学习总结
- 一个类中可以引入多个trait,中间用逗号隔开
- 如果使用trait后,出现方法命名冲突,可以使用insteadof关键字进行替代,或者使用as关键字起个别名
- 可以在trait中实现接口的方法,然后在工作类中使用trait
- 幸运大转盘实例
1.类中引入多个trait后,方法命名冲突的解决办法
<?php
//一个类中可以引入多个trait
//trait中的方法如果重名
//1.可以使用insteadof关键字进行替代
//2.可以使用as关键字起一个别名,还可以修改方法的访问限制
trait tQhdx
{
public function printSDCInfo($school = '清华大学',$department = '自动化系',$classes = '05-01')
{
echo '班名:',$classes,'<br>';
echo '系名:',$department,'<br>';
echo '校名:',$school,'<br>';
echo '<hr>';
}
}
trait tRmdx
{
public function printSDCInfo($school = '人民大学',$department = '工商管理',$classes = '08-02')
{
echo '班名:',$classes,'<br>';
echo '系名:',$department,'<br>';
echo '校名:',$school,'<br>';
echo '<hr>';
}
}
trait tSchool
{
use tQhdx,tRmdx{
//如果访问printSDCInfo方法,则用清华大学替代人民大学
tQhdx::printSDCInfo insteadOf tRmdx;
//给清华大学中的printSDCInfo方法起别名为printQhdx;
tQhdx::printSDCInfo as protected printQhdx;
//给人民大学中的printSDCInfo方法起别名为printRmdx,并且修改方法的访问限制为受保护的;
tRmdx::printSDCInfo as protected printRmdx;
}
}
class StuInfo
{
use tSchool;
public function printStuInfo($name = 'angle',$age = 32,$sex = '女',$school = '清华大学')
{
echo '姓名:',$name,'<br>';
echo '年龄:',$age,'<br>';
echo '性别:',$sex,'<br>';
if ($school == '清华大学'):
$this ->printQhdx();
else:
$this ->printRmdx();
endif;
}
}
$stu1 = new StuInfo;
$stu1 ->printStuInfo();
$stu2 = new StuInfo;
$stu2 ->printStuInfo('hugn',31,'男','人民大学');
?>
- 运行效果图
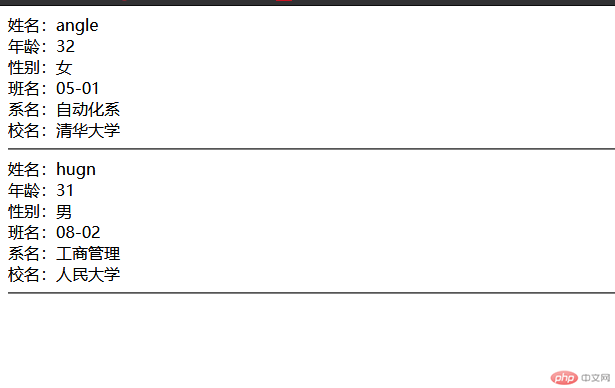
2.trait中可以实现接口中的方法,而不一定在工作类中实现
<?php
interface iSchool
{
public function printSDCInfo($school = '清华大学',$department = '自动化系',$classes = '05-01');
}
trait tStuInfo
{
//trait中实现接口的方法,可以使工作类中的代码更加简洁,易于维护
public function printSDCInfo($school = '清华大学',$department = '自动化系',$classes = '05-01')
{
echo '班名:',$classes,'<br>';
echo '系名:',$department,'<br>';
echo '校名:',$school,'<br>';
echo '<hr>';
}
public function printStuInfo($name = 'angle',$age = 32,$sex = '女',$school = '清华大学')
{
echo '姓名:',$name,'<br>';
echo '年龄:',$age,'<br>';
echo '性别:',$sex,'<br>';
if ($school == '清华大学'):
$this ->printSDCInfo();
else:
$this ->printSDCInfo('人民大学','工商管理系','08-02');
endif;
}
}
//接口中的方法可以在trait中实现
class StuInfo implements iSchool
{
use tStuInfo;
}
$stu1 = new StuInfo;
$stu1 ->printStuInfo();
$stu2 = new StuInfo;
$stu2 -> printStuInfo('hugn',31,'男','人民大学');
?>
- 运行效果图
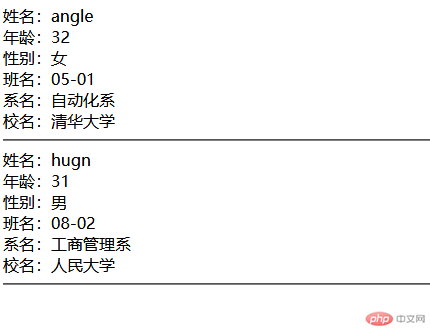
3.幸运大转盘实例
<?php
//当点击开始按钮时,每个奖品框的背景依次变为白色
//在0-60中取一个随机数做为抽奖的时间(奖品框背景依次变为白色的时间),时间到后,哪个奖品的背景为白色,就选中哪个奖品
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>幸运大转盘</title>
</head>
<style>
* {
padding: 0px;
margin: 0px;
box-sizing: border-box;
}
.container {
width: 500px;
height: 800px;
margin: auto;
display: flex;
flex-flow: column nowrap;
justify-content: center;
align-items: center;
}
.titleImage {
width: 300px;
height: 180px;
margin-bottom: 15px;
}
.items {
height: 500px;
display: grid;
grid-template-rows: repeat(3, 100px);
grid-template-columns: repeat(3, 150px);
grid-auto-flow: row;
gap: 5px;
justify-content: center;
align-items: center;
}
.items>div {
width: 100%;
height: 100%;
background-color: lightgreen;
}
.items>div:nth-of-type(5) {
width: auto;
height: auto;
background-color: white;
box-shadow: none;
}
.items>div {
display: flex;
justify-content: center;
align-items: center;
border-radius: 30%;
box-shadow: 2px 2px 2px #555;
}
span {
letter-spacing: 2px;
color: blueviolet;
text-shadow: 0.5px 0.5px 0.5px #555;
}
img {
width: 100%;
height: 100%;
}
</style>
<body>
<div class="container">
<div class="titleImage"><img src="luck.jpg" alt=""></div>
<div class="items">
<div><span>50银铃铛</span></div>
<div><span>10金铃铛</span></div>
<div><span>10元购书卷</span></div>
<div><span>谢谢参与</span></div>
<div><a href=""><img src="start.gif" alt=""></a></div>
<div><span>300银铃铛</span></div>
<div><span>再来一次</span></div>
<div><span>电子书一本</span></div>
<div><span>悦读卡1天</span></div>
</div>
</div>
</body>
</html>
- 运行效果图
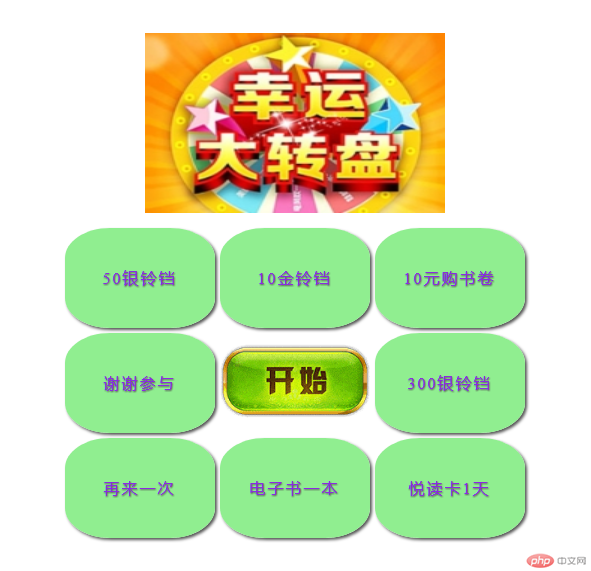