Let's talk about the usage skills of JavaScript conditional judgment
This article brings you relevant knowledge about JavaScript. It mainly talks about the usage skills of JavaScript conditional judgment. Friends who are interested can take a look at it together. I hope it will be helpful to everyone. help.
This article will introduce how to write simpler conditional judgments in JavaScript to help you write more concise code. Source code address
Suppose we have a function that converts color values into hexadecimal encoding.
function convertToHex(color) { if (typeof color === 'string') { if (color === 'slate') { return '#64748b' } else if (color === 'gray') { return '#6b7280' } else if (color === 'red') { return '#ef4444' } else if (color === 'orange') { return '#f97316' } else if (color === 'yellow') { return '#eab308' } else if (color === 'green') { return '#22c55e' } else { return '#ffffff' } } else { return '#ffffff' } }
The function of this function is very simple, that is, to pass in the color string and then return the corresponding hexadecimal number. If the passed in is not a string, or nothing is passed, then a white ten is returned. Hexadecimal.
Next we will start optimizing this code.
Avoid using strings directly as conditions
There is a problem with using strings directly as conditions, that is, when we make a spelling mistake, so awkward.
convertToHex("salte")
To avoid this error, we can use constants.
const Colors = { SLATE: 'slate', GRAY: 'gray', // ... } function convertToHex(color) { if (typeof color === 'string') { if (color === Colors.SLATE) { return '#64748b' } else if (color === Color.GRAY) { return '#6b7280' } // ... } else { return '#ffffff' } } convertToHex(Colors.SLATE)
If you are using typescript, you can use enumerations directly.
Using Object
In fact, it is not difficult to find from the above code that we can directly store the hexadecimal value into the object in value.
const Colors = { SLATE: '#64748b', GRAY: '#6b7280', // ... } function convertToHex(color) { if (color in Colors) { return Colors[color] } else { return '#ffffff' } } convertToHex(Colors.SLATE)
This way the code will be more concise and easier to read.
Does not meet expectations, return in advance
Another best practice is that we can write the situation that does not meet expectations to the front of the function and return in advance to avoid forgetting to return. const Colors = { SLATE: '#64748b', GRAY: '#6b7280', // ... } function convertToHex(color) { if (!color in Colors) { return '#ffffff' } return Colors[color] } convertToHex(Colors.SLATE)
const Colors = { SLATE: '#64748b', GRAY: '#6b7280', // ... } function convertToHex(color) { if (!color in Colors) { return '#ffffff' } return Colors[color] } convertToHex(Colors.SLATE)
This way there is no need for else. Using this technique, we can eliminate a lot of else in our code.
Use Map with Object
Using map is more professional, because map can store any type of key, and it inherits from Map.prototype, Has more convenient methods and properties.
And Object is more convenient to access properties. We can continue to use Object to implement enumeration.
const ColorsEnum = { SLATE: 'slate', GRAY: 'gray', // ... } const Colors = new Map() Colors.set(ColorsEnum.SLATE, '#64748b') Colors.set(ColorsEnum.GRAY, '#6b7280') // ... Colors.set('DEFAULT', '#ffffff') function convertToHex(color) { if (!Colors.has(color)) { return Colors.get('DEFAULT') } return Colors.get(color) } convertToHex(Colors.SLATE)
Map can also store functions
Suppose we store a lot of colors, up to thousands, and we also need to support back-end configuration, and the results can be obtained through some kind of calculation process.
Then we can use Map to store functions.
return Colors.get(color)()
Try to avoid ternary expressions and switch
Although ternary expressions are brief, their readability is greatly reduced. If it is a multi-level condition, it will be very difficult to read.
switch has no obvious advantage over if. Instead, it is sometimes easy to return, causing the code to not execute as expected.
The above is the detailed explanation of JavaScript conditional judgment usage techniques.
Recommended study: "JavaScript Video Tutorial"
The above is the detailed content of Let's talk about the usage skills of JavaScript conditional judgment. For more information, please follow other related articles on the PHP Chinese website!
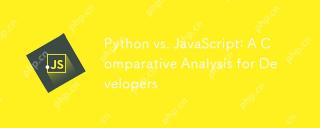
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
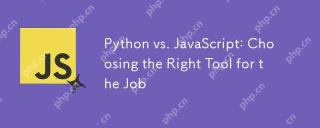
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
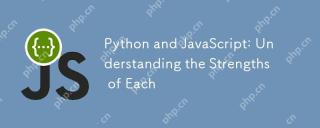
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
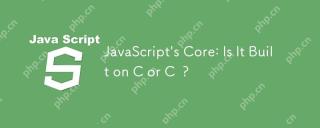
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
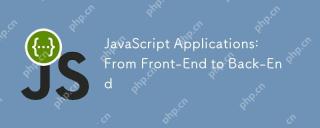
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
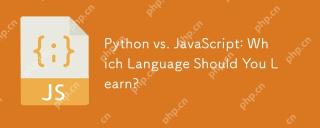
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
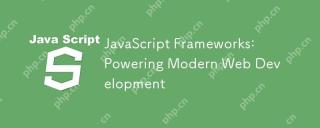
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
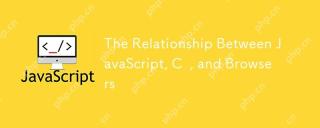
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
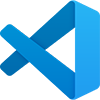
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Notepad++7.3.1
Easy-to-use and free code editor
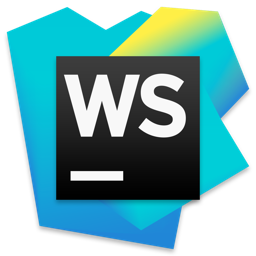
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 Chinese version
Chinese version, very easy to use

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
