PHP分页
1.连接数据库
<?php
//连接数据库
$pdo = new PDO('mysql:host=localhost;dbname=tp5','root','wang1111');
//设置默认的提取模式
$pdo->setAttribute(PDO::ATTR_DEFAULT_FETCH_MODE,PDO::FETCH_ASSOC);
2.分页原理
<?php
//分页原理
//SELECT * FROM `user` 每页的数量 显示的偏移量
//每页显示的数量 LIMIT n
//显示的偏移量:从哪个索引开始 OFFSET m
//偏移量 = (页码-1)* 每页的显示数量
require 'connect.php';
//获取分页数据,一定要知道的二个数据
//1.每页显示的数量
$num = 5;
//2.当前页码
$page = $_GET['p'] ?? 1;
//3.计算每一页的第一条记录的显示偏移量
$offset = ($page-1)*$num;
//4.获取分页数据
$sql = "SELECT * FROM `user` LIMIT {$num} OFFSET {$offset}";
$users = $pdo->query($sql)->fetchAll();
//print_r($users);
//计算总页数
//ceil()向上取整
//总页数 = ceil(记录总数/每页的记录数)
$sql = "SELECT CEIL(COUNT(`id`)/{$num}) AS `total` FROM `user`";
$pages = $pdo->query($sql)->fetch()['total'];
//print_r($res);
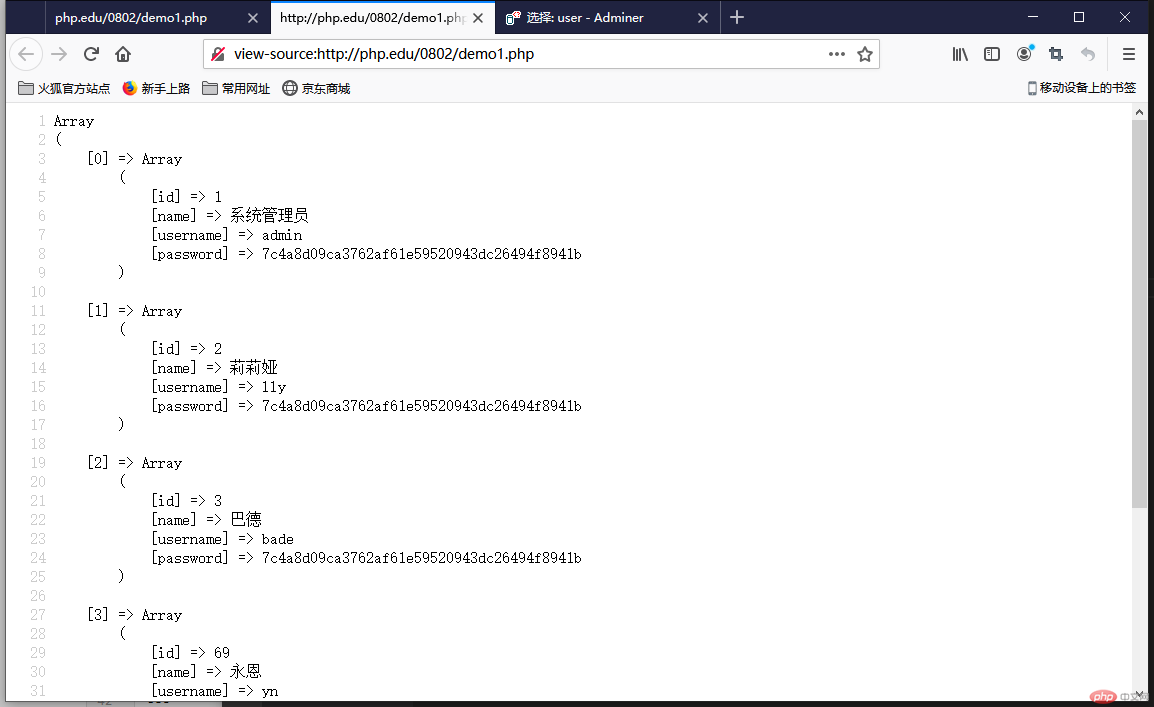
3.显示分页
<?php require 'demo1.php'; ?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>数据展示</title>
<style>
body{
padding:0;
margin:0;
box-sizing:border-box;
display:flex;
justify-content:center;
}
thead > tr{
background:#77DDFF;
}
table{
text-align:center;
width:800px;
}
caption{
padding:10px 0;
}
.delete{
color:#E63F00;
}
p{
display:flex;
justify-content:center;
}
p > a{
display:block;
border:1px solid #000;
text-align:center;
text-decoration:none;
width:20px;
height:20px;
color:#000;
margin:0 5px;
}
p > a:hover{
background:#000;
color:#fff;
border:1px solid #fff;
}
.active{
background:#000;
color:#fff;
border:1px solid #fff;
}
.shouye{
width:40px;
height:20px;
font-size:15px;
}
.shang{
width:60px;
height:20px;
font-size:15px;
}
</style>
</head>
<body>
<div>
<table border="1px" cellspacing="0" cellpadding="10px">
<caption>用户信息表</caption>
<thead>
<tr>
<td>id</td>
<td>name</td>
<td>username</td>
<td>操作</td>
</tr>
</thead>
<tbody>
<?php foreach ($users as $user): ?>
<tr>
<td><?=$user['id']?></td>
<td><?php echo $user['name'] ?></td>
<td><?php echo $user['username'] ?></td>
<td>
<button onclick="location.href='handle.php?action=edit&id=<?=$user['id']?>'">编辑</button>
<button class="delete" onclick="del(<?=$user['id']?>)">删除</button></td>
</tr>
<?php endforeach; ?>
</tbody>
</table>
<!-- 分页条 -->
<p>
<?php
$previous = $page-1;
if($previous < 1) $previous = 1;
?>
<?php if($page != 1): ?>
<a href="<?=$_SERVER['PHP_SELF']?>?p=1" class="shouye">首页</a>
<a href="<?=$_SERVER['PHP_SELF']?>?p=<?=$previous?>" class="shang">上一页</a>
<?php endif; ?>
<!-- ********************************************************** -->
<?php
if($page<=10):
for($i=1;$i<=10;$i++):
?>
<?php
$jump = sprintf('%s?p=%s',$_SERVER['PHP_SELF'],$i );
//当前页码与当前页相等的时候
$active = ($i == $page) ? 'active' : null;
?>
<!-- 将类名变量化 -->
<a href="<?=$jump?>" class="<?=$active?>"><?=$i?></a>
<?php
endfor;
endif;
?>
<!-- ********************************************************** -->
<?php
//$twenty = ceil($page/10)*10;
//$ten = floor($page);
$a = $page + 5;
$b = $page -4;
$c = $pages - 10;
if($page>10 && $page < $c):
for($i=$b;$i<=$a;$i++):
?>
<?php
$jump = sprintf('%s?p=%s',$_SERVER['PHP_SELF'],$i );
//当前页码与当前页相等的时候
$active = ($i == $page) ? 'active' : null;
?>
<!-- 将类名变量化 -->
<a href="<?=$jump?>" class="<?=$active?>"><?=$i?></a>
<?php
endfor;
endif;
?>
<!-- ********************************************************** -->
<?php
$c = $pages - 10;
if($page>=$c):
for($i=$c;$i<=$pages;$i++):
?>
<?php
$jump = sprintf('%s?p=%s',$_SERVER['PHP_SELF'],$i );
//当前页码与当前页相等的时候
$active = ($i == $page) ? 'active' : null;
?>
<!-- 将类名变量化 -->
<a href="<?=$jump?>" class="<?=$active?>"><?=$i?></a>
<?php
endfor;
endif;
?>
<!-- ********************************************************** -->
<?php
$next = $page+1;
if($next >= $pages) $next = $pages;
?>
<?php if($page < $pages): ?>
<a href="<?=$_SERVER['PHP_SELF']?>?p=<?=$next?>" class="shang">下一页</a>
<a href="<?=$_SERVER['PHP_SELF']?>?p=<?=$pages?>" class="shouye">尾页</a>
<?php endif; ?>
</p>
<script>
//删除
function del(id){
str = 'handle.php?action=delete&id=';
str+=id;
if(confirm('是否删除?')){
location.href=str;
}
}
</script>
</div>
</body>
</html>
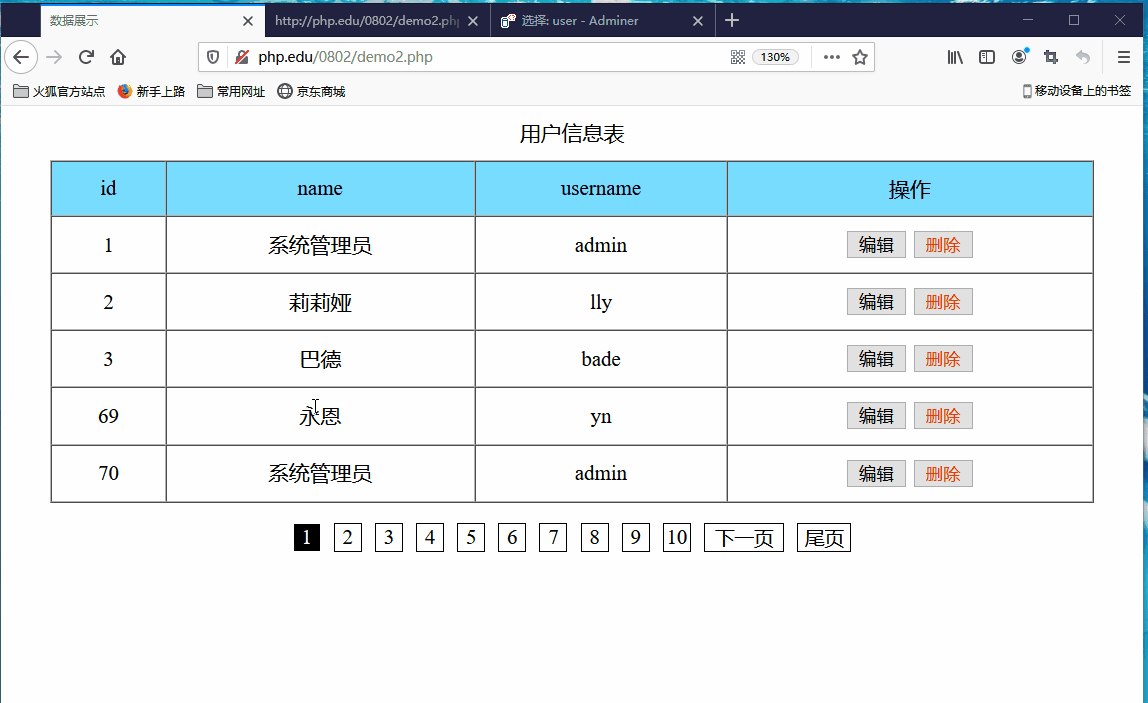
4.编辑与删除
<?php
//获取要被编辑的数据
$user = $pdo->query('SELECT * from `user` where `id` =' . $id)->fetch();
print_r($user);
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>用户编辑</title>
</head>
<body>
<form action="<?php echo $_SERVER['PHP_SELF'] . '?action=doedit&id='.$id?>" method="post">
<p>
<label for="name">用户名</label>
<input type="text" name="name" id="name" value="">
</p>
<p>
<label for="username">账号名</label>
<input type="text" name="username" id="username" value="">
</p>
<input type="hidden" name="id" value="">
<p>
<button>保存</button>
</p>
</form>
</body>
</html>
<?php
require 'connect.php';
//获取操作
$action = $_GET['action'];
$id= $_GET['id'];
switch ($action){
//编辑操作
case 'edit':
include 'edit.php';
break;
//2.执行编辑操作
case 'doedit':
//更新
$sql = 'UPDATE `user` SET `name`=?, `username`=? where `id`=?';
$stmt = $pdo->prepare($sql);
//新的数据在$_Post
if(!empty($_POST)){
$stmt->execute([$_POST['name'],$_POST['username'],$id]);
if($stmt->rowCount() == 1) echo '<script>alert("更新成功");location.href="demo2.php"</script>';
}
break;
//3.删除
case 'delete':
$sql = "DELETE FROM `user` where `id`=" . $id;
$res = $pdo->exec($sql);
if($res){
echo '<script>alert("删除成功");location.href="demo2.php"</script>';
}
break;
}
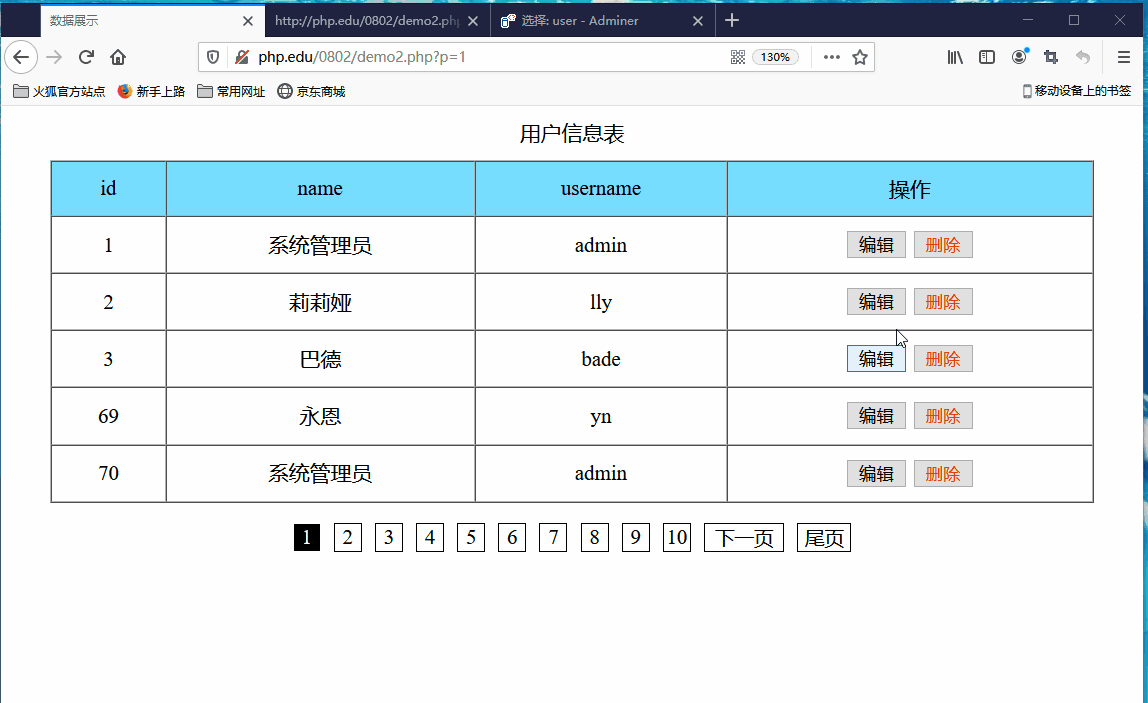
5.学习总结
- 分页要确定每页显示几条数据,当前页码,总记录数,总页数(向上取整),然后通过循环遍历数据库中的内容,编辑需要跳转到一个编辑表单中更改数据,然后保存到数据库中,报存成功,提示更新成功并跳转;删除直接执行删除语句,如果删除成功则提示删除成功并跳转。