID.".json"; //print_r($Path); if(!file_exists($Path)){ touch($Path); chmod($Path,0777); } $data['expire_time'] = time() + 7000; $data['access_token'] = $accesstoken; $fp = fopen($Path, "w"); fwrite($fp, json_encode($data)); fclose($fp); } //读取ACCESSTOKEN public function gAccessToken(){ $Path = $_SERVER['DOCUMENT_ROOT']."/jSon_file/access_token_".$this->ID.".json"; if(!file_exists($Path)){ $data['expire_time'] = 0; $data['access_token'] = ''; } else{ $data = json_decode(file_get_contents($Path)); //print_r($data); } return $data; } //获取所有商品 public function gStateProduct($state = 0){ //http://www.php.cn/ //{"status": 0} $this->AccessToken = $this->sAcessToken(); $url = "https://api.weixin.qq.com/merchant/getbystatus?access_token=".$this->AccessToken; //print_r($this->AccessToken); $ResData = $this->cUrlRequest($url,'{"status": '.$state.'}'); //echo "<pre class="brush:php;toolbar:false">"; print_r( json_decode($ResData) ); } //设置微小店物流支持列表 public function sLogisticsList(){ $this->Logistics['Fsearch_code'] = "邮政EMS"; $this->Logistics['002shentong'] = "申通快递"; $this->Logistics['066zhongtong'] = "中通速递"; $this->Logistics['056yuantong'] = "圆通速递"; $this->Logistics['042tiantian'] = "天天快递"; $this->Logistics['003shunfeng'] = "顺丰速运"; $this->Logistics['059Yunda'] = "韵达快运"; $this->Logistics['064zhaijisong'] = "宅急送"; $this->Logistics['020huitong'] = "汇通快运"; $this->Logistics['zj001yixun'] = "易迅快递"; } //获取订单详情 public function gOrderInfo($order){ $this->AccessToken = $this->sAcessToken(); //print_r($this->AccessToken); $url = "https://api.weixin.qq.com/merchant/order/getbyid?access_token=".$this->AccessToken; $ResData = $this->cUrlRequest($url,'{"order_id": "'.$order.'"}'); //$url = "https://api.weixin.qq.com/merchant/order/getbyfilter?access_token=".$this->AccessToken; //$ResData = $this->cUrlRequest($url,'{"status": 2}'); print_r( json_decode($ResData) ); } //查询全部订单 public function gOrderAll($data = array()){ $this->AccessToken = $this->sAcessToken(); $url = "https://api.weixin.qq.com/merchant/order/getbyfilter?access_token=".$this->AccessToken; if(!empty($data)){ $data = json_encode($data); } else{ $firstday = strtotime(date("Y-m-01",time())); $data = array('begintime' => $firstday,'endtime' => strtotime("$firstday +1 month -1 day")); $data = json_encode($data); } $ResData = $this->cUrlRequest($url,$data); print_r( json_decode($ResData) ); } //设置订单发货 public function sOrderDelivery($data = array("need_delivery" => '0')){ $this->AccessToken = $this->sAcessToken(); $url = "https://api.weixin.qq.com/merchant/order/setdelivery?access_token=".$this->AccessToken; if(!empty($data)){ $data = json_encode($data); } else{ $data = array("need_delivery" => '0'); $data = json_encode($data); } $ResData = $this->cUrlRequest($url,$data); print_r( json_decode($ResData) ); } //关闭订单 public function sOrderClose($order){ $this->AccessToken = $this->sAcessToken(); $url = "https://api.weixin.qq.com/merchant/order/close?access_token=".$this->AccessToken; $ResData = $this->cUrlRequest($url,'{"order_id": "'.$order.'"}'); print_r( json_decode($ResData) ); } }
2. [代码]页面执行代码
<?php include_once 'class/weixiaodian.php'; $wXd = new wXd(); echo "<pre class="brush:php;toolbar:false">"; //查询全部商品 $wXd->gStateProduct(); //获取订单信息 $wXd->gOrderInfo('12963133879983601645'); //关闭订单 $wXd->sOrderClose('12963133879983600740'); //发货订单设置 $data['need_delivery'] = '1'; $data['order_id'] = '12963133879983600667'; $data['delivery_company'] = '059Yunda'; $data['delivery_track_no'] = '1000464090326'; $wXd->sOrderDelivery($data); //获取所有订单 $wXd->gOrderAll(); echo "";

Heiße KI -Werkzeuge

Undresser.AI Undress
KI-gestützte App zum Erstellen realistischer Aktfotos

AI Clothes Remover
Online-KI-Tool zum Entfernen von Kleidung aus Fotos.

Undress AI Tool
Ausziehbilder kostenlos

Clothoff.io
KI-Kleiderentferner

Video Face Swap
Tauschen Sie Gesichter in jedem Video mühelos mit unserem völlig kostenlosen KI-Gesichtstausch-Tool aus!

Heißer Artikel

Heiße Werkzeuge
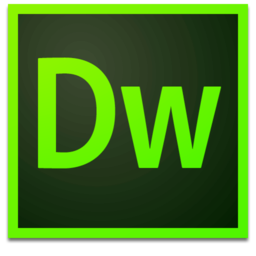
Dreamweaver Mac
Visuelle Webentwicklungstools
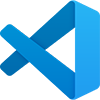
VSCode Windows 64-Bit-Download
Ein kostenloser und leistungsstarker IDE-Editor von Microsoft
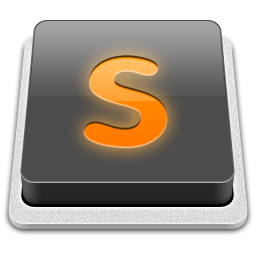
SublimeText3 Mac-Version
Codebearbeitungssoftware auf Gottesniveau (SublimeText3)

Sicherer Prüfungsbrowser
Safe Exam Browser ist eine sichere Browserumgebung für die sichere Teilnahme an Online-Prüfungen. Diese Software verwandelt jeden Computer in einen sicheren Arbeitsplatz. Es kontrolliert den Zugriff auf alle Dienstprogramme und verhindert, dass Schüler nicht autorisierte Ressourcen nutzen.
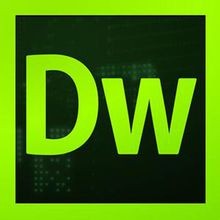
Dreamweaver CS6
Visuelle Webentwicklungstools
