学习总结
- 如果需要加载重要的文件,则使用require进行文件加载,比如数据库连接的文件加载
- 其余文件使用include进行加载
- 如果想把重复的文件加载去除,使用require_once或者include_once
- 类是一个包含属性和方法的模板,属性就是变量,方法就是函数,把需要重复使用的变量和函数包裹在一个类模板中,如果想使用这个类中的变量和函数,就把类实例化为对象,然后就可以访问类中的属性和方法
- php示例代码
<?php
// 如果加载失败会出现致命错误,后面的代码不执行
require 'fileRequire.php';
require 'fileRequire.php';
require 'fileRequire.php';
require 'fileRequire.php';
require 'fileRequire.php';
// 只加载一次
require_once 'fileRequireOnce.php';
require_once 'fileRequireOnce.php';
require_once 'fileRequireOnce.php';
require_once 'fileRequireOnce.php';
require_once 'fileRequireOnce.php';
// 加载失败后面的代码一样会执行
include 'fileInclude.php';
include 'fileInclude.php';
include 'fileInclude.php';
include 'fileInclude.php';
include 'fileInclude.php';
// 只加载一次
include_once 'fileIncludeOnce.php';
include_once 'fileIncludeOnce.php';
include_once 'fileIncludeOnce.php';
include_once 'fileIncludeOnce.php';
include_once 'fileIncludeOnce.php';
class StudentInfo
{
//公共类属性 类中定义的变量必须加访问控制符,用public定义的为公共类属性
public $name = 'angle';
public $age = 32;
public $sex = '女';
public $class = '05-02班';
//静态类属性 用public static 定义的属性为静态属性,后期可能被更新
public static $school = '人民大学';
//抽象类属性 定义属性没有被赋值
public $chineseScore;
public $mathScore;
public $enghlishScore;
}
$stu1 = new StudentInfo();
// 直接使用公共属性
echo '姓名:',$stu1 ->name,'<br>';
echo '年龄:',$stu1 ->age,'<br>';
echo '性别:',$stu1 ->sex,'<br>';
echo '班级:',$stu1 ->class,'<br>';
echo '所在学校:',StudentInfo::$school,'<br>';
// 给抽象属性赋值
$stu1 ->chineseScore = 86;
$stu1 ->mathScore = 88;
$stu1 ->enghlishScore = 96;
echo $stu1 ->name,'语文成绩:',$stu1 ->chineseScore,'<br>';
echo $stu1 ->name,'数学成绩:',$stu1 ->mathScore,'<br>';
echo $stu1 ->name,'英语成绩:',$stu1 ->enghlishScore,'<br>';
echo '<hr>';
$stu2 = new StudentInfo();
// 也可以更新类中公共属性的值
$stu2 ->name = 'hugn';
$stu2 ->age = 31;
$stu2 ->sex = '男';
$stu2 ->class = '05-01班';
//更新静态属性
StudentInfo::$school = '清华大学';
$stu2 ->chineseScore = 96;
$stu2 ->mathScore = 98;
$stu2 ->enghlishScore = 94;
echo '姓名:',$stu2 ->name,'<br>';
echo '年龄:',$stu2 ->age,'<br>';
echo '性别:',$stu2 ->sex,'<br>';
echo '班级:',$stu2 ->class,'<br>';
echo '所在学校:',StudentInfo::$school,'<br>';
$stu2 ->chineseScore = 86;
$stu2 ->mathScore = 88;
$stu2 ->enghlishScore = 96;
echo $stu2 ->name,'语文成绩:',$stu2 ->chineseScore,'<br>';
echo $stu2 ->name,'数学成绩:',$stu2 ->mathScore,'<br>';
echo $stu2 ->name,'英语成绩:',$stu2 ->enghlishScore,'<br>';
?>
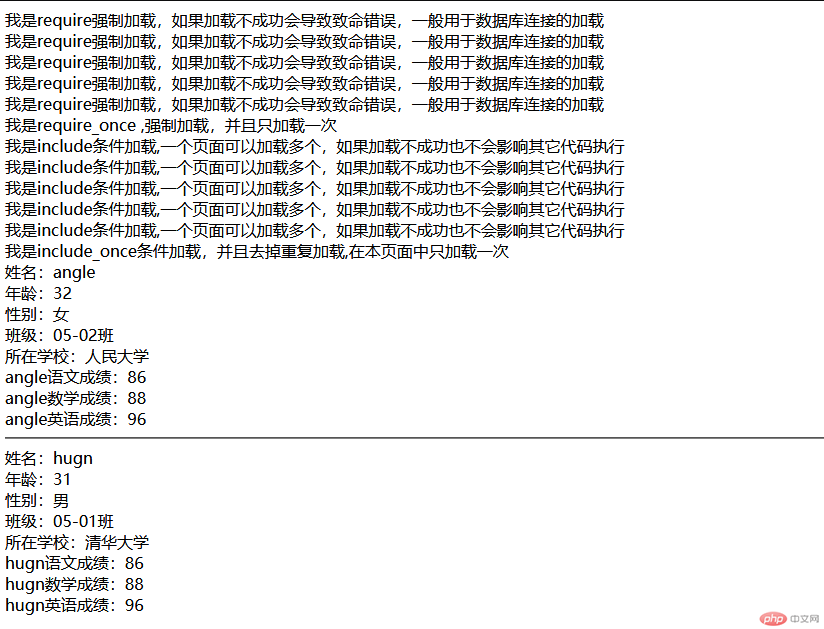