学习总结
- 掌握了通过PHP中的PDO类实现与数据库的连接
- PDO可以加载多种类型的数据库引擎,如果更换数据库,只需要更改数据库连接参数中的dsn->type即可
- 可以把数据库的连接和增删改查封装在一个类中方便使用
1.自动加载一个PDO的连接类 DBconn.php
<?php
namespace compotents\conn
{
use Exception;
use PDO;
class DBconn
{
private $config = [];
protected $dbConn;
public function __construct($dbName = 'db_phpstudy',$userName = 'root',$passWord ='root')
{
$this ->config['type'] = 'mysql';
$this ->config['host'] = 'localhost';
$this ->config['dbName'] = $dbName;
$this ->config['charset'] = 'utf8';
$this ->config['port'] = '3306';
$this ->config['userName'] = $userName;
$this ->config['passWord'] = $passWord;
$this ->connect();
}
public function connect()
{
//拆分数组,键名当做变量名,值当做变量的值,拆分成数据库连接的变量
extract($this->config,EXTR_PREFIX_SAME,'config');
//pdo连接必须的dsn;
$dsn = sprintf('%s:host=%s;dbname=%s;',$type,$host,$dbName);
try
{
$this->dbConn = new PDO($dsn,$userName,$passWord);
}
catch(Exception $e)
{
die($e->getMessage());
}
}
//查询返回查询结果集
public function select($table,$where)
{
$sql = "SELECT * FROM `$table` WHERE $where";
//pdo的预处理对象
$stmt = $this->dbConn->prepare($sql);
$stmt->execute();
$records = $stmt->fetchAll(PDO::FETCH_ASSOC);
return $records;
}
//插入记录,输出是否成功添加记录
public function insert($table,$insData)
{
//把传入的添加数据的数组转换为一个SQL添加字符串
$insertSet = $this->toSqlStr($insData);
//pdo的预处理对象
$sql = "INSERT `$table` SET $insertSet";
$stmt = $this->dbConn->prepare($sql);
$stmt->execute();
$rowCount = $stmt->rowCount();//返回受影响的记录数
if($rowCount >= 1):
echo $rowCount,'条记录添加成功','<br>';
else:
echo '添加记录失败,原因:', $stmt ->errorInfo(),'<br>';
endif;
}
//更新记录,输出更新几条记录
public function update($table,$data,$where)
{
//把传入的添加数据的数组转换为一个SQL添加字符串
$updateSet = $this->toSqlStr($data);
//pdo的预处理对象
$sql = "UPDATE `$table` SET $updateSet WHERE $where";
try
{
$stmt = $this->dbConn->prepare($sql);
$stmt->execute();
$rowCount = $stmt->rowCount();//返回受影响的记录数
if($rowCount === 0):
echo '没有记录被更新<br>';
else:
echo $rowCount,'条记录更新成功','<br>';
endif;}
catch(Exception $e)
{
echo '更新失败,原因:',$e->getMessage();
}
}
//删除记录,输出是否删除成功
public function delete($table,$where)
{
$sql = "DELETE FROM $table WHERE $where";
//pdo的预处理对象
$stmt = $this->dbConn->prepare($sql);
$stmt->execute();
$rowCount = $stmt->rowCount();//返回受影响的记录数
if($rowCount === 0):
echo '没有记录被删除<br>';
else:
echo $rowCount,'条记录删除成功','<br>';
endif;
}
public function toSqlStr($arr):string
{
//把数组的键提取到一个数组中
$keys = array_keys($arr);
//把数组的值提取到一个数组中
$value = array_values($arr);
$con = count($keys);
$sqlStr ='';
for ($i=0;$i<$con;$i++):
if($i===$con-1):
$sqlStr .= " `$keys[$i]`='$value[$i]'";
else:
$sqlStr .= " `$keys[$i]`='$value[$i]' ,";
endif;
endfor;
return $sqlStr;
}
}
}
?>
2.自动加载文件 autoLoad.php
<?php
try
{
spl_autoload_register(function($className){
//DIRECTORY_SEPARATOR返回当前系统的目录分隔符
//将空间中的分隔符替换成当前系统的目录分隔符
$path = str_replace('\\', DIRECTORY_SEPARATOR, $className);
//__DIR__返回当前文件所在路径
//生成要加载的类文件名称
$file = __DIR__ . DIRECTORY_SEPARATOR . $path . '.php';
// 3. 加载这个文件
require $file;
});
}
catch(Exception $e)
{
$e->getMessage();
}
?>
- 文件目录结构
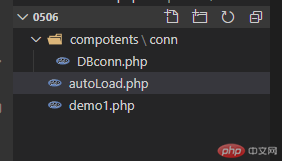
3.对数据库的CURD(增删改查)操作demo1.php
<?php
require 'autoLoad.php';
use compotents\conn\DBconn;
function printUserInfo($records)
{
foreach($records as $record):
echo '用户名:',$record['name'],'<br>';
echo '性别:',$record['sex'],'<br>';
echo '年龄:',$record['age'],'<br>';
echo '<hr>';
endforeach;
}
$user =new DBconn();
$table = 'tb_user';//表名
$where =''; //判断的条件
$data =[];//添加或者更新的数据
//显示所有用户信息
// $records = $user->select('SELECT * FROM `tb_user`');
// printUserInfo($records);
echo '****************显示所有男用户***************','<br>';
//查询操作
$where = '`sex`="男"';
$records = $user->select($table,$where);
printUserInfo($records);
//添加操作
$data = ['name'=>'wangjiao','password'=>'wj123','sex'=>'女','age'=>'14'];
$user->insert($table,$data);
// //更新操作
$where = '`id`>5';
$data = ['name'=>'hugn','password'=>'hugn456','sex'=>'男','age'=>'24'];
$user->update($table,$data,$where);
//添加操作
$where = '`id`>5';
$user->delete($table,$where);
?>
- 代码显示效果图
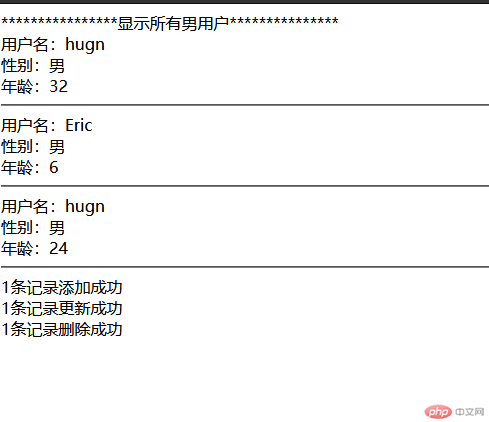