基础部分
<?php
class demo{
public $name;
protected $age;
private $country = '中国';
public function get(){
return $this->name . $this->age . $this->country;
}
public function __construct($a,$b)
{
$this->name = $a;
$this->age = $b;
}
}
$obj = new demo('张明轩',3);
echo $obj->name;
echo $obj->get();
trait公共区,子类继承
<?php
// trait 公共区
trait text{
public function get(){
return $this->name . $this->age . $this->country;
}
}
class demo{
public $name;
protected $age;
private $country = '中国';
// use 调用公共区
use text;
public function __construct($a,$b)
{
$this->name = $a;
$this->age = $b;
}
}
class sun extends demo{
public function __construct($a,$b,$c)
{
//子类 继承父类
parent::__construct($a,$b);
$this->c = $c;
}
public function get()
{
return parent::get() . $this->c . ' 这是子类调用';
}
}
$str = new sun('张明轩',3,'love');
echo $str->get();
interface接口
<?php
// 接口是一种约定, 定义了实现它的类中必须实现的方法
// 接口中没有方法的具体实现, 所以不能实例化
interface iDemo{
public function get();
public function hello();
}
class demo implements iDemo{
public $name;
protected $age;
private $country = '中国';
public function get(){
return $this->name . $this->age . $this->country;
}
public function hello(){
echo '接口文件,在类中必须一一呈现';
}
public function __construct($a,$b)
{
$this->name = $a;
$this->age = $b;
}
}
class sun extends demo{
public function __construct($a,$b,$c)
{
//子类 继承父类
parent::__construct($a,$b);
$this->c = $c;
}
//这里info()可以和父类get(),名字一致
public function info()
{
return parent::get() . $this->c . ' 这是子类调用';
}
}
$cc = new demo('张明轩',3,);
$cc->hello();
$str = new sun('张明轩',3,'love');
echo $str->info();
abstract抽象
<?php
// 抽象类:有抽象方法, 也是已实现的方法
// 接口全是抽象方法
// 共同之处: 统统不能实例化, 原因就是内部有抽象方法
abstract class iDemo{
public function hello(){
echo '抽象类:已经实现的方法';
}
// 抽象类中必须至少有一个抽象方法
abstract public function get();
}
class demo extends iDemo{
public $name;
protected $age;
private $country = '中国';
public function get(){
return $this->name . $this->age . $this->country;
}
public function __construct($a,$b)
{
$this->name = $a;
$this->age = $b;
}
}
class sun extends demo{
public function __construct($a,$b,$c)
{
//子类 继承父类
parent::__construct($a,$b);
$this->c = $c;
}
//这里info()可以和父类get(),名字一致
public function info()
{
return parent::get() . $this->c . ' 这是子类调用';
}
}
$arr = new demo('张明朗',1);
$arr->hello();
$str = new sun('张明轩',3,'love');
echo $str->info();
<?php
class main{
function __set($_name,$_val){
$this->$_name=$_val;//当外面直接设置私有成员属性$name的时候被自动调用
}
function __get($_name){
return $this->$_name;//当外面直接使用私有成员属性$name的时候被自动调用
}
}
$my = new main();
$my->name="李四";//此时,就调用了__set()魔术方法
echo $my->name;//此时,就调用__get()魔术方法
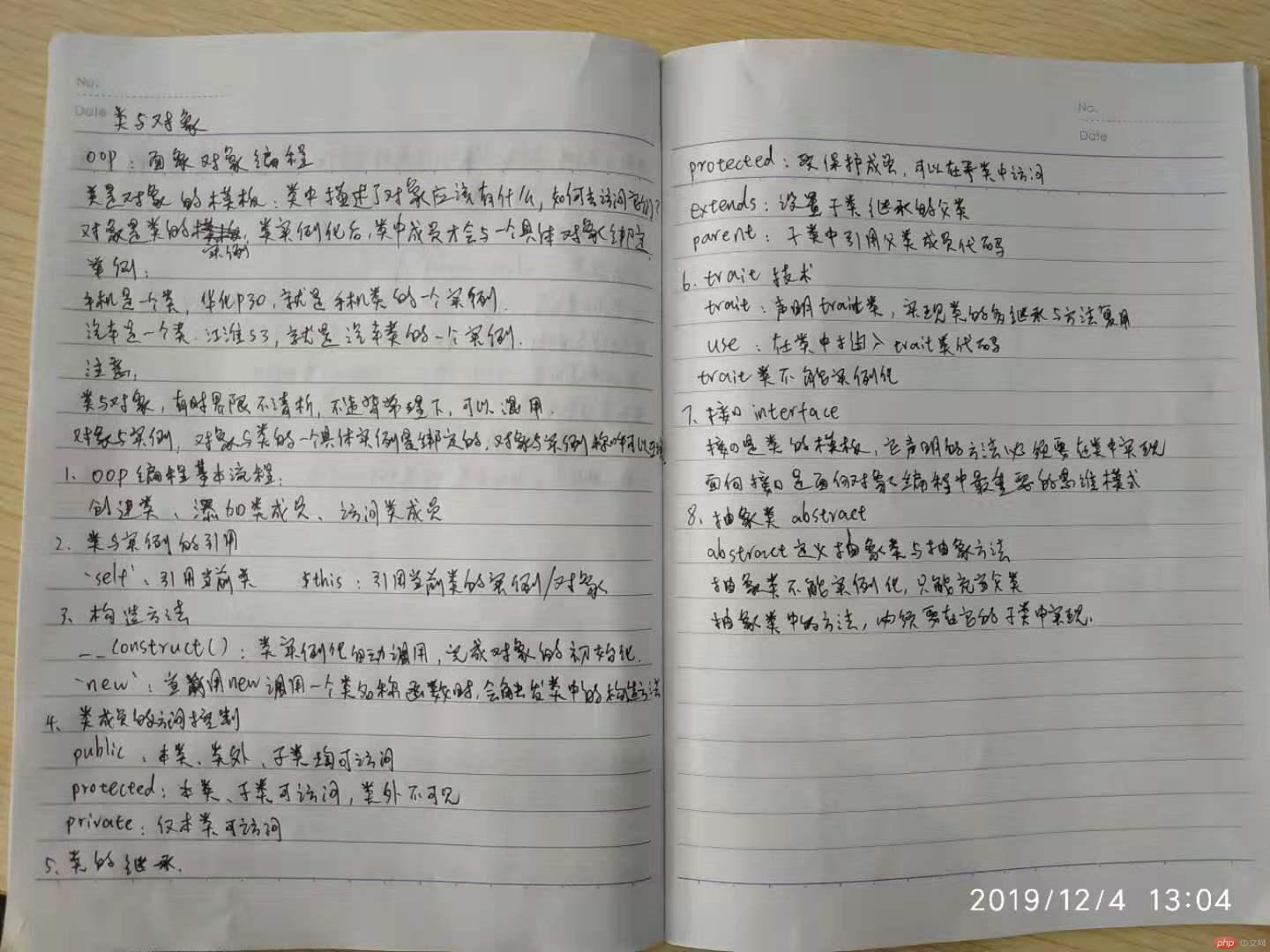