留言板:
样式代码:
<body>
<h2>留言板:</h2>
<!-- 引入jQyery库 -->
<script src="https://cdn.bootcdn.net/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<!-- jQuery中的dom操作,实现前面写的留言本 -->
<label><input type="text" id="lyb" /></label>
<ol class="list"></ol>
<script>
//留言板添加数据
$("#lyb").on("keydown", function (ev) {
// 非空回车判断
if ($(this).val().length > 0) {
if (ev.key == "Enter") {
let lyb = `<li>${$(
this
).val()} [<a href="javascript:;" onclick="$(this).parent().remove();">删除</a>]</li>`;
// 添加新的留言在前面
$("ol.list").prepend(lyb);
// 清空上一条留言
$(this).val(null);
}
}
});
</script>
</body>
效果预览:
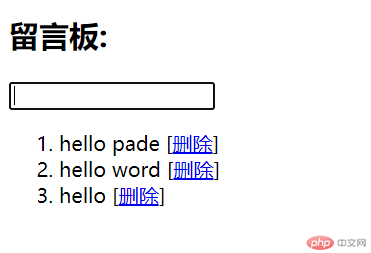
演示$.get、$.post:
样式代码:
<body style="display: grid; gap: 2em; padding: 2em">
<button class="get">1. $.get(): 请求数据</button>
<button class="post">2. $.post(): 请求数据</button>
<script src="https://cdn.bootcdn.net/ajax/libs/jquery/3.5.1/jquery.js"></script>
<script>
// 1. $.get(请求url,查询参数,成功回调)
$(".get").click(function (ev) {
// $.get("users.php?id=1", function (data) {
$.get("users.php", { id: 2 }, function (data) {
// console.log(data);
// console.log(ev.target);
$(ev.target).after("<div></div>").next().html(data);
});
});
// 2. post()
$(".post").click(function (ev) {
$.post("users.php", { id: 3 }, function (data) {
console.log(data);
$(ev.target).after("<div></div>").next().html(data);
});
});
</script>
</body>
php代码:
<?php
// 二维数组来模拟数据表的查询结果
$users = [
['id' => 1, 'name' => '老头子', 'age' => 66],
['id' => 2, 'name' => '小菇凉', 'age' => 18],
['id' => 3, 'name' => '小伙子', 'age' => 20],
];
// $_REQUEST: 相当于 $_GET + $_POST + $_COOKIE 三合一
if (in_array($_REQUEST['id'], array_column($users, 'id'))) {
foreach ($users as $user) {
if ($user['id'] == $_REQUEST['id']) {
// vprintf(输出模板, 数组表示的参数)
vprintf('%s: %s %s岁',$user);
// 以下语句配合$.getJSON()调用,其它请求时请注释掉
// echo json_encode($user);
}
}
} else {
die('<span style="color:red">没找到</span>');
}
效果预览:
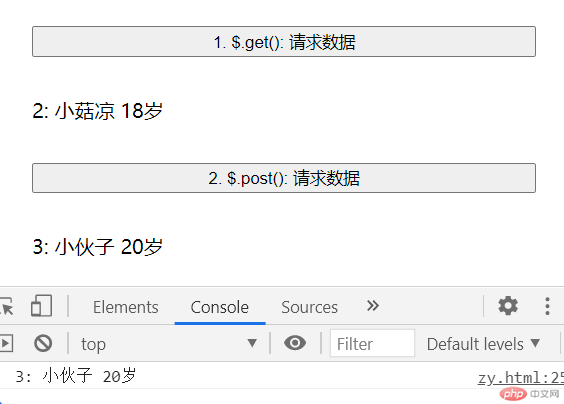
$ajax实现jsonp中跨域请求:
<body style="display: grid; gap: 2em; padding: 2em">
<button class="jsonp">3. $.ajax():JSONP: 跨域请求数据</button>
<script src="https://cdn.bootcdn.net/ajax/libs/jquery/3.5.1/jquery.js"></script>
<script>
$(".jsonp").click(function (ev) {
$.ajax({
type: "get",
url: "http://world.io/test.php?id=2&jsonp=?",
dataType: "jsonp",
// 告诉跨域访问的服务器需要返回的函数名称
// jsonpCallback: "show",
success: function (data) {
console.log(data);
$("button:last-of-type")
.after("<div>")
.next()
.html(`${data.name} [${data.email}]`);
},
});
});
</script>
</body>
php代码:
<?php
header('content-type:text/html;charset=utf-8');
// 获取回调名称
$callback = $_GET['jsonp'];
$id = $_GET['id'];
// 模拟接口数据
$users = [
0=>'{"name":"朱老师", "email":"peter@php.cn"}',
1=>'{"name":"西门老师", "email":"xm@php.cn"}',
2=>'{"name":"猪哥", "email":"pig@php.cn"}'
];
if (array_key_exists(($id-1), $users)) {
$user = $users[$id-1];
}
// echo $user;
// 动态生成handle()的调用
echo $callback . '(' . $user . ')';
效果预览:
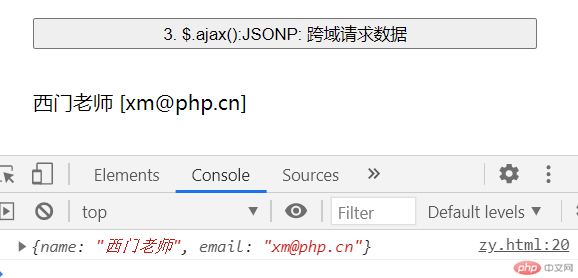