// 使用class类实现随机数生成并排列及简易计算
class Rndnumber {
constructor(arr,num) {
this.arr = arr
this.num = num
}
getRandomArrayValue() {
const newArr = []
for(let i = 0; i < this.num; i ++){
// Math.random() 默认取值范围 0 - 1
// (this.arr.length - 1) 当前数组长度 - 1
const random = Math.ceil((Math.random() * (this.arr.length - 1)));
// splice截取数组内容 返回的是一个截取的数组
// 取出截取数组的数据 存入新创建的数组
newArr.push(this.arr.splice(random,1)[0])
}
return newArr
}
static arr = [0,1,2,3,4,5,6,7,8,9]
}
class Combination extends Rndnumber{
constructor(arr,whether) {
super(arr);
this.whether = whether;
}
//数组排列方法
arraySort(){
if(this.whether === 0){
return this.arr;
}
return this.arr.sort()
}
}
class Calculator {
constructor(arr,isMethod) {
this.arr = arr;
this.isMethod = isMethod
}
calculationData(){
// 保存this 防止this指向被改变
const _this = this;
// 返回处理后的数组内容
return this.arr.reduce((last,first)=>{
switch (true){
case _this.isMethod === '+' :
return last + first;
case _this.isMethod === '-' :
return last - first;
default :
return last * first;
}
})
}
}
//生成n个随机数
const rndnumber = new Rndnumber(Rndnumber.arr,5)
const randomArr = rndnumber.getRandomArrayValue();
console.log('随机取数组内容 返回一个数组')
console.log(randomArr)
console.log('---------------')
//数组是否排列 1===排列 0===原值返回
const combination = new Combination(randomArr,1)
//调用子类Combination的数组排列方法
const sortArr = combination.arraySort();
console.log('数组由小到大进行排列如下')
console.log(sortArr)
/**
* 计算数组内的数据
* (isMethod值默认为*)
* + : 为数组内数据相加
* - :数组内数据相减
* * :数组内数组相乘
*/
const calculator = new Calculator(sortArr,"-")
const operation = calculator.calculationData();
console.log('上述数组内容数据计算结果如下')
console.log(operation)
运行图
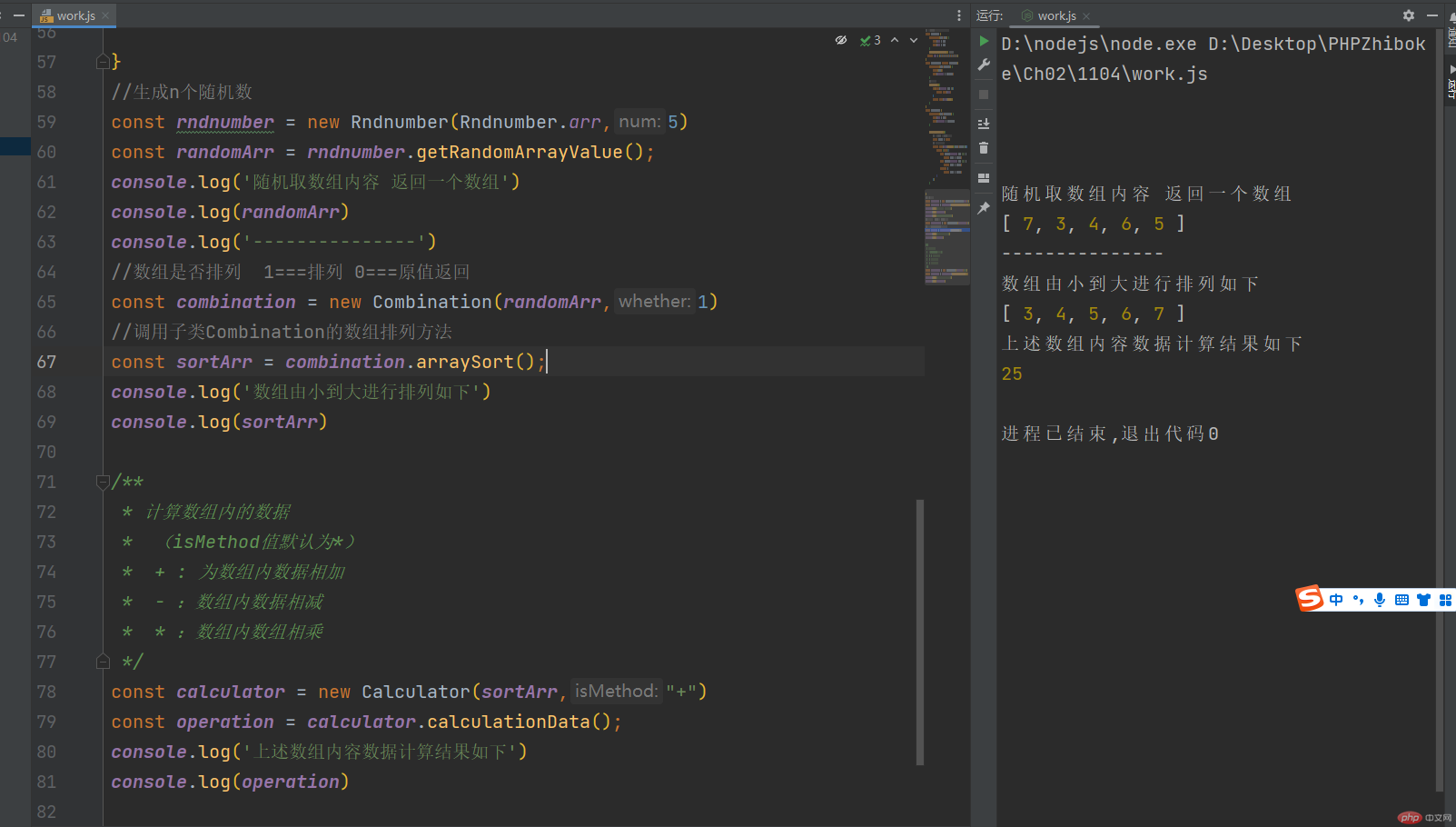