类的属性和方法
代码:
<?php
// 类声明
class Person
{
private $name;
private $age;
// 静态属性
private static $number = 0;
// 自定义方法
public function setClass($name,$age){
$this->name = $name;
$this->age = $age;
}
// 魔术方法
public function __construct(string $name,int $age)
{
$this->name = $name;
$this->age = $age;
self::$number++;
}
// 自定义方法:显示函数
public function Print(){
echo '姓名: '.$this->name.' 年龄:'.$this->age;
}
// 静态方法
public static function PeoPrint(){
echo '<br>'.'记录人数:'.self::$number.'<br>';
}
}
// 创建一个人类
$people1=new Person('xyz',20);
$people1->Print();
// 查看人数
Person::PeoPrint();
// 修改年龄和姓名;
$people1->setClass('111',10);
$people1->Print();
Person::PeoPrint();
// 多创建几个
$people2= new Person('222',1);
$people3= new Person('333',11);
$people4= new Person('444',13);
$people5= new Person('555',14);
// 查看记录人数:
Person::PeoPrint();
效果:
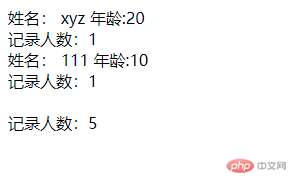
类的扩展/抽象/最终
<?php
// 定义一个抽象父类
abstract class Person{
// protect能被继承
protected $name;
protected $age;
protected $gender;
// 构造函数
public function __construct(string $name,int $age,string $gender)
{
$this->name=$name;
$this->age=$age;
$this->gender=$gender;
}
// 打印函数
public function PPrint(){
echo '姓名: '.$this->name.'<br>'.'年龄: '.$this->age.'<br>'.'性别: '.$this->gender.'<br>';
}
// 抽象方法: 只有方法名,参数列表,没有具体实现(大括号)
// 抽象方法被继承时,一定要重写
abstract protected function hr();
}
// 学生类,定义为最终类,防止继承
final class Student extends Person
{
private $class;
private $score;
// 构造函数
public function __construct(string $name,int $age,string $gender,string $class,int $score){
parent::__construct($name,$age,$gender);
$this->class = $class;
$this->score = $score;
}
public function PPrint()
{
parent::PPrint();
echo '<br>'.'课程: '.$this->class.'<br>'.'分数: '.$this->score.'<br>';
}
public function hr(){
echo '<hr>';
}
}
$student1=new Student('xyz',12,'男','数学',120);
$student1->PPrint();
$student1->hr();
效果:
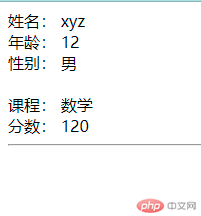
接口
<?php
// 接口: 大号的抽象类
// 接口的所有成员,必须是抽象
// interface: 声明接口
// implements: 实现接口
interface iPerson{
const Nation='CHINA';
// 必须是抽象,必须是public
public function PPrint();
public function hr();
}
abstract class Person implements iPerson{
// protect能被继承
protected $name;
protected $age;
protected $gender;
// 构造函数
public function __construct(string $name,int $age,string $gender)
{
$this->name=$name;
$this->age=$age;
$this->gender=$gender;
}
// 打印函数
public function PPrint(){
echo '姓名: '.$this->name.'<br>'.'年龄: '.$this->age.'<br>'.'性别: '.$this->gender.'<br>';
}
}
final class Student extends Person
{
private $class;
private $score;
// 构造函数
public function __construct(string $name,int $age,string $gender,string $class,int $score){
parent::__construct($name,$age,$gender);
$this->class = $class;
$this->score = $score;
}
public function PPrint()
{
parent::PPrint();
echo '<br>'.'课程: '.$this->class.'<br>'.'分数: '.$this->score.'<br>';
}
public function hr(){
echo '<hr>';
}
}
$student1=new Student('xyz',12,'男','数学',100);
$student1->PPrint();
$student1->hr();
效果:
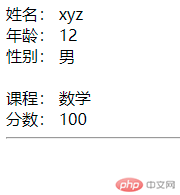