session
我没事的时候写的自主实现Session功能的类,基于文件方式存储Session数据,测试基本通过,还比较好玩,实际应用没有意义,只不过是学习Session是如何实现的。
一般基于文件存储Session数据效率不是很高,毕竟跟磁盘IO是有关系的,如果需要多台服务器共享数据,可以考虑使用NFS来存储数据,如果需要比较快的速度,可以考虑使用共享内存(shm)来保存数据,直接把Session数据存储路径指定为/dev/shm,这样磁盘IO会提高不少,不过shm空间比较小,一般Linux下是60多M,所以不可能保存太多数据。
//=======================================
// 程序:File-Based Session Class
// 功能:基于文件存储的 Session 功能类
// 作者: heiyeluren
// 博客: http://blog.csdn.net/heiyeshuwu
// 时间: 2006-12-22
//=======================================
/**
* 类名: FileSession Class
* 功能: 自主实现基于文件存储的 Session 功能
* 描述: 这个类就是实现Session的功能, 基本上是通过设置客户端的Cookie来保存SessionID,
* 然后把用户的数据保存在服务器端,最后通过Cookie中的Session Id来确定一个数据是否是用户的,
* 然后进行相应的数据操作, 目前的缺点是没有垃圾收集功能
*
* 本方式适合保存在普通文件、共享内存(SHM)、NFS服务器等基于文件存储的方式,推荐保存在共享
* 内存当中,因为共享内存存取效率比较高,但是空间比较小,重启后就销毁了
*/
class FileSession
{
var $sessId = '';
var $sessSavePath = '/tmp/';
var $isCreatePath = true;
var $sessExpireTime = '';
var $sessFilePrefix = 'sess_';
var $cookieName = '__SessHandler';
/**
* 构造函数
*
* @param bool $isInit - 是否实例化对象的时候启动Session
*/
function FileSession($isInit = false){
if ($isInit){
$this->start();
}
}
//-------------------------
// 外部方法
//-------------------------
/**
* 启动Session操作
*
* @param int $expireTime - Session失效时间,缺省是0,当浏览器关闭的时候失效, 该值单位是秒
*/
function start($expireTime = 0){
$sessId = $_COOKIE[$this->cookieName];
if (!$sessId){
if (!$this->_checkSavePath()){
$this->_showMessage('Session save path '. $this->sessSavePath .' not or create path failed');
}
$this->sessId = $this->_getId();
$this->sessExpireTime = ($expireTime > 0) ? time() + $expireTime : 0;
setcookie($this->cookieName, $this->sessId, $this->sessExpireTime, "/", '');
$_SESSION = array();
$this->_writeFile();
} else {
$this->sessId = $sessId;
$_SESSION = unserialize($this->_getFile($sessId));
}
}
/**
* 判断某个Session变量是否注册
*
* @param string $varName -
* @return bool 存在返回true, 不存在返回false
*/
function is_registered($varName){
if (!isset($_SESSION[$varName])){
return false;
}
return true;
}
/**
* 注册一个Session变量
*
* @param string $varName - 需要注册成Session的变量名
* @param mixed $varValue - 注册成Session变量的值
* @return bool - 该变量名已经存在返回false, 注册成功返回true
*/
function register($varName, $varValue){
if (isset($_SESSION[$varName])){
return false;
}
$_SESSION[$varName] = $varValue;
$this->_writeFile();
return true;
}
/**
* 销毁一个已注册的Session变量
*
* @param string $varName - 需要销毁的Session变量名
* @return bool 销毁成功返回true
*/
function unregister($varName){
unset($_SESSION[$varName]);
$this->_writeFile();
return true;
}
/**
* 销毁所有已经注册的Session变量
*
* @return 销毁成功返回true
*/
function destroy(){
$_SESSION = array();
$this->_writeFile();
return true;
}
/**
* 获取一个已注册的Session变量值
*
* @param string $varName - Session变量的名称
* @return mixed - 不存在的变量返回false, 存在变量返回变量值
*/
function get($varName){
if (!isset($_SESSION[$varName])){
return false;
}
return $_SESSION[$varName];
}
/**
* 获取所有Session变量
*
* @return array - 返回所有已注册的Session变量值
*/
function getAll(){
return $_SESSION;
}
/**
* 获取当前的Session ID
*
* @return string 获取的SessionID
*/
function getSid(){
return $this->sessId;
}
/**
* 获取服务器端保存的Session数据的路径
*
* @return string 保存Session的路径
*/
function getSavePath(){
return $this->sessSavePath;
}
/**
* 设置保存Session数据的路径
*
* @param string $savePath - 需要保存Session数据的绝对路径
*/
function setSavePath($savePath){
$this->sessSavePath = $savePath;
}
//-------------------------
// 内部接口
//-------------------------
/**
* 生成一个Session ID
*
* @return string 返回一个32位的Session ID
*/
function _getId(){
return md5(uniqid(microtime()));
}
/**
* 检查保存Session数据的路径是否存在
*
* @return bool 成功返回true
*/
function _checkSavePath(){
if (file_exists($this->sessSavePath)){
return true;
}
if (!$this->isCreatePath){
return false;
}
if (!@mkdir($this->sessSavePath)){
$this->_showMessage('Failed: Session cache path '. $this->sessSavePath .'is not exists, create failed');
}
@chmod($this->sessSavePath, 0777);
return true;
}
/**
* 获取Session文件中的数据
*
* @param string $sessId - 需要获取Session数据的SessionId
* @return unknown
*/
function _getFile($sessId = ''){
$sessId = ($sessId == '') ? $this->sessId : $sessId;
$sessFile = $this->sessSavePath . $this->sessFilePrefix . $sessId;
if (!file_exists($sessFile)){
$this->_showMessage('Failed: Session file '. $sessFile .' not exists');
}
return file_get_contents($sessFile);
}
/**
* 把当前的Session数据写入到数据文件
*
* @param string $sessId - Session ID
* @return 成功返回true
*/
function _writeFile($sessId = ''){
$sessId = ($sessId == '') ? $this->sessId : $sessId;
$sessFile = $this->sessSavePath . $this->sessFilePrefix . $sessId;
$sessStr = serialize($_SESSION);
if (!$fp = @fopen($sessFile, "w+")){
$this->_showMessage('Failed: Open session save file '. $sessFile .' failed');
}
if (!@fwrite($fp, $sessStr)){
$this->_showMessage('Failed: Write session data to '. $sessFile .' failed');
}
@fclose($fp);
return true;
}
/**
* 显示提示信息
*
* @param string $strMessage - 需要显示的信息内容
* @param bool $isFailed - 是否是失败信息, 缺省是true
*/
function _showMessage($strMessage, $isFailed = true){
if ($isFailed){
exit($strMessage);
}
echo $strMessage;
}
}
?>

Heiße KI -Werkzeuge

Undresser.AI Undress
KI-gestützte App zum Erstellen realistischer Aktfotos

AI Clothes Remover
Online-KI-Tool zum Entfernen von Kleidung aus Fotos.

Undress AI Tool
Ausziehbilder kostenlos

Clothoff.io
KI-Kleiderentferner

AI Hentai Generator
Erstellen Sie kostenlos Ai Hentai.

Heißer Artikel

Heiße Werkzeuge

MantisBT
Mantis ist ein einfach zu implementierendes webbasiertes Tool zur Fehlerverfolgung, das die Fehlerverfolgung von Produkten unterstützen soll. Es erfordert PHP, MySQL und einen Webserver. Schauen Sie sich unsere Demo- und Hosting-Services an.
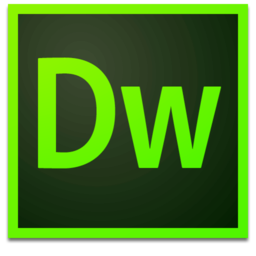
Dreamweaver Mac
Visuelle Webentwicklungstools
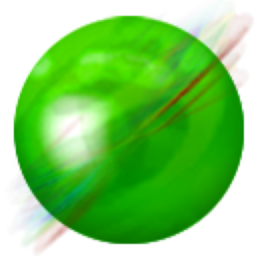
ZendStudio 13.5.1 Mac
Leistungsstarke integrierte PHP-Entwicklungsumgebung

MinGW – Minimalistisches GNU für Windows
Dieses Projekt wird derzeit auf osdn.net/projects/mingw migriert. Sie können uns dort weiterhin folgen. MinGW: Eine native Windows-Portierung der GNU Compiler Collection (GCC), frei verteilbare Importbibliotheken und Header-Dateien zum Erstellen nativer Windows-Anwendungen, einschließlich Erweiterungen der MSVC-Laufzeit zur Unterstützung der C99-Funktionalität. Die gesamte MinGW-Software kann auf 64-Bit-Windows-Plattformen ausgeführt werden.

SublimeText3 chinesische Version
Chinesische Version, sehr einfach zu bedienen