A linear data structure for storing the elements in a non-contiguous manner is called a LinkedList in which the pointers are used to link the elements in the linked list with each other and System.Collections.Generic namespace consists of the LinkedList class in C# from which the elements can be removed or can be inserted into in a very quick manner implementing a classic linked list and the allocation of each object is separate in linked list and there is no necessity of copying of entire collection to perform certain operations on the linked list.
Syntax:
The syntax of LinkedList class in C# is as follows:
LinkedList<Type> linkedlist_name = new LinkedList <Type>();
Where Type represents the type of linked list.
Working of LinkedList Class in C#
- There are nodes present in the linked list and every node consists of two parts namely data field and a link to the node that comes next in the linked list.
- The type of every node in the linked list is LinkedListNode type.
- A node can be removed from the linked list and can be inserted back to the same linked list or cab be inserted to another linked list and hence there is no extra allocation on the heap.
- Inserting the elements into a linked list, removing the elements from the linked list, and obtaining the property of count which is an internal property maintained by the liked list are all O(1) operations.
- Enumerators are supported by the linked list class as it is a general-purpose linked list.
- Nothing that makes the linked list inconsistent is supported by the linked list.
- If the linked list is doubly linked list, then each node has two pointers, one pointing to the previous node in the list and the other one pointing to the next node in the list.
Constructors of LinkedList Class
There are several constructors in the LinkedList class in C#. They are:
-
LinkedList(): A new instance of the linked list class is initialized which is empty.
-
LinkedList(IEnumerable): A new instance of the linked list class is initialized which is taken from the specified implementation of IEnumerable whose capacity is enough to accumulate all the copied elements.
-
LinkedList(SerializationInfo, StreamingContext): A new instance of the linked list class is initialized which can be serialized with the serializationInfo and StreamingContext specified as parameters.
Methods of LinkedList Class in C#
There are several methods in the LinkedList class in C#. They are:
-
AddAfter: A value or new node is added after an already present node in the linked list using the AddAfter method.
-
AddFirst: A value or new node is added at the beginning of the linked list using the AddFirst method.
-
AddBefore: A value or new node is added before an already present node in the linked list using the AddBefore method.
-
AddLast: A value or new node is added at the end of the linked list using the AddLast method.
-
Remove(LinkedListNode): A node specified as a parameter will be removed from the linked list using Remove(LinkedListNode) method.
-
RemoveFirst(): A node at the beginning of the linked list will be removed from the linked list using RemoveFirst() method.
-
Remove(T): The first occurrence of the value specified as a parameter in the linked list will be removed from the linked list using the Remove(T) method.
-
RemoveLast(): A node at the end of the linked list will be removed from the linked list using the RemoveLast() method.
-
Clear(): All the nodes from the linked list will be removed using the Clear() method.
-
Find(T): The value specified as the parameter present in the very first node will be identified by using the Find(T) method.
-
Contains(T): We can use the Contains(T) method to find out if a value is present in the linked list or not.
-
ToString(): A string representing the current object is returned by using the ToString() method.
-
CopyTo(T[], Int32): The whole linked list is copied to an array which is one dimensional and is compatible with the linked list and the linked list begins at the index specified in the array to be copied to using CopyTo(T[], Int32) method.
-
OnDeserialization(Object): After the completion of deserialization, an event of deserialization is raised and the ISerializable interface is implemented using OnDeserialization(Object) method.
-
Equals(Object): If the object specified as the parameter is equal to the current object or not is identified using Equals(Object) method.
-
FindLast(T): The value specified as the parameter present in the last node will be identified by using FindLast(T) method.
-
MemberwiseClone(): A shallow copy of the current object is created using MemeberwiseClone() method.
-
GetEnumerator(): An enumerator is returned using GetEnumerator() method and the returned enumerator loops through the linked list.
-
GetType(): The type of the current instance is returned using GetType() method.
-
GetHashCode(): The GetHashCode() method is the hash function by default.
-
GetObjectData(SerializationInfo, StreamingContext): The data which is necessary to make the linked list serializable is returned by using GetObjectData(SerializationInfo, StreamingContext) method along with implementing the ISerializable interface.
Example of LinkedList Class in C#
C# program to demonstrate AddLast() method, Remove(LinkedListNode) method, Remove(T) method, RemoveFirst() method, RemoveLast() method and Clear() method in Linked List class:
Code:
using System;
using System.Collections.Generic;
//a class called program is defined
public class program
{
// Main Method is called
static public void Main()
{
//a new linked list is created
LinkedList<String> list = new LinkedList<String>();
//AddLast() method is used to add the elements to the newly created linked list
list.AddLast("Karnataka");
list.AddLast("Mumbai");
list.AddLast("Pune");
list.AddLast("Hyderabad");
list.AddLast("Chennai");
list.AddLast("Delhi");
Console.WriteLine("The states in India are:");
//Using foreach loop to display the elements of the newly created linked list
foreach(string places in list)
{
Console.WriteLine(places);
}
Console.WriteLine("The places after using Remove(LinkedListNode) method are:");
//using Remove(LinkedListNode) method to remove a node from the linked list
list.Remove(list.First);
foreach(string place in list)
{
Console.WriteLine(place);
}
Console.WriteLine("The places after using Remove(T) method are:");
//using Remove(T) method to remove a node from the linked list
list.Remove("Chennai");
foreach(string plac in list)
{
Console.WriteLine(plac);
}
Console.WriteLine("The places after using RemoveFirst() method are:");
//using RemoveFirst() method to remove the first node from the linked list
list.RemoveFirst();
foreach(string pla in list)
{
Console.WriteLine(pla);
}
Console.WriteLine("The places after using RemoveLast() method are:");
//using RemoveLast() method to remove the last node from the linked list
list.RemoveLast();
foreach(string pl in list)
{
Console.WriteLine(pl);
}
//using Clear() method to remove all the nodes from the linked list
list.Clear();
Console.WriteLine("The count of places after using Clear() method is: {0}",
list.Count);
}
}
The output of the above program is as shown in the snapshot below:
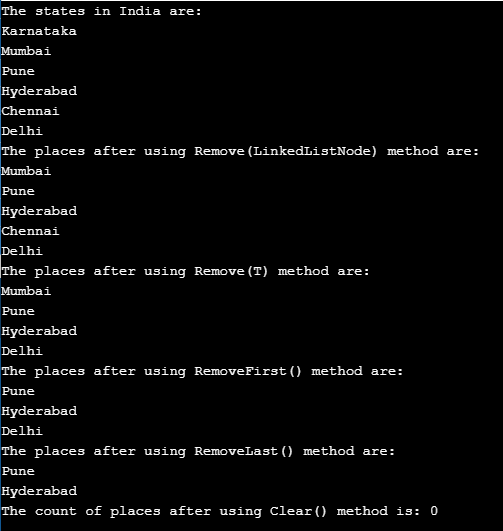
In the above program, a class called program is defined. Then the main method is called. Then a new linked list is created. Then AddLast() method is used to add the elements to the newly created linked list. Then foreach loop is used to display the elements of the newly created linked list. Then Remove(LinkedListNode) method is used to remove a node from the linked list. Then Remove(T) method is used to remove a node from the linked list. Then RemoveFirst() method is used to remove the first node from the linked list. Then RemoveLast() method is used to remove the last node from the linked list. Then Clear() method is used to remove all the nodes from the linked list. The output of the program is shown in the snapshot above.
Das obige ist der detaillierte Inhalt vonC# LinkedList. Für weitere Informationen folgen Sie bitte anderen verwandten Artikeln auf der PHP chinesischen Website!
Stellungnahme:Der Inhalt dieses Artikels wird freiwillig von Internetnutzern beigesteuert und das Urheberrecht liegt beim ursprünglichen Autor. Diese Website übernimmt keine entsprechende rechtliche Verantwortung. Wenn Sie Inhalte finden, bei denen der Verdacht eines Plagiats oder einer Rechtsverletzung besteht, wenden Sie sich bitte an admin@php.cn