浮动:
浮动只限于水平方向
浮动元素脱离了文档流,后面的元素会上移填充它原来的空间
浮动元素不会影响到它前面的元素的布局,只会影响到后面的元素排列
任何元素(包括行内元素)浮动后,都具备了块级元素的特征
父元素计算高度的时候,会忽略内部的浮动元素(父级高度的塌陷)
可以通过伪元素或创建BFC的方式这两种来修改父级高度塌陷问题
css:
<style>
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
.box {
padding: 1em;
border: 1px solid #000;
background-color: lightcyan;
}
.box .desc a {
width: 10em;
height: 10em;
background-color: violet;
float: left;
}
</style>
<body>
<div class="box">
<div class="desc">
<a href="">这是一个浮动</a>
</div>
</div>
</body>
示例:
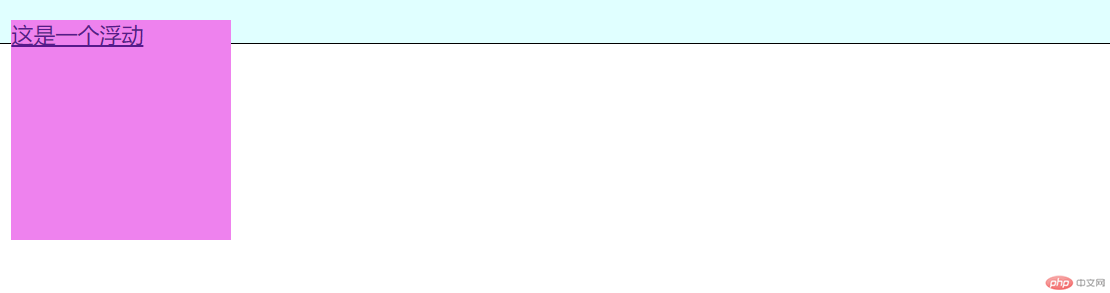
修改方式:
<style>
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
.box {
padding: 1em;
border: 1px solid #000;
background-color: lightcyan;
}
.box .desc a {
width: 10em;
height: 10em;
background-color: violet;
float: left;
}
//伪元素
.box:after{
content: '';
display: block;
clear: both;
}
//创建BFC
.box .desc{
overflow: hidden;
}
</style>
示例:
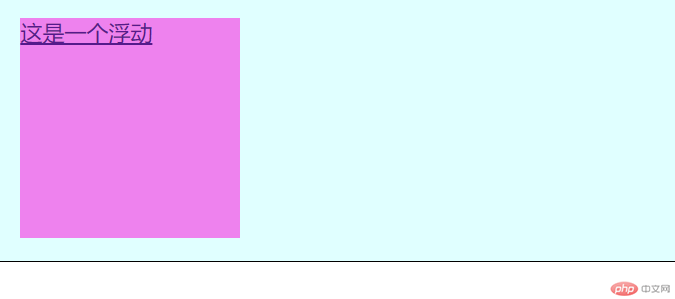
定位类型:
静态定位static 这是默认的
相对定位relative
绝对定位absolue
固定定位fixed
<style>
.box {
width: 10em;
height: 10em;
border: 5px solid #000;
margin: 3em auto;
}
.box h2 {
border: 1px solid #000;
background-color: yellowgreen;
//相对定位
position: relative;
top: 1em;
left: 1em;
}
//将h2改为绝对定位
.box h2 {
//绝对定位会脱离文档流
position: absolute;
}
.box {
// 转为定位元素,它内部的元素就相对于它进行绝对定位
position: relative;
}
.box h2{
top: 0;
left: 0;
right: 0;
bottom: 0;
width: 4em;
height: 2em;
margin: auto;
}
html{
min-height: 100em;
}
//使用固定定位使用h2不动
.box h2{
position: fixed;
}
</style>
<body>
<div class="box">
<h2>二维码</h2>
</div>
</body>
示例:
<title>模态框</title>
<link rel="stylesheet" href="style.css" />
</head>
<body>
//页眉
<header>
<h2>点击右侧登录按钮</h2>
<button>登录</button>
</header>
//模态框
<div class="modal">
//蒙板:用来盖住后面的内容,使它半透明
<div class="modal-backdrop"></div>
//主体
<div class="modal-body">
<button class="close">关闭</button>
<form action="" method="POST">
<table>
<caption>
用户登录
</caption>
<tbody>
<tr>
<td><label for="email">用户名:</label></td>
<td><input type="email" name="email" id="email" /></td>
</tr>
<tr>
<td><label for="password">密码:</label></td>
<td><input type="password" name="password" id="password" /></td>
</tr>
<tr>
<td></td>
<td><button>登录</button></td>
</tr>
</tbody>
</table>
</form>
</div>
</div>
<script src="modal.js"></script>
</body>
CSS代码:
//页眉
header {
background-color: #ccc;
padding: .5em 2em;
overflow: hidden;
}
header h2{
float: left;
}
header button {
float: right;
width: 10em;
height: 2.5em;
}
header button:hover{
cursor: pointer;
background-color: #fff;
}
//模态框
//蒙板
.modal .modal-backdrop {
background-color: rgb(0,0,0,0.5);
position: fixed;
top:0;
left:0;
right:0;
bottom: 0;
}
.modal .modal-body {
padding: 1em;
min-width: 20em;
border: 1px solid #000;
background: linear-gradient(to right, blue,red,#bbbbbb,violet,lightcyan, #fff,#ccc);
//固定定位
position: fixed;
top: 5em;
left: 30em;
right: 30em;
}
.modal form table {
width: 80%;
}
.modal form table caption {
font-weight: bold;
margin-bottom: 1em;
}
.modal form table td {
padding: 0.5em;
}
.modal form table td:first-of-type {
width: 5em;
}
.modal form table input {
width: 20em;
height: 2em;
}
.modal form table button {
width: 20em;
height: 2.5em;
}
//定位父级
.modal-body {
position: relative;
}
.modal .close {
position: absolute;
width: 4em;
height: 2em;
top: 1em;
right: 1em;
}
.modal .close:hover {
cursor: pointer;
background-color: red;
color: white;
}
//页面初始化时,模态框应该隐藏
.modal {
display: none;
}
js代码:
const btn = document.querySelector('header button');
const modal = document.querySelector('.modal');
const close = document.querySelector('.close');
btn.addEventListener('click', setModal, false);
close.addEventListener('click', setModal, false);
function setModal(ev) {
ev.preventDefault();
let status = window.getComputedStyle(modal, null).getPropertyValue('display');
modal.style.display = status === 'none' ? 'block' : 'none';
}
示例:
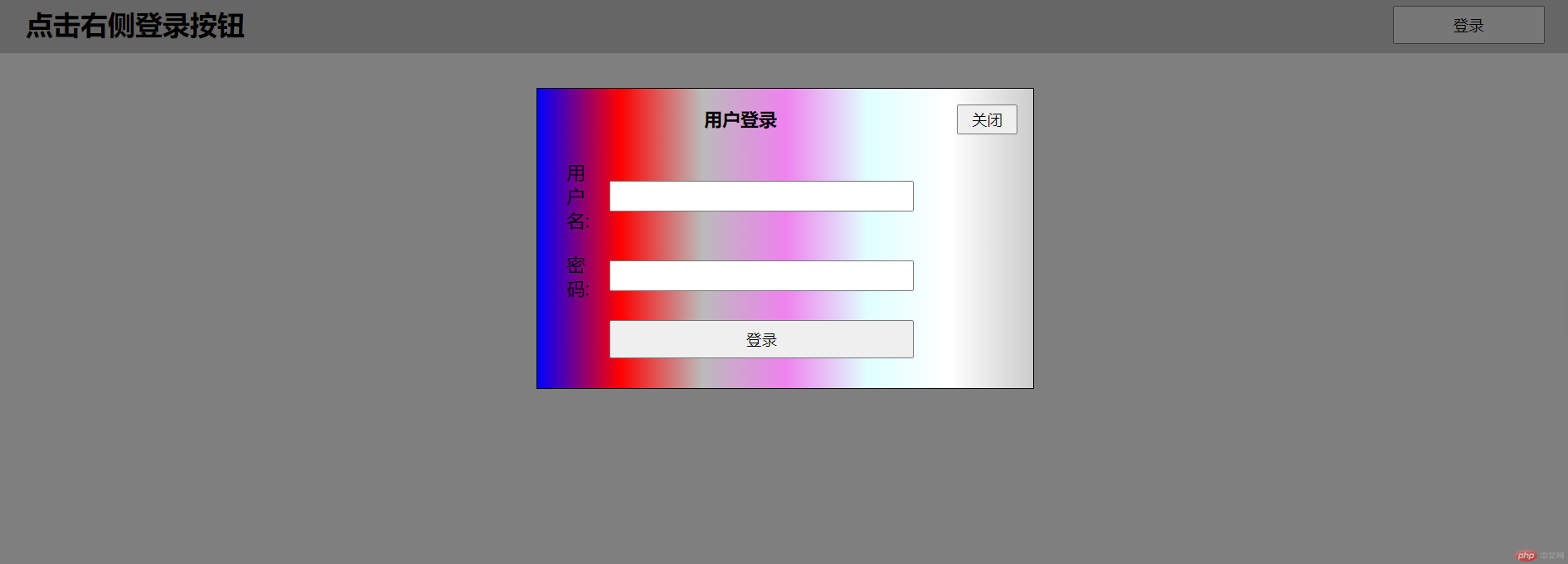