Bootstrap modal box
Bootstrap Modal plug-in
Modal is a subform that covers the parent form. Typically, the purpose is to display content from a separate source that can have some interaction without leaving the parent form. Subforms provide information, interaction, and more.
If you want to reference the functionality of the plugin separately, then you need to reference modal.js. Alternatively, as mentioned in the Bootstrap plugin overview chapter, you can reference bootstrap.js or the minified version of bootstrap.min.js.
Usage
You can switch the hidden content of the modal plug-in:
Through the data attribute: in Set the attribute data-toggle="modal" on the controller element (such as a button or link), and also set data-target="#identifier" or href="# identifier" to specify the specific modal box to switch (with id="identifier").
Via JavaScript: Using this technique, you can call a modal with id="identifier" with a simple line of JavaScript:
$('#identifier').modal(options)
Instance
A static modal window instance, as shown in the following example:
Instance
<!DOCTYPE html> <html> <head> <title>Bootstrap 实例 - 模态框(Modal)插件</title> <link rel="stylesheet" href="http://apps.bdimg.com/libs/bootstrap/3.3.0/css/bootstrap.min.css"> <script src="http://apps.bdimg.com/libs/jquery/2.1.1/jquery.min.js"></script> <script src="http://apps.bdimg.com/libs/bootstrap/3.3.0/js/bootstrap.min.js"></script> </head> <body> <h2>创建模态框(Modal)</h2> <!-- 按钮触发模态框 --> <button class="btn btn-primary btn-lg" data-toggle="modal" data-target="#myModal"> 开始演示模态框 </button> <!-- 模态框(Modal) --> <div class="modal fade" id="myModal" tabindex="-1" role="dialog" aria-labelledby="myModalLabel" aria-hidden="true"> <div class="modal-dialog"> <div class="modal-content"> <div class="modal-header"> <button type="button" class="close" data-dismiss="modal" aria-hidden="true"> × </button> <h4 class="modal-title" id="myModalLabel"> 模态框(Modal)标题 </h4> </div> <div class="modal-body"> 在这里添加一些文本 </div> <div class="modal-footer"> <button type="button" class="btn btn-default" data-dismiss="modal">关闭 </button> <button type="button" class="btn btn-primary"> 提交更改 </button> </div> </div><!-- /.modal-content --> </div><!-- /.modal --> </div> </body> </html>
Run Instance»
Click the "Run Instance" button to view the online instance
The result is as follows:
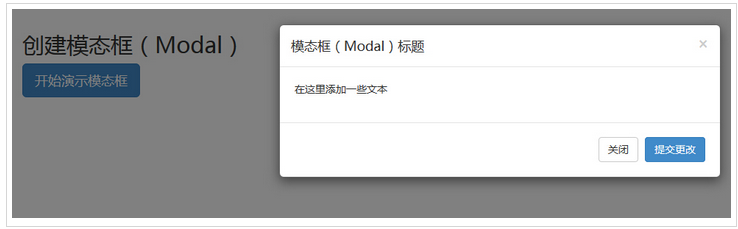
Code explanation:
To use a modal window, you need to have some kind of trigger. You can use buttons or links. Here we are using buttons.
If you look carefully at the above code, you will find that in the <button> tag, data-target="#myModal" is where you want to The target of the modal loaded on the page. You can create multiple modals on the page and then create different triggers for each modal. Now, obviously, you can't load multiple modules at the same time, but you can create multiple modules on the page that load at different times.
You need to pay attention to two points in the modal box:
The first is .modal, use To identify the content of <div> as a modal box.
The second is .fade class. When the modal is toggled, it causes the content to fade in and out.
aria-labelledby="myModalLabel", this attribute refers to the title of the modal box.
Attributearia-hidden="true" Used to keep the modal window invisible until the trigger is fired (such as clicking on the relevant button) .
<div class="modal-header">, modal-header is a class that defines the style for the head of the modal window.
class="close", close is a CSS class used to style the close button of a modal window.
data-dismiss="modal", is a custom HTML5 data attribute. Here it is used to close the modal window.
class="modal-body", is a CSS class of Bootstrap CSS, used to set styles for the body of modal windows.
class="modal-footer", is a CSS class of Bootstrap CSS, used to set styles for the bottom of modal windows.
data-toggle="modal", HTML5 custom data attribute data-toggle is used to open a modal window.
Options
There are some options that can be used to customize the look and feel of the modal window (Modal Window). They are passed through the data attribute or JavaScript. The following table lists these options:
Option Name | Type/Default Value | Data Property Name | Description |
---|---|---|---|
backdrop | boolean or string 'static' Default value: true | data-backdrop | Specify a static background when The modal does not close when the user clicks outside the modal. |
keyboard | boolean Default value: true | data-keyboard | When pressed When the escape key is pressed, the modal box is closed. When set to false, the key press has no effect. |
show | boolean Default value: true | data-show | When initialized Show modal box. |
remote | path Default value: false | data-remote | Use jQuery .load method, injects content into the main body of the modal box. If you add an href with a valid URL, the content within it will be loaded. As shown in the following example: <a data-toggle="modal" href="remote.html" data-target="#modal">请点击我</a> |
Methods
Here are some useful methods that can be used with modal().
Method | Description | Instance |
---|---|---|
Options: .modal(options) | Activate the content as a modal box. Accepts an optional options object. | $('#identifier').modal({ keyboard: false }) |
Toggle: .modal('toggle') | Manually switch the modal box. | $('#identifier').modal('toggle') |
Show: .modal('show') | Manually open the modal box. | $('#identifier').modal('show') |
Hide: .modal('hide') | Manually hide the modal box. | $('#identifier').modal('hide') |
Example
The following example demonstrates the use of the method:
Example
<!DOCTYPE html> <html> <head> <title>Bootstrap 实例 - 模态框(Modal)插件方法</title> <link rel="stylesheet" href="http://apps.bdimg.com/libs/bootstrap/3.3.0/css/bootstrap.min.css"> <script src="http://apps.bdimg.com/libs/jquery/2.1.1/jquery.min.js"></script> <script src="http://apps.bdimg.com/libs/bootstrap/3.3.0/js/bootstrap.min.js"></script> </head> <body> <h2>模态框(Modal)插件方法</h2> <!-- 按钮触发模态框 --> <button class="btn btn-primary btn-lg" data-toggle="modal" data-target="#myModal"> 开始演示模态框 </button> <!-- 模态框(Modal) --> <div class="modal fade" id="myModal" tabindex="-1" role="dialog" aria-labelledby="myModalLabel" aria-hidden="true"> <div class="modal-dialog"> <div class="modal-content"> <div class="modal-header"> <button type="button" class="close" data-dismiss="modal" aria-hidden="true">× </button> <h4 class="modal-title" id="myModalLabel"> 模态框(Modal)标题 </h4> </div> <div class="modal-body"> 按下 ESC 按钮退出。 </div> <div class="modal-footer"> <button type="button" class="btn btn-default" data-dismiss="modal">关闭 </button> <button type="button" class="btn btn-primary"> 提交更改 </button> </div> </div><!-- /.modal-content --> </div><!-- /.modal-dialog --> </div><!-- /.modal --> <script> $(function () { $('#myModal').modal({ keyboard: true })}); </script> </body> </html>
Run Instance»
Click the "Run Instance" button to view the online instance
The result is as follows:
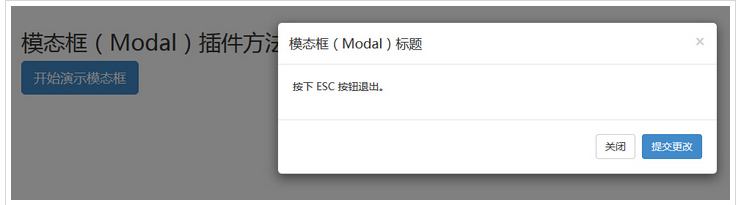
Just click the ESC key and the modal window will exit.
Events
The following table lists the events used in modal boxes. These events can be used as hooks in functions.
Event | Description | Instance |
---|---|---|
show.bs.modal | Triggered after calling the show method. | $('#identifier').on('show.bs.modal', function () { // 执行一些动作... }) |
shown.bs.modal | Fires when the modal becomes visible to the user (will wait for CSS transition effects to complete). | $('#identifier').on('shown.bs.modal', function () { // 执行一些动作... }) |
hide.bs.modal | Fired when the hide instance method is called. | $('#identifier').on('hide.bs.modal', function () { // 执行一些动作... }) |
hidden.bs.modal | Fires when the modal is completely hidden from the user. | $('#identifier').on('hidden.bs.modal', function () { // 执行一些动作... }) |
Example
The following example demonstrates the usage of events:
Example
<!DOCTYPE html> <html> <head> <title>Bootstrap 实例 - 模态框(Modal)插件事件</title> <link rel="stylesheet" href="http://apps.bdimg.com/libs/bootstrap/3.3.0/css/bootstrap.min.css"> <script src="http://apps.bdimg.com/libs/jquery/2.1.1/jquery.min.js"></script> <script src="http://apps.bdimg.com/libs/bootstrap/3.3.0/js/bootstrap.min.js"></script> </head> <body> <h2>模态框(Modal)插件事件</h2> <!-- 按钮触发模态框 --> <button class="btn btn-primary btn-lg" data-toggle="modal" data-target="#myModal"> 开始演示模态框 </button> <!-- 模态框(Modal) --> <div class="modal fade" id="myModal" tabindex="-1" role="dialog" aria-labelledby="myModalLabel" aria-hidden="true"> <div class="modal-dialog"> <div class="modal-content"> <div class="modal-header"> <button type="button" class="close" data-dismiss="modal" aria-hidden="true">× </button> <h4 class="modal-title" id="myModalLabel"> 模态框(Modal)标题 </h4> </div> <div class="modal-body"> 点击关闭按钮检查事件功能。 </div> <div class="modal-footer"> <button type="button" class="btn btn-default" data-dismiss="modal"> 关闭 </button> <button type="button" class="btn btn-primary"> 提交更改 </button> </div> </div><!-- /.modal-content --> </div><!-- /.modal-dialog --> </div><!-- /.modal --> <script> $(function () { $('#myModal').modal('hide')})}); </script> <script> $(function () { $('#myModal').on('hide.bs.modal', function () { alert('嘿,我听说您喜欢模态框...');}) }); </script> </body> </html>
Run Example»
Click the "Run Instance" button to view the online instance
The results are as follows:
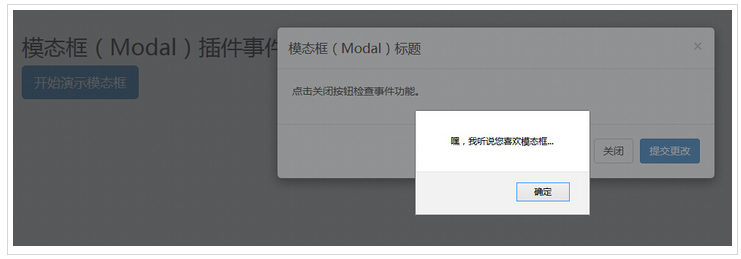
As shown in the above example, if you When the Close button is clicked, which is the hide event, a warning message will be displayed.