代码
indx.php
<?php
session_start();
if(isset($_SESSION['user'])) {
$user = unserialize($_SESSION['user']);
}
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>登录界面</title>
<style>
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
a{
text-decoration: none;
width: 100%;
height: 100%;
}
header {
background-color: #2C3E50;
padding: 0.5em 1em;
display: flex;
height: 12vh;
}
header .title {
font-weight:300;
font-style:normal;
font-size: 35pt;
color: aliceblue;
letter-spacing: 1px;
text-shadow: 1px 1px 1px #D0D0D0;
margin-top: 15px;
}
@import url("https://fonts.googleapis.com/css?family=Source+Sans+Pro");
figure {
width: 200px;
height: 60px;
cursor: pointer;
perspective: 500px;
-webkit-perspective: 500px;
margin-left: auto;
margin-top: 10px;
}
figure div {
height: 100%;
transform-style: preserve-3d;
-webkit-transform-style: preserve-3d;
transition: 0.25s;
-webkit-transition: 0.25s;
}
figure:hover div {
transform: rotateX(-90deg);
}
span {
width: 100%;
height: 100%;
position: absolute;
box-sizing: border-box;
border: 5px solid #fff;
font-family: "Source Sans Pro", sans-serif;
line-height: 50px;
font-size: 17pt;
text-align: center;
text-transform: uppercase;
}
span:nth-child(1) {
color: #fff;
transform: translate3d(0, 0, 30px);
-webkit-transform: translate3d(0, 0, 30px);
}
span:nth-child(2) {
color: #1c175d;
background: #fff;
transform: rotateX(90deg) translate3d(0, 0, 30px);
-webkit-transform: rotateX(90deg) translate3d(0, 0, 30px);
}
</style>
</head>
<body>
<header>
<?php if(isset($user)):?>
<h2 class="title"><?= $user['email']?></h2>
<?php else:?>
<h2 class="title">请先登录</h2>
<?php endif?>
<figure>
<div>
<span>Hover Me</span>
<?php if(isset($user)):?>
<span><a href="" id="logout">退出</a></span>
<?php else :?>
<span><a href="login.php">登录</a></span>
<?php endif?>
</div>
</figure>
</header>
<script>
document.querySelector('#logout').addEventListener('click',function(event){
if(confirm('是否退出?')){
event.preventDefault();
location.assign('handle.php?action=logout')
}
});
</script>
</body>
</html>
login.php
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>登录界面</title>
<style>
form {
display: inline-block;
}
a{
text-decoration: none;
}
</style>
</head>
<body>
<form action="handle.php?action=login" method="POST">
<fieldset>
<legend>用户登录</legend>
<p>
<label for="">邮 箱:</label>
<input type="emali" name="email">
</p>
<p>
<label for="">密 码:</label>
<input type="password" name="psw1" id="psw1">
</p>
<p>
<button>登录</button>
</p>
<p>
<a href="register.php">如果没有账号,请先注册>></a>
</p>
</fieldset>
</form>
</body>
</html>
register.php
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>新用户注册</title>
<style>
form {
display: inline-block;
}
</style>
</head>
<body>
<form action="handle.php?action=register" method="POST">
<fieldset>
<legend>用户注册</legend>
<p>
<label for="">邮 箱:</label>
<input type="email" name="email" id="email">
</p>
<p>
<label for="">密 码:</label>
<input type="password" name="psw2" id="psw2" placeholder="密码必须大于8位">
</p>
<p>
<label for="">确认密码:</label>
<input type="password" name="" id="psw3" placeholder="再次输入密码">
</p>
<button>提交</button>
</fieldset>
</form>
<script>
document.querySelector('button').addEventListener('click',function(event){
let psw1 = document.querySelector('#psw2');
let psw2 = document.querySelector('#psw3');
// let form = document.querySelector('form');
if(psw1.value.length > 8) {
if(psw1.value !== psw2.value){
event.preventDefault();
alert("两次密码输入不一致");
location.assign('register.php');
}
}else{
event.preventDefault();
alert("密码长度不够");
}
})
</script>
</body>
</html>
handle.php
<?php
// 开启会话
session_start();
// 链接数据库
$db = new PDO('mysql:dbname=phpedu', 'root', 'root');
$stmt = $db->prepare('SELECT * FROM `login`');
$stmt->execute();
$users = $stmt->fetchAll(PDO::FETCH_ASSOC);
// print_r($users);
// print_r($_GET);
$action = $_GET['action'];
switch(strtolower($action)){
// 登录
case 'login':
// 验证数据是否是以POST请求发送
if($_SERVER['REQUEST_METHOD']==='POST'){
extract($_POST);
$result = array_filter($users,function($user) use ($email,$psw1){
return $user['email']===$email && $user['password']=== md5($psw1);
});
if(count($result)===1){
$_SESSION['user']=serialize(array_pop($result));
exit('<script>alert("验证通过");location.href="index.php"</script>');
}else{
exit('<script>alert("邮箱或者验证码错误");location.href="login.php"</script>');
}
}else{
die("请求错误");
}
break;
// 退出
case 'logout':
if(isset($_SESSION['user'])){
session_destroy();
exit('<script>alert("退出成功");location.assign("index.php")</script>');
}
break;
// 注册
case 'register':
// 获取新用户的信息
$email = $_POST['email'];
$password =md5($_POST['psw2']);
$register_time=time();
// 查重
$sql = 'SELECT `email` FROM `login`';
$stmt=$db->prepare($sql);
$stmt->execute();
$staffs = $stmt->fetchAll(PDO::FETCH_ASSOC);
$flag=true;
printf('<pre>%s</pre>', print_r($staffs, true));
foreach($staffs as $staff){
if(in_array($email,$staff)){
$flag = false;
exit('<script>alert("邮箱已被注册");location.href="register.php"</script>');
break;
}
}
if($flag){
// 添加到数据库中
$sql = 'INSERT `login` SET `email`=?,`password`=?,`register_time`=? ';
$stmt= $db->prepare($sql);
$stmt->execute([$email,$password,$register_time]);
if($stmt->rowCount()>0){
exit('<script>alert("注册成功");location.assign("login.php")</script>');
}else{
exit('<script>alert("注册失败");location.assign("register.php")</script>');
}
}
break;
}
演示
1、先在数据库中插入一个已有数据
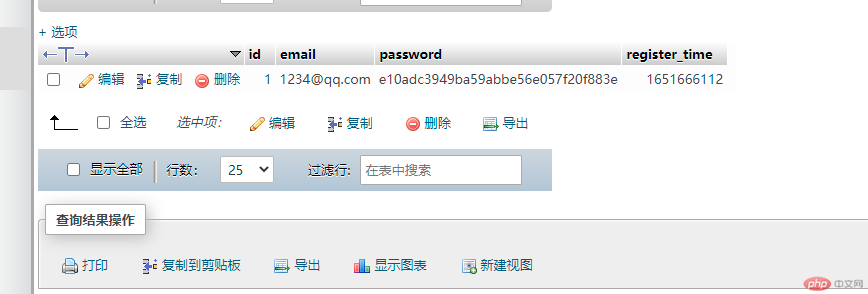
2、登录操作
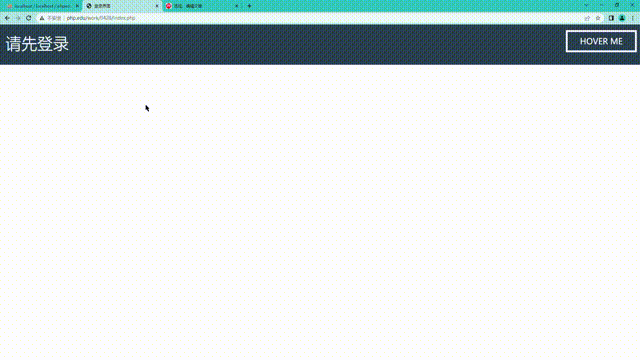
3、注册操作
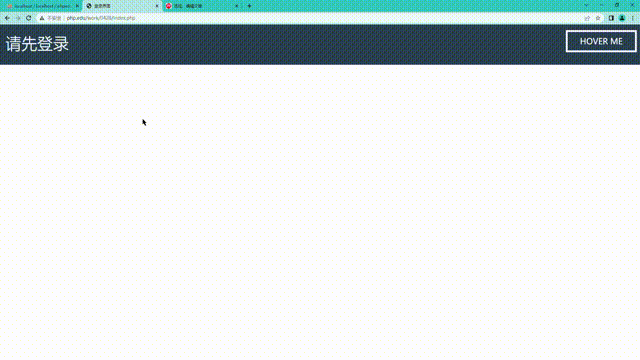

4、注册失败(密码长度不够)
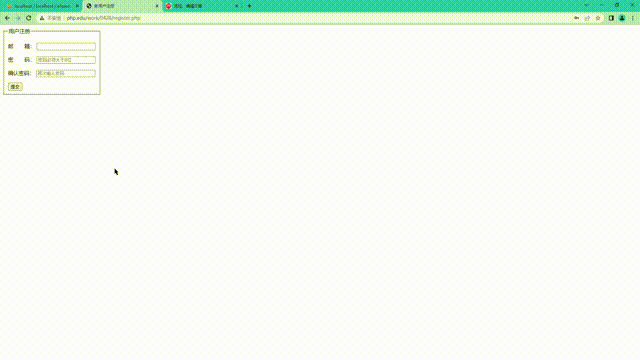
5、注册失败(邮箱已被注册)
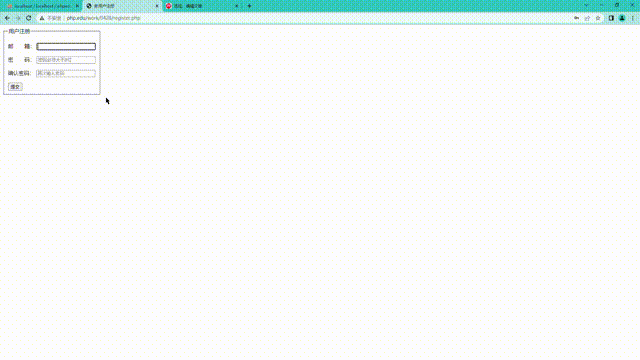