教学内容
1. 跨域请求
1. CORS
CORS
: 跨域资源共享CSRF
: 跨站请求伪造
同源策略
多个页面的协议, 域名, 端口完全相同, 则认为他们遵循了”同源策略”
同源策略是一种安全机制
- 浏览器禁止通过脚本发起跨域请求, 如
XMLHttpRequest
,但是允许通知 html 标签属性跨域
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title></title>
</head>
<body>
<button>
跨域请求
</button>
<h2></h2>
<script>
// 获取按钮
var btn = document.querySelector("button");
// 给按钮添加点击事件
btn.addEventListener("click",function(){
// 创建监听对象
var xhr = new XMLHttpRequest();
// 监听请求
xhr.onreadystatechange =function(){
if(xhr.readyState === 4 && xhr.status === 200){
console.log(xhr.responseText);
// 将结果打印到h2元素中
document.querySelector("h2").innerHTML=xhr.responseText;
}
};
// 初始化请求参数
xhr.open("GET","http://localhost/0415php/0522/test1.php",true);
// 访问另一个服务器 http://localhost/0415php/0522/test1.php
// 发送请求
xhr.send(null);
},
false
);
</script>
</body>
</html>
- 其中,最重要的就是
Access-Control-Allow-Origin
,标识允许哪个域的请求。 Access-Control-Allow-Origin
有多种设置方法:- 设置
*
,不安全开放式请求。 - 指定域,例如
http://js.edu
- 动态设置为请求域
- 设置
<?php
header("Access-Control-Allow-Origin:http://js.edu");
echo "跨域测试";
flush();
" class="reference-link">- 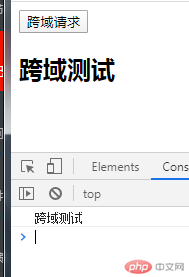
2. JSONP
- 同源策略禁止通过脚本发起跨域请求, 但是允许通过标签和属性发起一个跨域
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<button>
跨域请求
</button>
<script>
// 回调处理函数
function handle(jsonData){
console.log(jsonData);
// 转为JS对象
var data =JSON.parse(jsonData);
console.log(data);
// 将数据渲染到页面中
var ul = document.createElement("ul");
ul.innerHTML += "<li>" + data.title + "</li>";
ul.innerHTML += "<li>姓名:" + data.user.name + "</li>";
ul.innerHTML += "<li>邮箱:" + data.user.email + "</li>";
document.body.appendChild(ul);
}
// 获取按钮
var btn = document.querySelector("button");
// 添加事件
btn.addEventListener("click",function(){
// 动态发起jsonp的跨域请求
var script =document.createElement("script");
script.src="http://localhost/0415php/0522/test2.php?jsonp=handle&id=1";
document.head.appendChild(script);
},
false
);
</script
</body>
</html>
<?php
// json只支持utf-8
header('content-type:text/html;charset=utf-8');
// 获取前端传入的数据
$callback = $_GET['jsonp'];
$id=$_GET['id'];
// echo $callback;
// 用对象
$users = [
'{"name":"admin","email":"admin@qq.com"}',
'{"name":"ming","email":"admin@qq.com"}',
'{"name":"admin","email":"admin@qq.com"}',
];
// 检测是否存在
if(array_key_exists(($id-1),$users)){
$user =$users[$id-1];
}
$json ='{
"title": "用户表",
"user": '.$user.'
}';
echo $callback . '(' . json_encode($json) . ')';
节点
- 节点:html文档中所有的内容都是节点
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<p style="color: red;">php.cn</p>
<form action="1.php"></form>
<form action="2.php"></form>
<form action="3.php"></form>
<!-- <form action="3.php" id="login"></form> -->
<form action="3.php" name="login">
<input type="text" name="username" value="xxx" />
</form>
<script>
// 节点:html文档中所有的内容都是节点
// 节点树:DOM树
// 节点类型:元素、文本节点、属性节点、注释节点、文档节点
// doctype html
// console.log(document.doctype);
// html ---html
// console.log(document.documentElement);
// head
// console.log(document.head);
// body
// console.log(document.body);
// console.log(document.forms[0]);
// 通过ID拿
// console.log(document.getElementById("login"));
// console.log(document.forms.namedItem("login"));
console.log(document.forms.namedItem("login").username.value);
</script>
</body>
</html>
总结
- 了解跨域请求的方法、json只支持utf-8。
Access-Control-Allow-Origin
的使用- 了解获取HTML文档中的各个节点