显示用户登录代码 login.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>用户登录</title>
<style>
.login {
width: 480px;
margin: 0 auto;
padding: 20px;
background-color: lightblue;
}
form {
display: grid;
gap: 0.5rem;
}
form * {
margin: 5px 20px;
}
h2 {
text-align: center;
}
</style>
</head>
<body>
<div class="login">
<h2>用户登录</h2>
<form action="">
<fieldset>
<div>
<label for="account">账 户:</label>
<input
type="text"
id="account"
name="account"
required
placeholder="请输入用户名"
/>
</div>
<div>
<label for="password">密 码:</label>
<input
type="password"
id="password"
name="password"
required
placeholder="请输入密码"
/>
</div>
</fieldset>
<button type="button" onclick="check(this.form)">登录</button>
</form>
</div>
<script>
function check(thisForm) {
const account = thisForm.account.value.trim();
const password = thisForm.password.value.trim();
//加密成JSON数据
const user = JSON.stringify({ account: account, password: password });
fetch("check.php", { method: "post", body: user })
.then((response) => response.json())
.then((json) => {
if (json.status != 0) {
alert(json.msg);
return false;
}
alert(json.msg);
setTimeout(() => {
window.location.href = "showuser.php";
}, 1000);
});
}
</script>
</body>
</html>
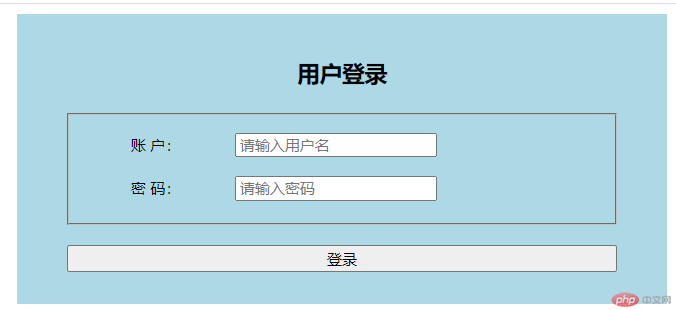
<?php
require_once "auto.php";
session_start();
header("Content-Type:application/json");
$data=trim(file_get_contents('php://input'));
// json 转为数组
$user=json_decode($data,true);
//
$res=UserContr::login($user['account'],$user['password']);
if($res['stutas']==0){
setcookie('id',1);
$_SESSION['account']=$user['account'];
}
echo json_encode($res);
- UserContr.php文件UserContr类中的用户登录代码
//用户登录
public static function login($account,$password){
$pdo =CreatePDO::Create();
$sql="SELECT * FROM `php_user` WHERE account='".$account."'";
$pre=$pdo->prepare($sql);
$exec=$pre->execute();
$arr=$pre->fetch();
if(empty($arr)){
$res=['status'=>1,'msg'=>'用户不存在'];
}elseif($arr['password']!=md5($password)){
$res=['status'=>2,'msg'=>'密码不正确'];
}else{
$res=['status'=>0,'msg'=>'登录成功'];
}
return $res;
}
<?php
session_start();
if(empty($_COOKIE['id'])){
echo "<script>window.location.href='login.html'</script>";
exit;
}
$account=$_SESSION['account'];
?>
<div class="header">
<div><span><?=$account?></span> <a href="destroy.php">注销</a>
</div>
</div>
<style>
*{
margin: 0;
padding: 0;
}
.header{
height: 30px;
background-color: purple;
}
.header div{
width: 15em;
margin:5px 0px ;
position: fixed;
right: 2px;
color: lightsalmon;
}
a{
text-decoration: none;
}
.header a{
margin:auto 1rem;
color: #eee;
}
</style>
- 在showuser.php文件中引入header.php文件。
<?php require_once "header.php" ?>
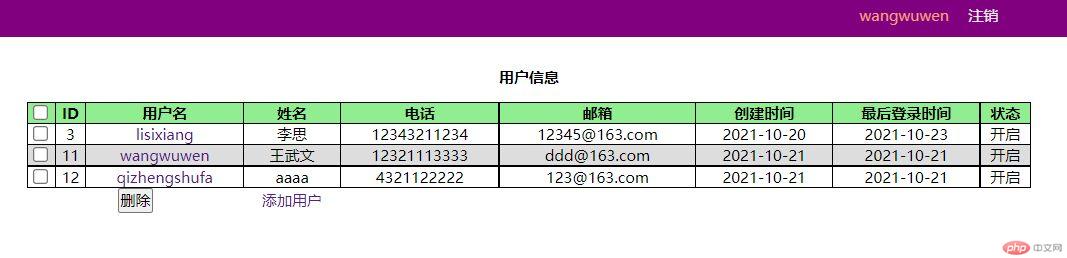
<?php
setcookie('id',1,time());
$_SESSION['account']=null;
echo '<script>alert("退出登录");location.href="login.html"</script>';