使用函数封装一个动态表格,自行写5个系统函数
1.使用函数封装一个动态表格
<?php
$car = ['品牌','车型','价格','排量'];
$arr = [
[
'brand' => 'BMW',
'model' => '330运动曜夜版',
'price' => '380000元',
'displacement' =>'2.0T高功'
],
[
'brand' => 'BMW',
'model' => '530豪华版',
'price' => '480000元',
'displacement' =>'2.0T高功'
],
[
'brand' => 'Audi',
'model' => 'A8行政版',
'price' => '980000元',
'displacement' =>'3.0T高功'
],
[
'brand' => 'Audi',
'model' => 'A4运动版',
'price' => '280000元',
'displacement' =>'2.0T低功'
],
];
function table(array $car, array $arr,$color){
$table = '<table border="1" width="500px" bgcolor="'.$color.'">';
$table .='<thead>';
$table .= '<tr>';
foreach($car as $value){
$table .='<th>'.$value.'</th>';
}
$table .= '</tr>';
$table .= '</thead>';
$table .= '<tbody>';
foreach($arr as $key1 => $value1){
$table .= '<tr>';
$table .= '<td>'.$value1['brand'].'</td>';
$table .= '<td>'.$value1['model'].'</td>';
$table .= '<td>'.$value1['price'].'</td>';
$table .= '<td>'.$value1['displacement'].'</td>';
$table .= '</tr>';
}
$table .= '</tbody>';
$table .='</table>';
return $table;
}
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<?php echo table($car,$arr,'#ccc') ?>
</body>
</html>
代码运行
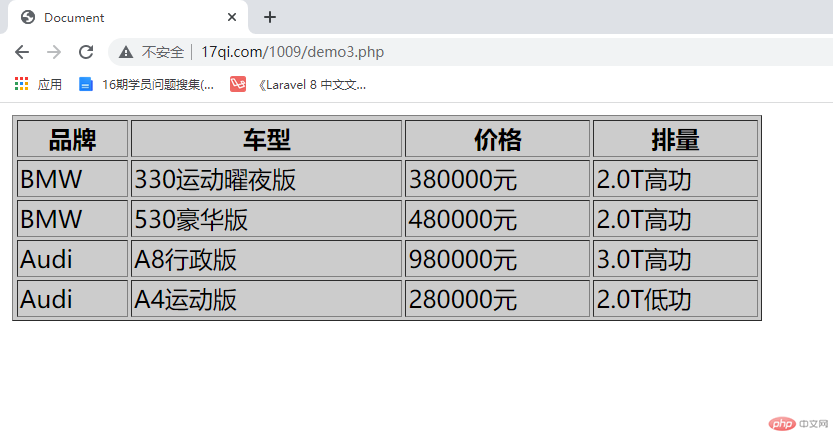
2.自行演示5个字符串系统函数
<?php
// 字符串函数
// 1.ucfirst(string $str): string,将字符串的首字母变成大写
$name = 'peter';
echo ucfirst($name);
echo '<hr>';
// 2.parse_str — 将字符串解析成多个变量
$str = 'name=peter&age=28';
parse_str($str,$str1);
echo $str1['name'].'<br>';
echo $str1['age'];
echo '<hr>';
// 3.str_split 将字符串且切割成数组
$str1 = 'abcdefghigk';
print_r(str_split($str1));
echo "<br>";
$str2 = 'abcdefghit';
//第二个参数,是将字符串取几个字符,拆分到数组中
print_r(str_split($str2,3));
echo '<hr>';
// 4.str_repeat 重复一个字符串,第二个参数是重复的次数,如果是0,
// 则返回一个空数组;
$str3 = '^_^';
echo str_repeat($str3,10);
echo '<hr>';
// 5.strpos 查找字符串首次出现的位置,第二个参数是查询哪个字符
//返回的是第二个参数,首次出现的位置,
$str4 = 'This is a isset';
echo strpos($str4,'is');
演示结果
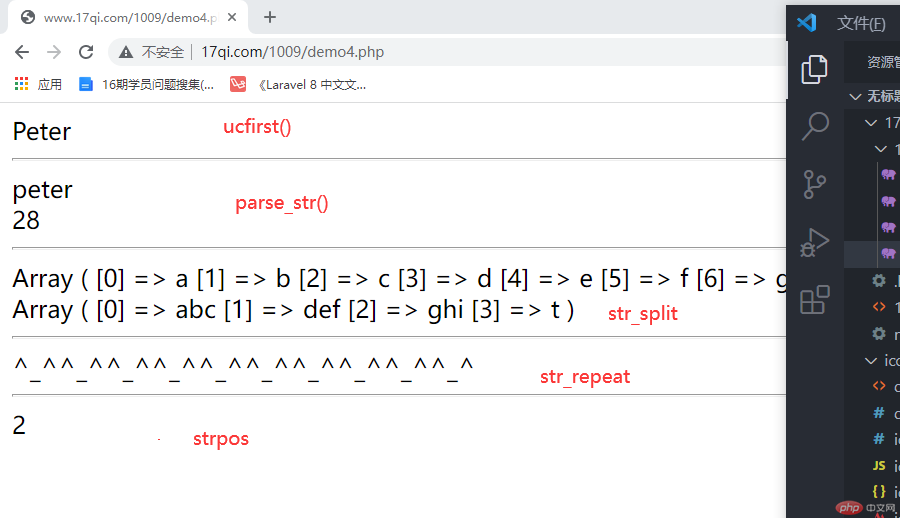